- Java Programming Basics
- Java Tutorial
- Java Overview
- Java Environment Setup
- Java Program Structure
- Java Basic Syntax
- Java First Program
- Java Constants
- Java Separators
- Java Keywords
- Java Data Types
- Java Data Types
- Java Integers
- Java Floating Point
- Java Characters
- Java Booleans
- Java Numbers
- Java Programming Variables
- Java Variables
- Java Variable Types
- Java Variable Scope
- Java Type Conversion
- Java Type Casting
- Java Auto Type Promotion
- Java Type Promotion Rules
- Java Programming Arrays
- Java Arrays
- Java One Dimensional Array
- Java Multidimensional Array
- Java Programming Operators
- Java Operators
- Java Arithmetic Operators
- Java Increment Decrement
- Java Bitwise Operators
- Java Left Shift
- Java Right Shift
- Java Relational Operators
- Java Boolean Logical Operators
- Java Ternary(?) Operator
- Java Operator Precedence
- Java Control Statements
- Java Decision Making
- Java if if-else if-else-if
- Java switch Statement
- Java Loops
- Java while Loop
- Java do-while Loop
- Java for Loop
- Java for-each Loop
- Java Nested Loops
- Java break Statement
- Java continue Statement
- Java Class Object Method
- Java Classes and Objects
- Java Class
- Java Object
- Java new Operator
- Java Methods
- Java Constructors
- Java this Keyword
- Java Stack
- Java Overloading Recursion
- Java Method Overloading
- Java Constructor Overloading
- Java Object as Parameter
- Java Call by Value Reference
- Java Returning Objects
- Java Recursion
- Java Modifier Types
- Java Encapsulate Poly String
- Java Encapsulation
- Java Polymorphism
- Java Nested Inner Class
- Java Strings
- Java Command Line Arguments
- Java Variable Length Arguments
- Java Inheritance Abstraction
- Java Inheritance
- Java super Superclass
- Java Multilevel Hierarchy
- Java Method Overriding
- Java Abstraction
- Java Packages Interfaces
- Java Packages
- Java Access Protection
- Java Import Statement
- Java Interfaces
- Java Programming Exceptions
- Java Exception Handling
- Java try catch
- Java throw throws
- Java finally Block
- Java Built In Exceptions
- Java Exception Subclasses
- Java Chained Exceptions
- Java Multithreading
- Java Multithreading
- Java Thread Model
- Java Main Thread
- Java Create Thread
- Java Thread Priorities
- Java Synchronization
- Java Inter Thread Communication
- Java Suspend Resume Stop Thread
- Java Get Thread State
- Java Enum Autobox Annotation
- Java Enumerations
- Java Type Wrappers
- Java Autoboxing
- Java Annotation
- Java Marker Annotations
- Java Single Member Annotation
- Java Built In Annotations
- Java Type Annotations
- Java Repeating Annotations
- Java Data File Handling
- Java Files I/O
- Java Streams
- Java Read Console Input
- Java Write Console Output
- Java PrintWriter Class
- Java Read Write Files
- Java Automatically Close File
- Java Programming Advance
- Java Date and Time
- Java Regular Expressions
- Java Collections Framework
- Java Generics
- Java Data Structures
- Java Network Programming
- Java Serialization
- Java Send Email
- Java Applet Basics
- Java Documentation
- Java Programming Examples
- Java Programming Examples
Java Suspend Resume Stop Threads
Sometimes, suspending the execution of a thread is useful. For example, a separate thread can be used to display the time of day. If user does not want a clock, then its thread can be suspended. Whatever the case, suspending a thread is a simple matter. Once suspended, restarting the thread is also a simple matter.
The mechanisms to suspend, resume, and stop threads differ between early versions of Java, such as Java 1.0, and modern versions, beginning with Java 2. Prior to Java 2, a program used the suspend(), resume(), and stop() methods, which are defined by the Thread, to pause, restart, and stop the execution of a thread. Although these methods seem to be a perfectly reasonable and convenient approach to managing the execution of threads, they must not be used for new Java programs.
Java suspend Thread
The suspend() method of Thread class was deprecated by Java 2 several years ago. This was done because the suspend() can sometimes cause serious system failures.
Assume that a thread has obtained locks on the critical data structures. If that thread is suspended at that point, those locks are not relinquished. Other threads that may be waiting for those resources can be deadlocked.
