- Java Programming Basics
- Java Tutorial
- Java Overview
- Java Environment Setup
- Java Program Structure
- Java Basic Syntax
- Java First Program
- Java Constants
- Java Separators
- Java Keywords
- Java Data Types
- Java Data Types
- Java Integers
- Java Floating Point
- Java Characters
- Java Booleans
- Java Numbers
- Java Programming Variables
- Java Variables
- Java Variable Types
- Java Variable Scope
- Java Type Conversion
- Java Type Casting
- Java Auto Type Promotion
- Java Type Promotion Rules
- Java Programming Arrays
- Java Arrays
- Java One Dimensional Array
- Java Multidimensional Array
- Java Programming Operators
- Java Operators
- Java Arithmetic Operators
- Java Increment Decrement
- Java Bitwise Operators
- Java Left Shift
- Java Right Shift
- Java Relational Operators
- Java Boolean Logical Operators
- Java Ternary(?) Operator
- Java Operator Precedence
- Java Control Statements
- Java Decision Making
- Java if if-else if-else-if
- Java switch Statement
- Java Loops
- Java while Loop
- Java do-while Loop
- Java for Loop
- Java for-each Loop
- Java Nested Loops
- Java break Statement
- Java continue Statement
- Java Class Object Method
- Java Classes and Objects
- Java Class
- Java Object
- Java new Operator
- Java Methods
- Java Constructors
- Java this Keyword
- Java Stack
- Java Overloading Recursion
- Java Method Overloading
- Java Constructor Overloading
- Java Object as Parameter
- Java Call by Value Reference
- Java Returning Objects
- Java Recursion
- Java Modifier Types
- Java Encapsulate Poly String
- Java Encapsulation
- Java Polymorphism
- Java Nested Inner Class
- Java Strings
- Java Command Line Arguments
- Java Variable Length Arguments
- Java Inheritance Abstraction
- Java Inheritance
- Java super Superclass
- Java Multilevel Hierarchy
- Java Method Overriding
- Java Abstraction
- Java Packages Interfaces
- Java Packages
- Java Access Protection
- Java Import Statement
- Java Interfaces
- Java Programming Exceptions
- Java Exception Handling
- Java try catch
- Java throw throws
- Java finally Block
- Java Built In Exceptions
- Java Exception Subclasses
- Java Chained Exceptions
- Java Multithreading
- Java Multithreading
- Java Thread Model
- Java Main Thread
- Java Create Thread
- Java Thread Priorities
- Java Synchronization
- Java Inter Thread Communication
- Java Suspend Resume Stop Thread
- Java Get Thread State
- Java Enum Autobox Annotation
- Java Enumerations
- Java Type Wrappers
- Java Autoboxing
- Java Annotation
- Java Marker Annotations
- Java Single Member Annotation
- Java Built In Annotations
- Java Type Annotations
- Java Repeating Annotations
- Java Data File Handling
- Java Files I/O
- Java Streams
- Java Read Console Input
- Java Write Console Output
- Java PrintWriter Class
- Java Read Write Files
- Java Automatically Close File
- Java Programming Advance
- Java Date and Time
- Java Regular Expressions
- Java Collections Framework
- Java Generics
- Java Data Structures
- Java Network Programming
- Java Serialization
- Java Send Email
- Java Applet Basics
- Java Documentation
- Java Programming Examples
- Java Programming Examples
Java Exception Handling
In Java, an exception is an unnatural condition that arises in a code sequence at run time. In other words, an exception is a run-time error.
In those computer languages which don't support the exception handling, then the errors must be checked and handled manually.
Exception handling in Java, brings run-time error management into the object-oriented world.
In Java exception is an object which describes an exceptional (i.e., error) condition that has occurred in a piece of code.
What if an exception arises ?
When an exceptional condition arises, then an object representing that exception is created and thrown in the method that caused the error. That method might choose to handle the exception itself, or pass it on. Either way, at several point, the exception is caught and processed.
How exception can be generated ?
Exceptions can be generated through the Java's run-time system, or they can be generated by your code manually.
