- Java Programming Basics
- Java Tutorial
- Java Overview
- Java Environment Setup
- Java Program Structure
- Java Basic Syntax
- Java First Program
- Java Constants
- Java Separators
- Java Keywords
- Java Data Types
- Java Data Types
- Java Integers
- Java Floating Point
- Java Characters
- Java Booleans
- Java Numbers
- Java Programming Variables
- Java Variables
- Java Variable Types
- Java Variable Scope
- Java Type Conversion
- Java Type Casting
- Java Auto Type Promotion
- Java Type Promotion Rules
- Java Programming Arrays
- Java Arrays
- Java One Dimensional Array
- Java Multidimensional Array
- Java Programming Operators
- Java Operators
- Java Arithmetic Operators
- Java Increment Decrement
- Java Bitwise Operators
- Java Left Shift
- Java Right Shift
- Java Relational Operators
- Java Boolean Logical Operators
- Java Ternary(?) Operator
- Java Operator Precedence
- Java Control Statements
- Java Decision Making
- Java if if-else if-else-if
- Java switch Statement
- Java Loops
- Java while Loop
- Java do-while Loop
- Java for Loop
- Java for-each Loop
- Java Nested Loops
- Java break Statement
- Java continue Statement
- Java Class Object Method
- Java Classes and Objects
- Java Class
- Java Object
- Java new Operator
- Java Methods
- Java Constructors
- Java this Keyword
- Java Stack
- Java Overloading Recursion
- Java Method Overloading
- Java Constructor Overloading
- Java Object as Parameter
- Java Call by Value Reference
- Java Returning Objects
- Java Recursion
- Java Modifier Types
- Java Encapsulate Poly String
- Java Encapsulation
- Java Polymorphism
- Java Nested Inner Class
- Java Strings
- Java Command Line Arguments
- Java Variable Length Arguments
- Java Inheritance Abstraction
- Java Inheritance
- Java super Superclass
- Java Multilevel Hierarchy
- Java Method Overriding
- Java Abstraction
- Java Packages Interfaces
- Java Packages
- Java Access Protection
- Java Import Statement
- Java Interfaces
- Java Programming Exceptions
- Java Exception Handling
- Java try catch
- Java throw throws
- Java finally Block
- Java Built In Exceptions
- Java Exception Subclasses
- Java Chained Exceptions
- Java Multithreading
- Java Multithreading
- Java Thread Model
- Java Main Thread
- Java Create Thread
- Java Thread Priorities
- Java Synchronization
- Java Inter Thread Communication
- Java Suspend Resume Stop Thread
- Java Get Thread State
- Java Enum Autobox Annotation
- Java Enumerations
- Java Type Wrappers
- Java Autoboxing
- Java Annotation
- Java Marker Annotations
- Java Single Member Annotation
- Java Built In Annotations
- Java Type Annotations
- Java Repeating Annotations
- Java Data File Handling
- Java Files I/O
- Java Streams
- Java Read Console Input
- Java Write Console Output
- Java PrintWriter Class
- Java Read Write Files
- Java Automatically Close File
- Java Programming Advance
- Java Date and Time
- Java Regular Expressions
- Java Collections Framework
- Java Generics
- Java Data Structures
- Java Network Programming
- Java Serialization
- Java Send Email
- Java Applet Basics
- Java Documentation
- Java Programming Examples
- Java Programming Examples
Java Read from Console Input
In Java 1.0, the only way to perform the console input was to use a byte stream. Today, using a byte stream to read console input is still acceptable. However, for the commercial applications, the preferred method of reading console input is to use a character-oriented stream. This makes your program easier to internationalize and maintain.
In Java, console input is accomplished by reading from System.in. To obtain a character-based stream that is attached to the console, wrap System.in in a BufferedReader object.
BufferedReader supports a buffered input stream. A commonly used constructor is shown below :
BufferedReader(Reader inputReader)
Here, inputReader is the stream that is linked to the instance of BufferedReader that is being created. Reader is an abstract class. One of its concrete subclasses is InputStreamReader, which converts bytes to characters. To obtain an InputStreamReader object that is linked to System.in, use the following constructor:
InputStreamReader(InputStream inputStream)
Because System.in refers to an object of type InputStream, it can be used for inputStream. Putting it all together, the following line of code creates a BufferedReader that is connected to the keyboard:
BufferedReader br = new BufferedReader(new InputStreamReader(System.in));
After this statement executes, br is a character-based stream that is linked to console through System.in.
