- Java Programming Basics
- Java Tutorial
- Java Overview
- Java Environment Setup
- Java Program Structure
- Java Basic Syntax
- Java First Program
- Java Constants
- Java Separators
- Java Keywords
- Java Data Types
- Java Data Types
- Java Integers
- Java Floating Point
- Java Characters
- Java Booleans
- Java Numbers
- Java Programming Variables
- Java Variables
- Java Variable Types
- Java Variable Scope
- Java Type Conversion
- Java Type Casting
- Java Auto Type Promotion
- Java Type Promotion Rules
- Java Programming Arrays
- Java Arrays
- Java One Dimensional Array
- Java Multidimensional Array
- Java Programming Operators
- Java Operators
- Java Arithmetic Operators
- Java Increment Decrement
- Java Bitwise Operators
- Java Left Shift
- Java Right Shift
- Java Relational Operators
- Java Boolean Logical Operators
- Java Ternary(?) Operator
- Java Operator Precedence
- Java Control Statements
- Java Decision Making
- Java if if-else if-else-if
- Java switch Statement
- Java Loops
- Java while Loop
- Java do-while Loop
- Java for Loop
- Java for-each Loop
- Java Nested Loops
- Java break Statement
- Java continue Statement
- Java Class Object Method
- Java Classes and Objects
- Java Class
- Java Object
- Java new Operator
- Java Methods
- Java Constructors
- Java this Keyword
- Java Stack
- Java Overloading Recursion
- Java Method Overloading
- Java Constructor Overloading
- Java Object as Parameter
- Java Call by Value Reference
- Java Returning Objects
- Java Recursion
- Java Modifier Types
- Java Encapsulate Poly String
- Java Encapsulation
- Java Polymorphism
- Java Nested Inner Class
- Java Strings
- Java Command Line Arguments
- Java Variable Length Arguments
- Java Inheritance Abstraction
- Java Inheritance
- Java super Superclass
- Java Multilevel Hierarchy
- Java Method Overriding
- Java Abstraction
- Java Packages Interfaces
- Java Packages
- Java Access Protection
- Java Import Statement
- Java Interfaces
- Java Programming Exceptions
- Java Exception Handling
- Java try catch
- Java throw throws
- Java finally Block
- Java Built In Exceptions
- Java Exception Subclasses
- Java Chained Exceptions
- Java Multithreading
- Java Multithreading
- Java Thread Model
- Java Main Thread
- Java Create Thread
- Java Thread Priorities
- Java Synchronization
- Java Inter Thread Communication
- Java Suspend Resume Stop Thread
- Java Get Thread State
- Java Enum Autobox Annotation
- Java Enumerations
- Java Type Wrappers
- Java Autoboxing
- Java Annotation
- Java Marker Annotations
- Java Single Member Annotation
- Java Built In Annotations
- Java Type Annotations
- Java Repeating Annotations
- Java Data File Handling
- Java Files I/O
- Java Streams
- Java Read Console Input
- Java Write Console Output
- Java PrintWriter Class
- Java Read Write Files
- Java Automatically Close File
- Java Programming Advance
- Java Date and Time
- Java Regular Expressions
- Java Collections Framework
- Java Generics
- Java Data Structures
- Java Network Programming
- Java Serialization
- Java Send Email
- Java Applet Basics
- Java Documentation
- Java Programming Examples
- Java Programming Examples
Java Overview
As in all the other computer programming languages, the elements of Java do not exist in isolation. Rather they work together to form the language as a whole.
Yet, this interrelatedness can make it difficult to describe one view of Java without involving the several others. Sometimes a discussion of one feature implies prior knowledge of another. For this cause, this tutorial presents a quick overview of several key features of Java. Let's start with OOP.
Object-Oriented Programming
Object-oriented programming (OOP) is at the core of Java. In fact, all the Java programs are to at least some extent object oriented. OOP is thus integral to Java that it is best to understand its basic principles before you begin writing even simple Java programs.
Two Paradigms
All computer programs consist of the two elements i.e., code and data. Moreover, a program can be organized around its code or around its data in a conceptual manner i.e., some programs are written around "what's going on ?" and other programs are written about "who's being affected ?". These are the two paradigms that govern how a program is constructed. The first style is called as the process-oriented-model. This approach characterized a program as a series of one-dimensional steps i.e., code. The process-oriented model can be supposed of as code acting on data.
To manage increasing complexity, the second approach is called as object-oriented-programming, was conceived. Object-oriented programming organizes a program around its data, objects and a set of well defined interfaces to that data. An object-oriented program can be described as data controlling access to the code.
Abstraction
An crucial element of object-oriented programming is abstraction. Humans manage complexity through the abstraction.
A powerful way to manage abstraction, by the use of hierarchical classifications. This allows you to layer the linguistics of complex systems, breaking them into more manageable pieces.
The Three OOP Principles
All the object-oriented programming languages provide mechanisms which helps you to implement the object-oriented model. Examples are encapsulation, inheritance, and polymorphism.
Encapsulation
Encapsulation is the process which binds together the code and the data it manipulates, and keeps both safe from the outside interference and misuse. One way to guess about encapsulation is as a protective wrapper that prevents the code and the data from being arbitrarily accessed by the other code that defined outside the wrapper. Access to the code and data inside the wrapper is tightly controlled by a well-defined interface.
Inheritance
Inheritance is the process by which one object adopts the properties of another objects. This is important because it supports the concept of hierarchical classification.
Polymorphism
Polymorphism (from Greek, meaning "many forms") is a characteristic which allows one interface to be used for a general class of actions. The particular action is decided by the exact nature of the situation.
Polymorphism, Encapsulation, and Inheritance Work Together
When properly applied, polymorphism, encapsulation, and inheritance put together to produce a programming environment that supports the development of far more robust and scalable programs which does the process-oriented model.
A well-designed hierarchy of classes is the basis of reusing the code in which you have invested the time and effort in developing and testing. Encapsulation allows you to migrate your implementations over the time without breaking the code that depends on the public interface of your classes. Polymorphism lets you to create code readable, clean, sensible, and resilient.
A First Simple Program
Now that the basic of object-oriented support of Java has been discussed. Now let's look at the simple Java program:
/* Java Overview - Example Program */ public class JavaProgram { /* A Java Program Begins with a call to main() */ public static void main(String args[]) { System.out.println("This is a Simple Java Program."); } }
When the above Java program is compile and executed, it will produce the following output:
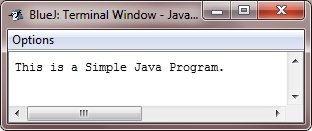
In next chapter, you will learn how to setup environment for Java programming to compile and run Java programs.
« Previous Tutorial Next Tutorial »