- Java Programming Basics
- Java Tutorial
- Java Overview
- Java Environment Setup
- Java Program Structure
- Java Basic Syntax
- Java First Program
- Java Constants
- Java Separators
- Java Keywords
- Java Data Types
- Java Data Types
- Java Integers
- Java Floating Point
- Java Characters
- Java Booleans
- Java Numbers
- Java Programming Variables
- Java Variables
- Java Variable Types
- Java Variable Scope
- Java Type Conversion
- Java Type Casting
- Java Auto Type Promotion
- Java Type Promotion Rules
- Java Programming Arrays
- Java Arrays
- Java One Dimensional Array
- Java Multidimensional Array
- Java Programming Operators
- Java Operators
- Java Arithmetic Operators
- Java Increment Decrement
- Java Bitwise Operators
- Java Left Shift
- Java Right Shift
- Java Relational Operators
- Java Boolean Logical Operators
- Java Ternary(?) Operator
- Java Operator Precedence
- Java Control Statements
- Java Decision Making
- Java if if-else if-else-if
- Java switch Statement
- Java Loops
- Java while Loop
- Java do-while Loop
- Java for Loop
- Java for-each Loop
- Java Nested Loops
- Java break Statement
- Java continue Statement
- Java Class Object Method
- Java Classes and Objects
- Java Class
- Java Object
- Java new Operator
- Java Methods
- Java Constructors
- Java this Keyword
- Java Stack
- Java Overloading Recursion
- Java Method Overloading
- Java Constructor Overloading
- Java Object as Parameter
- Java Call by Value Reference
- Java Returning Objects
- Java Recursion
- Java Modifier Types
- Java Encapsulate Poly String
- Java Encapsulation
- Java Polymorphism
- Java Nested Inner Class
- Java Strings
- Java Command Line Arguments
- Java Variable Length Arguments
- Java Inheritance Abstraction
- Java Inheritance
- Java super Superclass
- Java Multilevel Hierarchy
- Java Method Overriding
- Java Abstraction
- Java Packages Interfaces
- Java Packages
- Java Access Protection
- Java Import Statement
- Java Interfaces
- Java Programming Exceptions
- Java Exception Handling
- Java try catch
- Java throw throws
- Java finally Block
- Java Built In Exceptions
- Java Exception Subclasses
- Java Chained Exceptions
- Java Multithreading
- Java Multithreading
- Java Thread Model
- Java Main Thread
- Java Create Thread
- Java Thread Priorities
- Java Synchronization
- Java Inter Thread Communication
- Java Suspend Resume Stop Thread
- Java Get Thread State
- Java Enum Autobox Annotation
- Java Enumerations
- Java Type Wrappers
- Java Autoboxing
- Java Annotation
- Java Marker Annotations
- Java Single Member Annotation
- Java Built In Annotations
- Java Type Annotations
- Java Repeating Annotations
- Java Data File Handling
- Java Files I/O
- Java Streams
- Java Read Console Input
- Java Write Console Output
- Java PrintWriter Class
- Java Read Write Files
- Java Automatically Close File
- Java Programming Advance
- Java Date and Time
- Java Regular Expressions
- Java Collections Framework
- Java Generics
- Java Data Structures
- Java Network Programming
- Java Serialization
- Java Send Email
- Java Applet Basics
- Java Documentation
- Java Programming Examples
- Java Programming Examples
Java Operators
Java provides a rich operator environment. Most of its operators can be divided into the following four groups :
You will learn all the Java operators in the following groups:
- Arithmetic Operators
- Bitwise Operators
- Relational Operators
- Logical Operators
- Assignment Operators
- Misc Operators
Let's discuss about all these operators one by one.
Java Arithmetic Operators
Arithmetic operators are used for mathematical expressions. You will learn in detail about arithmetic operators in separate chapter.
Java Bitwise Operators
Bitwise operator works on bits and performs bit-by-bit operation. You will learn in detail about bitwise operator in separate chapter.
