- Java Programming Basics
- Java Tutorial
- Java Overview
- Java Environment Setup
- Java Program Structure
- Java Basic Syntax
- Java First Program
- Java Constants
- Java Separators
- Java Keywords
- Java Data Types
- Java Data Types
- Java Integers
- Java Floating Point
- Java Characters
- Java Booleans
- Java Numbers
- Java Programming Variables
- Java Variables
- Java Variable Types
- Java Variable Scope
- Java Type Conversion
- Java Type Casting
- Java Auto Type Promotion
- Java Type Promotion Rules
- Java Programming Arrays
- Java Arrays
- Java One Dimensional Array
- Java Multidimensional Array
- Java Programming Operators
- Java Operators
- Java Arithmetic Operators
- Java Increment Decrement
- Java Bitwise Operators
- Java Left Shift
- Java Right Shift
- Java Relational Operators
- Java Boolean Logical Operators
- Java Ternary(?) Operator
- Java Operator Precedence
- Java Control Statements
- Java Decision Making
- Java if if-else if-else-if
- Java switch Statement
- Java Loops
- Java while Loop
- Java do-while Loop
- Java for Loop
- Java for-each Loop
- Java Nested Loops
- Java break Statement
- Java continue Statement
- Java Class Object Method
- Java Classes and Objects
- Java Class
- Java Object
- Java new Operator
- Java Methods
- Java Constructors
- Java this Keyword
- Java Stack
- Java Overloading Recursion
- Java Method Overloading
- Java Constructor Overloading
- Java Object as Parameter
- Java Call by Value Reference
- Java Returning Objects
- Java Recursion
- Java Modifier Types
- Java Encapsulate Poly String
- Java Encapsulation
- Java Polymorphism
- Java Nested Inner Class
- Java Strings
- Java Command Line Arguments
- Java Variable Length Arguments
- Java Inheritance Abstraction
- Java Inheritance
- Java super Superclass
- Java Multilevel Hierarchy
- Java Method Overriding
- Java Abstraction
- Java Packages Interfaces
- Java Packages
- Java Access Protection
- Java Import Statement
- Java Interfaces
- Java Programming Exceptions
- Java Exception Handling
- Java try catch
- Java throw throws
- Java finally Block
- Java Built In Exceptions
- Java Exception Subclasses
- Java Chained Exceptions
- Java Multithreading
- Java Multithreading
- Java Thread Model
- Java Main Thread
- Java Create Thread
- Java Thread Priorities
- Java Synchronization
- Java Inter Thread Communication
- Java Suspend Resume Stop Thread
- Java Get Thread State
- Java Enum Autobox Annotation
- Java Enumerations
- Java Type Wrappers
- Java Autoboxing
- Java Annotation
- Java Marker Annotations
- Java Single Member Annotation
- Java Built In Annotations
- Java Type Annotations
- Java Repeating Annotations
- Java Data File Handling
- Java Files I/O
- Java Streams
- Java Read Console Input
- Java Write Console Output
- Java PrintWriter Class
- Java Read Write Files
- Java Automatically Close File
- Java Programming Advance
- Java Date and Time
- Java Regular Expressions
- Java Collections Framework
- Java Generics
- Java Data Structures
- Java Network Programming
- Java Serialization
- Java Send Email
- Java Applet Basics
- Java Documentation
- Java Programming Examples
- Java Programming Examples
Java Inheritance
Inheritance is one of the base of object-oriented programming because it allows the creation of hierarchical classifications.
With inheritance, you can create a general class that defines traits common to the set of related items. This class can then be inherited by the other, more specific classes, each adding those things that are unique to it.
In nomenclature of Java, a class which is inherited is known as a superlcass. The class that does the inheriting is called a subclass. Thus, a subclass is a specialized version of a superclass. It inherits all of the members defined by the superclass and then adds its own, unique elements.
Java Inheritance Example
To inherit a class, you incorporate the definition of one class into another by using the keyword extends. To see how, let's take a look at the following short example. Here this program creates a superclass named A and a subclass named B. Notice here that how the extends keyword is used to create a subclass of A.
/* Java Program Example - Java Inheritance * This is a simple example program of inheritance. */ /* create a superclass */ class A { int i, j; void showij() { System.out.println("i and j : " + i + " " + j); } } /* create a subclass by extending the class A */ class B extends A { int k; void showk() { System.out.println("k : " + k); } void sum() { System.out.println("i+j+k = " + (i+j+k)); } } class JavaProgram { public static void main(String args[]) { A superObj = new A(); B subObj = new B(); /* the superclass may be used by itself */ superObj.i = 100; superObj.j = 200; System.out.println("Contents of superObj : "); superObj.showij(); System.out.println(); /* the subclass has access to all the public * members of its superclass. */ subObj.i = 70; subObj.j = 80; subObj.k = 90; System.out.println("Contents of subObj : "); subObj.showij(); subObj.showk(); System.out.println(); System.out.println("Sum of i, j, and k in subObj : "); subObj.sum(); } }
When the above Java program is compile and executed, it will produce the following output:
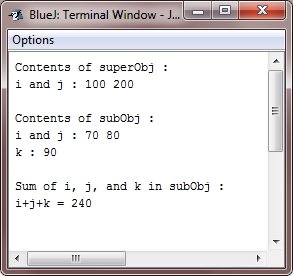
As you can see that the subclass B includes all of the members of its superclass, A. This is why subObj can access i and j and call the showij() method. Also, inside sum(), i and j can be referred to directly, as if they were part of B.
Even though A is a superclass for B, it is also completely independent, stand-alone class. Being a superclass for a subclass doesn't mean that the superclass can't be used by itself. Further, a subclass can be a superclass for another subclass.
Here is the general form of class declaration which inherits a superclass :
class subclass-name extends superclass-name { // body of the class }
You can only specify one superclass for any subclass which you create. Java does not support the inheritance of multiple superclasses into a single subclass. You can create a hierarchy of inheritance in which a subclass becomes a superclass of another subclass. However, no class can be a superclass of itself.
« Previous Tutorial Next Tutorial »