- Java Programming Basics
- Java Tutorial
- Java Overview
- Java Environment Setup
- Java Program Structure
- Java Basic Syntax
- Java First Program
- Java Constants
- Java Separators
- Java Keywords
- Java Data Types
- Java Data Types
- Java Integers
- Java Floating Point
- Java Characters
- Java Booleans
- Java Numbers
- Java Programming Variables
- Java Variables
- Java Variable Types
- Java Variable Scope
- Java Type Conversion
- Java Type Casting
- Java Auto Type Promotion
- Java Type Promotion Rules
- Java Programming Arrays
- Java Arrays
- Java One Dimensional Array
- Java Multidimensional Array
- Java Programming Operators
- Java Operators
- Java Arithmetic Operators
- Java Increment Decrement
- Java Bitwise Operators
- Java Left Shift
- Java Right Shift
- Java Relational Operators
- Java Boolean Logical Operators
- Java Ternary(?) Operator
- Java Operator Precedence
- Java Control Statements
- Java Decision Making
- Java if if-else if-else-if
- Java switch Statement
- Java Loops
- Java while Loop
- Java do-while Loop
- Java for Loop
- Java for-each Loop
- Java Nested Loops
- Java break Statement
- Java continue Statement
- Java Class Object Method
- Java Classes and Objects
- Java Class
- Java Object
- Java new Operator
- Java Methods
- Java Constructors
- Java this Keyword
- Java Stack
- Java Overloading Recursion
- Java Method Overloading
- Java Constructor Overloading
- Java Object as Parameter
- Java Call by Value Reference
- Java Returning Objects
- Java Recursion
- Java Modifier Types
- Java Encapsulate Poly String
- Java Encapsulation
- Java Polymorphism
- Java Nested Inner Class
- Java Strings
- Java Command Line Arguments
- Java Variable Length Arguments
- Java Inheritance Abstraction
- Java Inheritance
- Java super Superclass
- Java Multilevel Hierarchy
- Java Method Overriding
- Java Abstraction
- Java Packages Interfaces
- Java Packages
- Java Access Protection
- Java Import Statement
- Java Interfaces
- Java Programming Exceptions
- Java Exception Handling
- Java try catch
- Java throw throws
- Java finally Block
- Java Built In Exceptions
- Java Exception Subclasses
- Java Chained Exceptions
- Java Multithreading
- Java Multithreading
- Java Thread Model
- Java Main Thread
- Java Create Thread
- Java Thread Priorities
- Java Synchronization
- Java Inter Thread Communication
- Java Suspend Resume Stop Thread
- Java Get Thread State
- Java Enum Autobox Annotation
- Java Enumerations
- Java Type Wrappers
- Java Autoboxing
- Java Annotation
- Java Marker Annotations
- Java Single Member Annotation
- Java Built In Annotations
- Java Type Annotations
- Java Repeating Annotations
- Java Data File Handling
- Java Files I/O
- Java Streams
- Java Read Console Input
- Java Write Console Output
- Java PrintWriter Class
- Java Read Write Files
- Java Automatically Close File
- Java Programming Advance
- Java Date and Time
- Java Regular Expressions
- Java Collections Framework
- Java Generics
- Java Data Structures
- Java Network Programming
- Java Serialization
- Java Send Email
- Java Applet Basics
- Java Documentation
- Java Programming Examples
- Java Programming Examples
Java continue Statement
On certain occasions, it is useful to force an early iteration of the loop i.e., you might want to continue running the loop but stop processing the remainder of code in its body for this particular iteration. This is, in effect, a goto just pass the body of the loop to the end of the loop. The continue statement performs such an action.
In while and do-while loops, the continue statement causes a control to be transferred directly to the conditional expression that controls the loop. In the for loop, the control goes first to the iteration portion of the for statement and then to the conditional expression. And for all three loops (for, while, and do-while), any intermediate code is bypassed.
Java continue Statement Example
Here is an example program that uses the continue statement to cause the two numbers to be printed on each line :
/* Java Program Example - Java continue Statement * This program demonstrates the continue keyword */ public class JavaProgram { public static void main(String args[]) { for(int i=0; i<10; i++) { System.out.print(i + " "); if(i%2 == 0) continue; System.out.println(); } } }
When the above Java program is compile and executed, it will produce the following output:
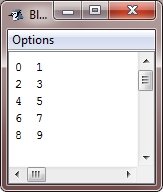
As with the break statement, continue may define a label to report which enclosing loop to continue. Here is an example program that uses the continue keyword to print the triangular multiplication table for 0 though 9 :
/* Java Program Example - Java continue Statement * Using continue with a label */ public class JavaProgram { public static void main(String args[]) { outer: for(int i=0; i<10; i++) { for(int j=0; j<10; j++) { if(j > i) { System.out.println(); continue outer; } System.out.print(" " + (i * j)); } } System.out.println(); } }
When the above Java program is compile and executed, it will produce the following output:
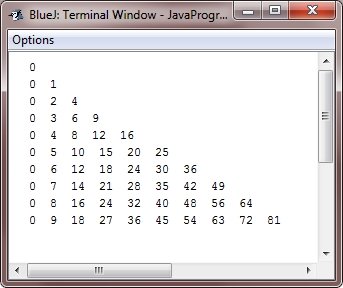
The continue statement in above example terminates the loop counting j and then continues with the next iteration of the loop counting i.
« Previous Tutorial Next Tutorial »