Exceptions thrown by Java, relate to the fundamental errors that violate the Java language's rules or the constraints of the execution environment of Java. Manually generated exceptions are generally used to report some error condition to the caller of a method.
How exception can be manage ?
Java's exception handling is managed via the following five keywords :
- try
- catch
- throw
- throws
- finally
Program statements which you want to monitor for exceptions are contained within the try block. If an exception occurs inside a try block, it is thrown. Your code can catch this exceptions using the catch and handle it in some rational manner. The System-generated exceptions are automatically thrown by the Java's run-time system. To manually throw an exception, use the throw keyword. Any exception that is thrown out of a method must be specified as such by the throws clause. Any code that must be executed after a try block completes is put in a finally block.
Java Exception Handling Syntax
Here is the general form of Java exception handling block :
try { // block of the code to monitor for the errors } catch(ExceptionType1 exObj) { // exception handler for ExceptionType1 } catch(ExceptionType2 exObj) { // exception handler for ExceptionType2 } // ... finally { // block of the code to be executed after try block ends }
Here, ExceptionType is the type of exception that has occurred.
Java Exception Types
All the exception types are subclasses of the Throwable built-in class. Therefore, Throwable is at the top of the exception class hierarchy. Just below Throwable are two subclasses that partition exceptions into the two distinct branches. One branch is headed by Exception. This class is used for the exceptional conditions that user programs should catch. This is also the class which you will subclass in creating your own custom exception types. There is an important subclass of Exception, called the RuntimeException. Exceptions of this type are automatically defined for the programs that you write and include the things like division by zero and invalid array indexing.
The other branch is topped by Error, which defines exceptions that are not expected to be caught under the normal circumstances by your program. Exceptions of the type Error are used by Java's run-time system to indicate the errors having to do with the run-time environment, itself. Stack overflow is an example of such an error. We will not deal with exceptions of the type Error, because these are typically created in response to ruinous failures that cannot usually be handled by your program.
The top-level exception hierarchy is shown in this figure :
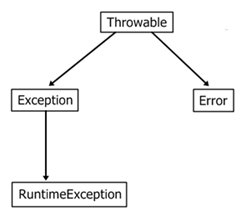
Java Uncaught Exceptions
Before you learn about how to handle exceptions in your Java program, it is useful to see what happens when you don't handle them. Here below small program includes an expression that intentionally causes a divide-by-zero error :
class Excp0 { public static void main(String args[]) { int denom = 0, num = 52; int res = num / denom; } }
When Java's run-time system detects the attempt to divide by zero, this constructs a new exception object and then throws this exception. This cause the execution of the Excp0 to stop, as once an exception has been thrown, it must be caught through an exception handler and dealt with immediately. In this example, we have not supplied any exception handlers of our own, therefore the exception is caught by the default handler that is provided by the Java's run-time system.
Any exception that is not caught by your program will finally be processed by the default handler. The default handler displays a string describing the exception, prints stack trace from the point at which the exception occurred, and terminates the program.
Below is the exception generated when this example is executed :
java.lang.ArithmeticException: / by zero at Excp0.main(Excp0.java:4)
Notice here that how the class name, Excp0,
the method name, main,
the filename, Excp0.java,
and the line number, 4,
are all included in simple stack trace. Also notice here that the type of exception thrown is a subclass of Exception called ArithmeticException,
which more especially describes what type of error happened.
The stack trace will always show the sequence of the method invocations which led up to the error. For instance, here is another version of the preceding program that simply introduces the same error but in a method separate from the main():
class Excp1 { static void dividemethod() { int denom = 0, num = 20; int res = num / denom; } public static void main(String args[]) { Excp1.dividemethod(); } }
The resulting stack trace from the default exception handler shows that how the entire call stack is displayed :
java.lang.ArithmeticException: / by zero at Excp1.dividemethod(Excp1.java:4) at Excp1.main(Excp1.java:7)
As you can see, the bottom of the stack is main's line 7, that is the call to the method named dividemethod(), which caused the exception at line 4. The call stack is quite useful for the debugging purpose, because it pinpoints the precise sequence of steps that led to the error.
« Previous Tutorial Next Tutorial »