Read Characters in Java
Use read() method, to read a character from a BufferedReader.
The version of read() that we will be using is
int read() throws IOException
Each time that the read() is called, it reads a character from the input stream and returns it as an integer value. It returns -1 when the end of the stream is encountered. As you can see, it can throw an IOException.
The following program demonstrates the read() by reading the characters from the console until the user types a "q". Notice that any I/O exceptions that might be generated are simply thrown out of the main(). Such an approach is common when reading from console in simple example programs, but in more sophisticated applications, you can handle the exceptions explicitly.
/* Java Program Example - Read Console Input in Java * Use a BufferedReader to read characters from the console */ import java.io.*; class ReadConsoleInput { public static void main(String args[]) throws IOException { char chr; BufferedReader br = new BufferedReader(new InputStreamReader(System.in)); System.out.print("Enter a character (Press 'q' to quit) : "); // read characters now do { chr = (char) br.read(); System.out.println(chr); } while(chr != 'q'); } }
Below is a sample run of the above program :
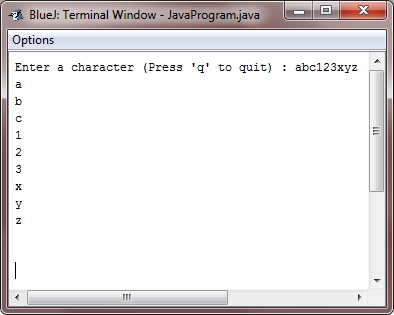
This output may look a little different from what you expected because System.in is line buffered, by default. This means that no input is actually passed to the program until you press ENTER. As you can guess, this doesn't make read() particularly valuable for the interactive console input.
Read Strings in Java
To read a string from the keyboard, use the version of the readLine() method that is a member of the BufferedReader class. Its general form is shown below:
String readLine() throws IOException
As you can see, it returns a String object.
The following program demonstrates the BufferedReader and the readLine() method, the program reads and displays the lines of text until you enter the word "stop" :
/* Java Program Example - Read Console Input in Java * Use a BufferedReader to read string from console */ import java.io.*; class ReadConsoleInput { public static void main(String args[]) throws IOException { /* create a BufferedReader using System.in */ BufferedReader br = new BufferedReader(new InputStreamReader(System.in)); String str; System.out.println("Enter some lines of text (enter 'stop' to quit) : "); // read string now do { str = br.readLine(); System.out.println(str); } while(!str.equals("stop")); } }
Now the next program creates a tiny text editor. It creates an array of String objects and then reads in lines of text, storing each line in the array. It will read up to 80 lines or until you enter "stop". It uses a BufferedReader to read from the console.
/* Java Program Example - Read Console Input in Java * Its a small text editor */ import java.io.*; class ReadConsoleInput { public static void main(String args[]) throws IOException { /* create a BufferedReader using System.in */ BufferedReader br = new BufferedReader(new InputStreamReader(System.in)); String str[] = new String[80]; System.out.println("Enter some lines of text (Enter 'stop' to quit) : "); for(int i=0; i<80; i++) { str[i] = br.readLine(); if(str[i].equals("stop")) { break; } } System.out.println("\nHere is you file :"); /* now display the lines */ for(int i=0; i<80; i++) { if(str[i].equals("stop")) { break; } System.out.println(str[i]); } } }
Below is sample run of the above Java program :
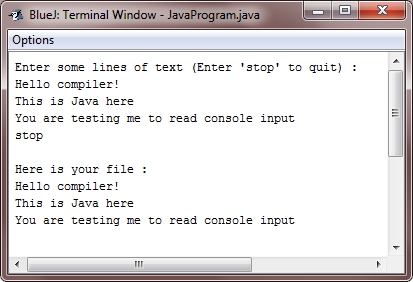
Examples on Files in Java
Here are some examples related to files in Java, you can go for.
- Read File in Java
- Write to File Java
- Read & Display File in Java
- Copy File in Java
- Merge two Files in Java
- List files in a Directory Java
- Delete File in Java
« Previous Tutorial Next Tutorial »