Java resume Thread
The resume() method is also deprecated. It doest not cause problems, but cannot be used without the suspend() method as its counterpart.
Java stop Thread
The stop() method of Thread class, too, was deprecated by Java 2. This was done because this method can sometimes cause serious system failures.
Assume that a thread is writing to a critically important data structure and has completed only part of its changes. If that thread is stopped at that point, that data structure might be left in a corrupted state. The trouble is that, the stop() causes any lock the calling thread holds to be released. Thus, the corrupted data might be used by another thread that is waiting on the same lock.
Java suspend resume stop Thread Example
Because you can not now use the suspend(), resume(), or stop() methods to control a thread, you might be thinking that no way exists to pause, restart, or terminate a thread. But, fortunately, this is not true. Instead, a thread must be designed so that the run() method periodically checks to determine whether that thread should suspend, resume, or stop its own execution. Typically, this is accomplished by establishing a flag variable that indicates the execution state of the thread. As long as this flag is set to "running", run() method must continue to let the thread execute. If this variable is set to "suspend", the thread must pause. If it is set to "stop", the thread must terminate. Of course, a variety of ways exist in which to write such code, but the central theme will be same for all the programs.
The following example illustrates how wait() and notify() methods that are inherited from the Object can be used to control the execution of a thread. Let's consider its operation. The NewThread class contains a boolean instance variable named suspendFlag which is used to control the execution of the thread. It is initialized to false by the constructor. The run() method contains a synchronized statement block that checks suspendFlag. If that variable is true, the wait() method is invoked to suspend the execution of the thread. The mysuspend() method sets suspendFlag to true. The myresume() method sets suspendFlag to false and invokes notify() to wake up the thread. Finally, the main() method has been modified to invoke the mysuspend() and myresume() methods.
/* Java Program Example - Java Suspend Resume Stop Thread * Suspending and resuming a thread the modern way */ class NewThread implements Runnable { String name; //name of thread Thread thr; boolean suspendFlag; NewThread(String threadname) { name = threadname; thr = new Thread(this, name); System.out.println("New thread : " + thr); suspendFlag = false; thr.start(); // start the thread } /* this is the entry point for thread */ public void run() { try { for(int i=12; i>0; i--) { System.out.println(name + " : " + i); Thread.sleep(200); synchronized(this) { while(suspendFlag) { wait(); } } } } catch(InterruptedException e) { System.out.println(name + " interrupted"); } System.out.println(name + " exiting..."); } synchronized void mysuspend() { suspendFlag = true; } synchronized void myresume() { suspendFlag = false; notify(); } } class SuspendResumeThread { public static void main(String args[]) { NewThread obj1 = new NewThread("One"); NewThread obj2 = new NewThread("two"); try { Thread.sleep(1000); obj1.mysuspend(); System.out.println("Suspending thread One..."); Thread.sleep(1000); obj1.myresume(); System.out.println("Resuming thread One..."); obj2.mysuspend(); System.out.println("Suspending thread Two..."); Thread.sleep(1000); obj2.myresume(); System.out.println("Resuming thread Two..."); } catch(InterruptedException e) { System.out.println("Main thread Interrupted..!!"); } /* wait for threads to finish */ try { System.out.println("Waiting for threads to finish..."); obj1.thr.join(); obj2.thr.join(); } catch(InterruptedException e) { System.out.println("Main thread Interrupted..!!"); } System.out.println("Main thread exiting..."); } }
When you compile and run the above Java program, you will see the threads suspend and resume as shown here :
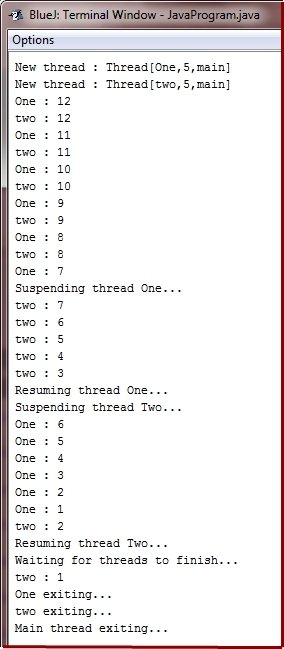
« Previous Tutorial Next Tutorial »