- Java Programming Basics
- Java Tutorial
- Java Overview
- Java Environment Setup
- Java Program Structure
- Java Basic Syntax
- Java First Program
- Java Constants
- Java Separators
- Java Keywords
- Java Data Types
- Java Data Types
- Java Integers
- Java Floating Point
- Java Characters
- Java Booleans
- Java Numbers
- Java Programming Variables
- Java Variables
- Java Variable Types
- Java Variable Scope
- Java Type Conversion
- Java Type Casting
- Java Auto Type Promotion
- Java Type Promotion Rules
- Java Programming Arrays
- Java Arrays
- Java One Dimensional Array
- Java Multidimensional Array
- Java Programming Operators
- Java Operators
- Java Arithmetic Operators
- Java Increment Decrement
- Java Bitwise Operators
- Java Left Shift
- Java Right Shift
- Java Relational Operators
- Java Boolean Logical Operators
- Java Ternary(?) Operator
- Java Operator Precedence
- Java Control Statements
- Java Decision Making
- Java if if-else if-else-if
- Java switch Statement
- Java Loops
- Java while Loop
- Java do-while Loop
- Java for Loop
- Java for-each Loop
- Java Nested Loops
- Java break Statement
- Java continue Statement
- Java Class Object Method
- Java Classes and Objects
- Java Class
- Java Object
- Java new Operator
- Java Methods
- Java Constructors
- Java this Keyword
- Java Stack
- Java Overloading Recursion
- Java Method Overloading
- Java Constructor Overloading
- Java Object as Parameter
- Java Call by Value Reference
- Java Returning Objects
- Java Recursion
- Java Modifier Types
- Java Encapsulate Poly String
- Java Encapsulation
- Java Polymorphism
- Java Nested Inner Class
- Java Strings
- Java Command Line Arguments
- Java Variable Length Arguments
- Java Inheritance Abstraction
- Java Inheritance
- Java super Superclass
- Java Multilevel Hierarchy
- Java Method Overriding
- Java Abstraction
- Java Packages Interfaces
- Java Packages
- Java Access Protection
- Java Import Statement
- Java Interfaces
- Java Programming Exceptions
- Java Exception Handling
- Java try catch
- Java throw throws
- Java finally Block
- Java Built In Exceptions
- Java Exception Subclasses
- Java Chained Exceptions
- Java Multithreading
- Java Multithreading
- Java Thread Model
- Java Main Thread
- Java Create Thread
- Java Thread Priorities
- Java Synchronization
- Java Inter Thread Communication
- Java Suspend Resume Stop Thread
- Java Get Thread State
- Java Enum Autobox Annotation
- Java Enumerations
- Java Type Wrappers
- Java Autoboxing
- Java Annotation
- Java Marker Annotations
- Java Single Member Annotation
- Java Built In Annotations
- Java Type Annotations
- Java Repeating Annotations
- Java Data File Handling
- Java Files I/O
- Java Streams
- Java Read Console Input
- Java Write Console Output
- Java PrintWriter Class
- Java Read Write Files
- Java Automatically Close File
- Java Programming Advance
- Java Date and Time
- Java Regular Expressions
- Java Collections Framework
- Java Generics
- Java Data Structures
- Java Network Programming
- Java Serialization
- Java Send Email
- Java Applet Basics
- Java Documentation
- Java Programming Examples
- Java Programming Examples
Java if if-else if-else-if Statement
In Java, the if statement is conditional branch statement. It can be used to route the program execution through two different paths. Here is the general form to use if statement in Java language:
if(condition) statement1; else statement2;
Here, each statement may be a single or compound statement enclosed in the curly braces (a block). The condition is any expression that returns a boolean value. The else clause is optional.
Java if Statement Working
The working of if statement is as follows:
if the condition is true, then statement1 is executed. Otherwise, the statement2 (if it will present) is executed. In none case will both the statements be executed.
For example, consider the following :
int a, b; //... if(a < b) a = 0; else b = 0;
Here, if a is less than b, then a is set to zero. Otherwise, b is set to zero. In no case are they both set to zero.
Usually, the expression used to control the if statement will involve the relational operators. Though, this is not technically necessary. It is possible to control the if using a single boolean variable, as shown in the following code fragment :
boolean dataAvailable; //... if(dataAvailable) ProcessData(); else waitForMoreData();
Remember, only one statement can appear directly after the if or the else. If you want to include more statements, you will use to create a block, as shown in the following code fragment :
int bytesAvailable; //... if(bytesAvailable > 0) { ProcessData(); bytesAvailable -= n; } else waitForMoreData();
Here, both the statements in the if block will execute if bytesAvailable is greater than zero.
Some programmers find it favorable to include the curly braces when using the if statement, even when there is only one statement in each clause. It makes it easy to add another statement later, and you don't have to worry about forgetting the braces.
Java Nested ifs
A nested if is an if statement that is the target of another if or else.
Nested ifs are very common in programming. When you nest ifs, the important thing to remember is that an else statement always refers to the nearest if statement that is in the same block as the else and that is not already associated with an else. Following is an example:
if(i == 10) { if(j < 20) a = b; if(k > 100) c = d; // this if is else // associated with this else a = c; } else a = d; // this else refers to if(i == 10)
Don't become confuse with the curly braces. Naturally if there is more than one block of code then include curly braces. Otherwise don't. Here is the improved version of the above program using curly braces :
if(i == 10) { if(j < 20) { a = b; } if(k > 100) { c = d; } else { a = c; } } else { a = d; }
As the comments indicator, the final else is not associated with if(j<20) as it is not in the same block (even though it is the nearest if without an else). Instead, the final else is associated with if(i==10). The inner else refers to the if(k>100) because it is the closest if within the same block.
Java if-else-if Ladder
A general programming construct that is based upon a sequence of nested ifs is if-else-if ladder. Here is the general form of if-else-if ladder in Java:
if(condition) statement; else if(condition) statement; else if(condition) statement . . . else statement;
The if statements are executed from the top down. As soon as one of the conditions controlling the if is true, the statement related with that if is executed, and the rest of the ladder is bypassed.
If none of the conditions is true, then the last else statement will be executed. The final else acts as a default condition; that is, if all the other conditional tests fail, then the final else statement is performed. If there is no final else and all the other conditions are false, then no action will take place.
Java if-else-if Example
Following is a program that uses the if-else-if ladder to determine which season a particular month is in :
/* Java Program Example - Java if Statement * This program demonstrates the if-else-if statements */ public class JavaProgram { public static void main(String args[]) { int month = 4; // April String season; if(month == 12 || month == 1 || month == 2) { season = "Winter"; } else if(month == 3 || month == 4 || month == 5) { season = "Spring"; } else if(month == 6 || month == 7 || month == 8) { season = "Summer"; } else if(month == 9 || month == 10 || month == 11) { season = "Autumn"; } else { season = "Bogus Month"; } System.out.println("April is in the " + season); } }
When the above Java program is compile and executed, it will produce the following output:
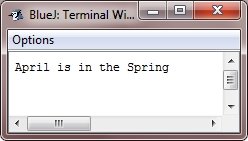
From the above program, you will find, no matter what value you give month, there is one and only one assignment statement within the ladder will be executed.
More Examples
Here are some list of example programs that uses the if statement:
- Check Even or Odd
- Check Prime or Not
- Check Alphabet or Not
- Check Vowel or Not
- Check Leap Year or Not
- Check Anagram or Not
« Previous Tutorial Next Tutorial »