- Java Programming Basics
- Java Tutorial
- Java Overview
- Java Environment Setup
- Java Program Structure
- Java Basic Syntax
- Java First Program
- Java Constants
- Java Separators
- Java Keywords
- Java Data Types
- Java Data Types
- Java Integers
- Java Floating Point
- Java Characters
- Java Booleans
- Java Numbers
- Java Programming Variables
- Java Variables
- Java Variable Types
- Java Variable Scope
- Java Type Conversion
- Java Type Casting
- Java Auto Type Promotion
- Java Type Promotion Rules
- Java Programming Arrays
- Java Arrays
- Java One Dimensional Array
- Java Multidimensional Array
- Java Programming Operators
- Java Operators
- Java Arithmetic Operators
- Java Increment Decrement
- Java Bitwise Operators
- Java Left Shift
- Java Right Shift
- Java Relational Operators
- Java Boolean Logical Operators
- Java Ternary(?) Operator
- Java Operator Precedence
- Java Control Statements
- Java Decision Making
- Java if if-else if-else-if
- Java switch Statement
- Java Loops
- Java while Loop
- Java do-while Loop
- Java for Loop
- Java for-each Loop
- Java Nested Loops
- Java break Statement
- Java continue Statement
- Java Class Object Method
- Java Classes and Objects
- Java Class
- Java Object
- Java new Operator
- Java Methods
- Java Constructors
- Java this Keyword
- Java Stack
- Java Overloading Recursion
- Java Method Overloading
- Java Constructor Overloading
- Java Object as Parameter
- Java Call by Value Reference
- Java Returning Objects
- Java Recursion
- Java Modifier Types
- Java Encapsulate Poly String
- Java Encapsulation
- Java Polymorphism
- Java Nested Inner Class
- Java Strings
- Java Command Line Arguments
- Java Variable Length Arguments
- Java Inheritance Abstraction
- Java Inheritance
- Java super Superclass
- Java Multilevel Hierarchy
- Java Method Overriding
- Java Abstraction
- Java Packages Interfaces
- Java Packages
- Java Access Protection
- Java Import Statement
- Java Interfaces
- Java Programming Exceptions
- Java Exception Handling
- Java try catch
- Java throw throws
- Java finally Block
- Java Built In Exceptions
- Java Exception Subclasses
- Java Chained Exceptions
- Java Multithreading
- Java Multithreading
- Java Thread Model
- Java Main Thread
- Java Create Thread
- Java Thread Priorities
- Java Synchronization
- Java Inter Thread Communication
- Java Suspend Resume Stop Thread
- Java Get Thread State
- Java Enum Autobox Annotation
- Java Enumerations
- Java Type Wrappers
- Java Autoboxing
- Java Annotation
- Java Marker Annotations
- Java Single Member Annotation
- Java Built In Annotations
- Java Type Annotations
- Java Repeating Annotations
- Java Data File Handling
- Java Files I/O
- Java Streams
- Java Read Console Input
- Java Write Console Output
- Java PrintWriter Class
- Java Read Write Files
- Java Automatically Close File
- Java Programming Advance
- Java Date and Time
- Java Regular Expressions
- Java Collections Framework
- Java Generics
- Java Data Structures
- Java Network Programming
- Java Serialization
- Java Send Email
- Java Applet Basics
- Java Documentation
- Java Programming Examples
- Java Programming Examples
Java do-while Loop
The do-while loop is mainly used in the menu-driven programs. As you just saw in the previous chapter, if the conditional expression controlling the while loop is initially false, then the body of the loop will not be executed at all. However, sometimes it is desirable to execute the body of the loop at once, even if the conditional expression is false to start with. In other words, there are times when you would like to test the termination expression at the end of the loop rather than at the beginning to run the body of the loop at once if the conditions becomes false at first. Fortunately, Java supplies a loop to overcome this problem, i.e., the do-while loop.
The do-while loop always executes its body at least once, as its conditional expression is at the bottom of the loop.
Java do-while Loop Syntax
Following is the general form of the do-while loop :
do { // body of the loop }while(conditional-expression);
Each iteration (looping) of the do-while loop first executes the body of the loop and then evaluates the conditional expression. If the expression becomes true, the loop will repeat. Otherwise, the loop will terminates. As with all of the Java's loop, condition must be a Boolean expression.
Java do-while Loop Example
Following is a reworked version of the "tick" program (as we saw earlier in the while loop chapter) which demonstrates the do-while loop here. It generates the same output as before :
/* Java Program Example - Java do-while Loop * This program demonstrates the do-while loop */ public class JavaProgram { public static void main(String args[]) { int n = 10; do { System.out.println("tick " + n); n--; }while(n > 0); } }
When the above Java program is compile and executed, it will produce the following output:
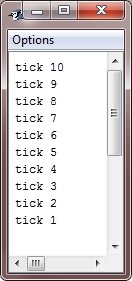
The loop in the above program, while technically correct, can be written more efficiently as follows :
do { System.out.println("tick " + n); }while(--n > 0);
In this example, the (--n > 0) expression combines the decrement of n and the test for zero into one expression.
