- Java Programming Basics
- Java Tutorial
- Java Overview
- Java Environment Setup
- Java Program Structure
- Java Basic Syntax
- Java First Program
- Java Constants
- Java Separators
- Java Keywords
- Java Data Types
- Java Data Types
- Java Integers
- Java Floating Point
- Java Characters
- Java Booleans
- Java Numbers
- Java Programming Variables
- Java Variables
- Java Variable Types
- Java Variable Scope
- Java Type Conversion
- Java Type Casting
- Java Auto Type Promotion
- Java Type Promotion Rules
- Java Programming Arrays
- Java Arrays
- Java One Dimensional Array
- Java Multidimensional Array
- Java Programming Operators
- Java Operators
- Java Arithmetic Operators
- Java Increment Decrement
- Java Bitwise Operators
- Java Left Shift
- Java Right Shift
- Java Relational Operators
- Java Boolean Logical Operators
- Java Ternary(?) Operator
- Java Operator Precedence
- Java Control Statements
- Java Decision Making
- Java if if-else if-else-if
- Java switch Statement
- Java Loops
- Java while Loop
- Java do-while Loop
- Java for Loop
- Java for-each Loop
- Java Nested Loops
- Java break Statement
- Java continue Statement
- Java Class Object Method
- Java Classes and Objects
- Java Class
- Java Object
- Java new Operator
- Java Methods
- Java Constructors
- Java this Keyword
- Java Stack
- Java Overloading Recursion
- Java Method Overloading
- Java Constructor Overloading
- Java Object as Parameter
- Java Call by Value Reference
- Java Returning Objects
- Java Recursion
- Java Modifier Types
- Java Encapsulate Poly String
- Java Encapsulation
- Java Polymorphism
- Java Nested Inner Class
- Java Strings
- Java Command Line Arguments
- Java Variable Length Arguments
- Java Inheritance Abstraction
- Java Inheritance
- Java super Superclass
- Java Multilevel Hierarchy
- Java Method Overriding
- Java Abstraction
- Java Packages Interfaces
- Java Packages
- Java Access Protection
- Java Import Statement
- Java Interfaces
- Java Programming Exceptions
- Java Exception Handling
- Java try catch
- Java throw throws
- Java finally Block
- Java Built In Exceptions
- Java Exception Subclasses
- Java Chained Exceptions
- Java Multithreading
- Java Multithreading
- Java Thread Model
- Java Main Thread
- Java Create Thread
- Java Thread Priorities
- Java Synchronization
- Java Inter Thread Communication
- Java Suspend Resume Stop Thread
- Java Get Thread State
- Java Enum Autobox Annotation
- Java Enumerations
- Java Type Wrappers
- Java Autoboxing
- Java Annotation
- Java Marker Annotations
- Java Single Member Annotation
- Java Built In Annotations
- Java Type Annotations
- Java Repeating Annotations
- Java Data File Handling
- Java Files I/O
- Java Streams
- Java Read Console Input
- Java Write Console Output
- Java PrintWriter Class
- Java Read Write Files
- Java Automatically Close File
- Java Programming Advance
- Java Date and Time
- Java Regular Expressions
- Java Collections Framework
- Java Generics
- Java Data Structures
- Java Network Programming
- Java Serialization
- Java Send Email
- Java Applet Basics
- Java Documentation
- Java Programming Examples
- Java Programming Examples
Java Variable Scope
So far, all of the variables used have been declared at the start of main() method. However, Java allows the variables to be declared within any block.
A block is begin with an opening curly brace and ended by a closing curly brace. A block defines a scope. Thus, each time you start a new block, you are creating a new scope. A scope determines what objects are visible to the other parts of your program. It also determines the lifetime of those objects.
Many other computer languages defines two general categories of scopes: global and local. However, these traditional scopes do not fit well with Java's strict, object-oriented model. While it is possible to create what amounts to being a global scope, it is far by the exception, not the rule.
In Java, two major scopes are those defined by a class and those defined by a method. Even this distinction is somewhat artificial. However, since the class scope has several unique properties and attributes that do not apply to the scope defined by a method, this distinction makes some sense. You will learn later about class in separate chapter. For now, we will only examine the scopes defined by/within a method.
The scope defined by a method begins with its opening curly brace. However, if that method has parameters, they too are included within the method's scope.
Java Scope Rules
As a general rule, variables declared inside a scope are not visible/accessible to the code that is defined outside the scope. Thus, when you declare a variable within a scope, you are localizing that variable and protecting it from an unauthorized access and/or modification. Indeed, the scope rules provide the foundation for encapsulation.
Scopes can be nested. For example, each time you create a block of code, you are creating a new, nested scope. When this occurs, the outer scope encloses the inner scope. This means that the objects declared in the outer scope will be visible to code within the inner scope. However, the reverse is not true. Objects declared within the inner scope will not be visible outside it.
Java Variable Scope Example
To understand the effect of nested scopes, consider the following program:
/* Java Program Example - Java Variables Scope */ public class JavaProgram { public static void main(String args[]) { int x; //known to all code within main x = 10; if(x == 10) { int y = 20; //known only to this block /* x and y both known here */ System.out.println("x : " + x + "\ny : " + y); x = y * 2; } // y = 100; //error! y not known here /* x is still known here */ System.out.println("x is " +x); } }
When the above Java program is compile and run, it will produce the following output:
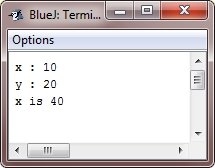
As the comments indicate, the variable x is declared at the start of the main()'s scope and is accessible to all subsequent code within the main(). Within the if block, y is declared. Since a block defines a scope, y is only visible to other code within its block. This is why outside of its block, the line y = 100; is commented out. If you remove the leading comment symbol i.e., //, a compile-time error will occur, because y is not visible outside of its block. Within the if block, x can be used because code within a block (i.e., a nested scope) has access to the variables declared by an enclosing scope.
Within a block, variables can be declared at any point, but are valid only after they are declared. Thus, if you define a variable at the start of a method, it is available to all of the code within that method. Conversely, if you declare a variable at the end of a block, it is effectively useless, because no code will have access to it. For example, the following code fragment is invalid because count cannot be used prior to its declaration :
// This fragment is wrong! count = 100; // oops! cannot use count before it is declared! int count;
Here is another important point to remember i.e., the variables are created when their scope is entered, and destroyed when their scope is left. This means that a variable will not hold its value once it has gone out of the scope. Therefore, the variables declared within a method will not hold their values between calls to that method. Also, a variable declared within a block will lose its value when the block is left. Thus, the lifetime of a variable is confined to its scope.
If a variable declaration includes an initializer, then that variable will be reinitialized each time the block in which it is declared is entered. For example, consider the following program :
/* Java Program Example - Demonstrate lifetime of a variable - Java Scope Rules */ public class JavaProgram { public static void main(String args[]) { int x; for(x=0; x<5; x++) { int y = -1; //y is initialized each time block is entered System.out.println("y is : " +y); //this always prints -1 y = 100; System.out.println("y is now : " +y); } } }
When the above Java program is compile and run, it will produce the following output:
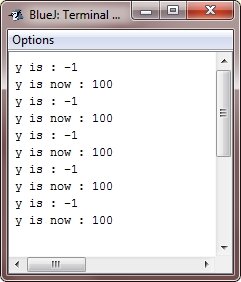
As you can see, y is reinitialized to -1 each time the inner for loop is entered. Even though it is subsequently assigned the value 100, this value is lost.
« Previous Tutorial Next Tutorial »