Java Relational Operator
Relational operator operates on relationship that one operand has to the other. You will learn in detail about relational operator in separate chapter.
Java Logical Operator
Boolean Logical operator operate only on boolean operands. You will learn in detail about logical operator.
Java Assignment Operators
There are following assignment operators in Java language:
Operator | Name | Meaning |
---|---|---|
= | Simple Assignment Operator | This operators is used to assign the values from the right side operands to the left side operand |
-= | Subtract and Assignment Operator | This operator simply subtracts right operand from the left operand and assign the result to the left operand |
+= | Add and Assignment Operator | This operator simply adds the right operand to the left operand and assign the result to the left operand |
/= | Divide and Assignment Operator | This operator is simply divides the left operand with the right operand and assign the result to the left operand |
*= | Multiply and Assignment Operator | This operator is simply multiplies the right operand with the left operand and assign the result to the left operand |
%= | Modulus and Assignment Operator | This operator simply takes modulus using the two operands and assign the result to the left operand |
>>= | Right Shift and Assignment Operator | This operator is used to shift right the value and then assign the result to the left operand |
<<= | Left Shift and Assignment Operator | This operator is used to left shift the value and then assign the result to the left operand |
^= | Bitwise Exclusive OR and Assignment Operator | This is a bitwise exclusive OR and assignment operator, takes the exclusive OR of the of the value and then assign the result to the left operand |
&= | Bitwise and Assignment Operator | This is a bitwise AND and assignment operator, takes the bitwise AND of the value and then assign the result to the left operand |
|= | Bitwise Inclusive OR and Assignment Operator | This is a bitwise inclusive OR and assignment operator, takes the inclusive OR of the value, and then assign the value to the left operand |
Example
Look at the following example program which demonstrates the assignment operators:
/* Java Program Example - Java Operators * Java Assignment Operators */ public class JavaProgram { public static void main(String args[]) { int num1 = 10, num2 = 20, res = 0; res = num1 + num2; System.out.println("res = num1 + num2 = " + res); res += num1 ; System.out.println("res += num1 = " + res); res -= num1 ; System.out.println("res -= num1 = " + res); res *= num1 ; System.out.println("res *= num1 = " + res); num1 = 10; res = 15; res /= num1 ; System.out.println("res /= num1 = " + res); num1 = 10; res = 15; res %= num1 ; System.out.println("res %= num1 = " + res); res <<= 2 ; System.out.println("res <<= 2 = " + res); res >>= 2 ; System.out.println("res >>= 2 = " + res); res >>= 2 ; System.out.println("res >>= num1 = " + res); res &= num1 ; System.out.println("res &= 2 = " + res); res ^= num1 ; System.out.println("res ^= num1 = " + res); res |= num1 ; System.out.println("res |= num1 = " + res); } }
Here is the sample output of the above Java program:
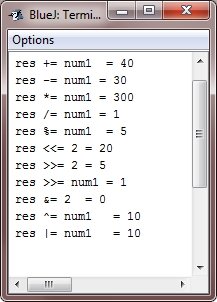
Java Misc Operators
There are also some other miscellaneous operators available in Java language.
Java Conditional Operator ( ? : )
Conditional operator is also known as the ternary operator. You will learn in detail about ternary operator in separate chapter.
Java instanceof Operator
The instanceof operator in Java, used only for the object reference variables. This operator basically checks whether the object is of a specific type (class or interface type). Here is the general form to use instanceof operator in Java language:
(Object reference variable) instanceof (class/interface type)
Example
/* Java Program Example - Java Operators * Java instanceof Operator */ public class JavaProgram { public static void main(String args[]) { String str_name = "James"; boolean res = str_name instanceof String; System.out.println(res); } }
Here is the output produced by the above Java program:
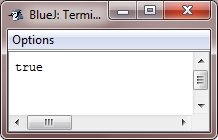
« Previous Tutorial Next Tutorial »