Here is how it works.
First, the statement (--n) executes, decrementing the n and returning the new value of n. This value is then compared with
zero
. If it will greater than zero, then the loop continues, otherwise, terminates.
The do-while loop is especially useful when you process a menu selection program, as you will usually want the body of a menu loop to execute at least once. Consider the program given here, which implements a very simple help system for the Java's selection and iteration statements :
Java do-while Loop Example - Menu Selection
Here is a simple menu-driven program using do-while loop in Java:
/* Java Program Example - Java do-while Loop * Using a do-while loop to process a menu selection */ import java.util.Scanner; public class JavaProgram { public static void main(String args[]) { Scanner scan = new Scanner(System.in); char choice; do { System.out.println("Help on : "); System.out.println("1. if"); System.out.println("2. switch"); System.out.println("3. while"); System.out.println("4. do-while"); System.out.println("5. for\n"); System.out.println("Choose any one : "); choice = scan.next().charAt(0); }while(choice < '1' || choice > '5'); System.out.println("\n"); switch(choice) { case '1' : System.out.println("The if :\n"); System.out.println("if(condition)\n{\n\tstatement\n}"); System.out.println("else\n{\n\tstatement\n}"); break; case '2' : System.out.println("The switch :\n"); System.out.println("switch(expression)\n{"); System.out.println("\tcase constant: statement sequence\n\tbreak;"); System.out.println("\t//...\n}"); break; case '3' : System.out.println("The while :\n"); System.out.println("while(condition)\n{"); System.out.println("\t// body of loop\n}"); break; case '4' : System.out.println("The do-while :\n"); System.out.println("do\n{"); System.out.println("\t// body of loop\n\n}while(condition);"); break; case '5' : System.out.println("The for :\n"); System.out.println("for(initialization; condition; iteration)\n{"); System.out.println("\t// body of loop\n}"); break; } } }
When the above Java program is compile and executed, it will produce the following output. Output when none selected (fresh menu):
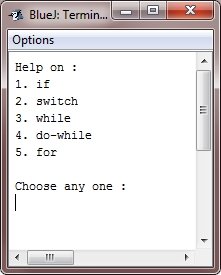
Here is the output produced when 1 is selected
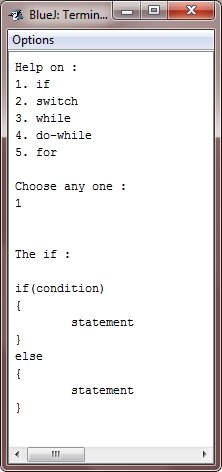
Output produced when 2 is selected:
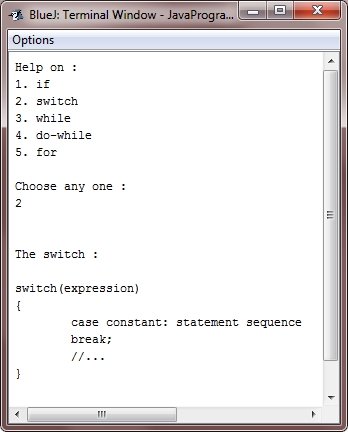
Output produced when 3 is selected:
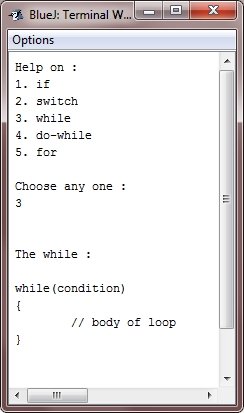
Output produced when 4 is selected:
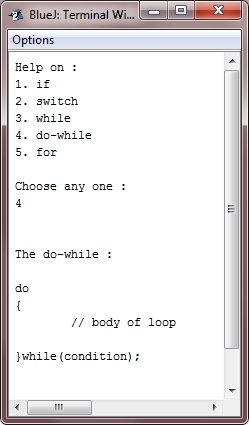
Output produced when 5 is selected:
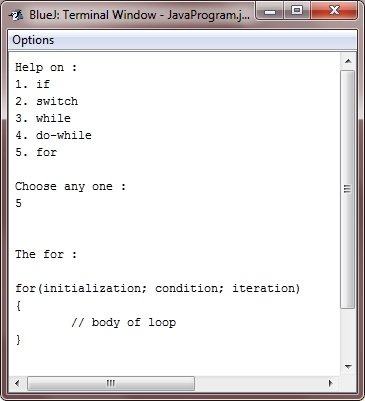
In the above Java program, the do-while loop is used to verify that the user has entered a valid choice. If not, then the user is re-prompted. As the menu must be displayed at least once, the do-while is the perfect loop to accomplish this.
« Previous Tutorial Next Tutorial »