- Java Programming Basics
- Java Tutorial
- Java Overview
- Java Environment Setup
- Java Program Structure
- Java Basic Syntax
- Java First Program
- Java Constants
- Java Separators
- Java Keywords
- Java Data Types
- Java Data Types
- Java Integers
- Java Floating Point
- Java Characters
- Java Booleans
- Java Numbers
- Java Programming Variables
- Java Variables
- Java Variable Types
- Java Variable Scope
- Java Type Conversion
- Java Type Casting
- Java Auto Type Promotion
- Java Type Promotion Rules
- Java Programming Arrays
- Java Arrays
- Java One Dimensional Array
- Java Multidimensional Array
- Java Programming Operators
- Java Operators
- Java Arithmetic Operators
- Java Increment Decrement
- Java Bitwise Operators
- Java Left Shift
- Java Right Shift
- Java Relational Operators
- Java Boolean Logical Operators
- Java Ternary(?) Operator
- Java Operator Precedence
- Java Control Statements
- Java Decision Making
- Java if if-else if-else-if
- Java switch Statement
- Java Loops
- Java while Loop
- Java do-while Loop
- Java for Loop
- Java for-each Loop
- Java Nested Loops
- Java break Statement
- Java continue Statement
- Java Class Object Method
- Java Classes and Objects
- Java Class
- Java Object
- Java new Operator
- Java Methods
- Java Constructors
- Java this Keyword
- Java Stack
- Java Overloading Recursion
- Java Method Overloading
- Java Constructor Overloading
- Java Object as Parameter
- Java Call by Value Reference
- Java Returning Objects
- Java Recursion
- Java Modifier Types
- Java Encapsulate Poly String
- Java Encapsulation
- Java Polymorphism
- Java Nested Inner Class
- Java Strings
- Java Command Line Arguments
- Java Variable Length Arguments
- Java Inheritance Abstraction
- Java Inheritance
- Java super Superclass
- Java Multilevel Hierarchy
- Java Method Overriding
- Java Abstraction
- Java Packages Interfaces
- Java Packages
- Java Access Protection
- Java Import Statement
- Java Interfaces
- Java Programming Exceptions
- Java Exception Handling
- Java try catch
- Java throw throws
- Java finally Block
- Java Built In Exceptions
- Java Exception Subclasses
- Java Chained Exceptions
- Java Multithreading
- Java Multithreading
- Java Thread Model
- Java Main Thread
- Java Create Thread
- Java Thread Priorities
- Java Synchronization
- Java Inter Thread Communication
- Java Suspend Resume Stop Thread
- Java Get Thread State
- Java Enum Autobox Annotation
- Java Enumerations
- Java Type Wrappers
- Java Autoboxing
- Java Annotation
- Java Marker Annotations
- Java Single Member Annotation
- Java Built In Annotations
- Java Type Annotations
- Java Repeating Annotations
- Java Data File Handling
- Java Files I/O
- Java Streams
- Java Read Console Input
- Java Write Console Output
- Java PrintWriter Class
- Java Read Write Files
- Java Automatically Close File
- Java Programming Advance
- Java Date and Time
- Java Regular Expressions
- Java Collections Framework
- Java Generics
- Java Data Structures
- Java Network Programming
- Java Serialization
- Java Send Email
- Java Applet Basics
- Java Documentation
- Java Programming Examples
- Java Programming Examples
Java Chained Exceptions
With chained exceptions feature, you can associate another exception with an exception. The second exception accounts the cause of first exception. For instance, imagine a situation in which a method throws an ArithmeticException because of an attempt to divide-by-zero. Nevertheless, the actual cause of problem was that an I/O error occurred, which caused the divisor to be set improperly.
Even though the method must certainly throw an ArithmeticException, since that is the error that occurred, you might want to let the calling code know that the underlying cause was an I/O error. Chained exceptions let you to manage this, and any other situation in which layers of exceptions exist.
How to allow Chained Exceptions in Java ?
To allow chained exceptions in Java, two constructors and two methods were added to the Throwable. The constructors are shown here:
Throwable(Throwable causeExc) Throwable(String msg, Throwable causeExc)
In the first form, causeExc is the exception, causes the current exception i.e., causeExc is underlying reason that an exception occurred. And the second form allows you to specify a description at the same time that you specify a cause exception. These two constructors have been added to the Error , Exception, and RuntimeException classes also.
The methods getCause() and initCause() are the chained exception methods that supported by Throwable. These methods are shown in table (in the previous chapter) and are repeated here for the sake of discussion.
Throwable getCause() Throwable initCause(Throwable causeExc)
The method getCause() returns the exception that underlies the current exception. If there is no underlying exception, null is returned.
The method initCause() associates causeExc with the invoking exception and returns a reference to the exception. Therefore, you can associate a cause with an exception after the exception has been created. Nevertheless, the cause exception can be set only once. Therefore, you can call the initCause() only once for each exception object. Moreover, if the cause exception was set by a constructor, then you can not set it again using the initCause().
In general, the initCause() is utilized to set a cause for legacy exception classes that don't support the two additional constructors described earlier.
Java Chained Exception Example
Below is an example program that demonstrates the mechanics of handling the chained exceptions
/* Java Program Example - Java Chained Exceptions * This program demonstrates the exception chaining */ class JavaProgram { static void demometh() { /* creating an exception */ NullPointerException e = new NullPointerException("top layer"); /* add a cause */ e.initCause(new ArithmeticException("cause")); throw e; } public static void main(String args[]) { try { demometh(); } catch(NullPointerException e) { /* display the top level exception */ System.out.println("caught : " + e); /* display the cause exception */ System.out.println("original cause : " + e.getCause()); } } }
When the above Java program is compile and executed, it will produce the following output:
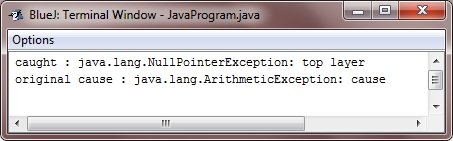
In this example, NullPointerException is the top-level exception. To it is added a cause exception, ArithmeticException. When the exception is thrown out of demometh() method, it is caught by the main(). There, the top-level exception is displayed, followed by underlying exception, which is obtained by calling the method getCause().
Chained exceptions can be carried on to whatever depth is necessary. Therefore, the cause exception can, itself, have a cause. Be aware that overly long chains of exceptions may show poor design.
Chained exceptions are not something that every program will need. However, in cases in which knowledge of an underlying cause is useful, then they offer an elegant solution.
« Previous Tutorial Next Tutorial »