- Java Programming Basics
- Java Tutorial
- Java Overview
- Java Environment Setup
- Java Program Structure
- Java Basic Syntax
- Java First Program
- Java Constants
- Java Separators
- Java Keywords
- Java Data Types
- Java Data Types
- Java Integers
- Java Floating Point
- Java Characters
- Java Booleans
- Java Numbers
- Java Programming Variables
- Java Variables
- Java Variable Types
- Java Variable Scope
- Java Type Conversion
- Java Type Casting
- Java Auto Type Promotion
- Java Type Promotion Rules
- Java Programming Arrays
- Java Arrays
- Java One Dimensional Array
- Java Multidimensional Array
- Java Programming Operators
- Java Operators
- Java Arithmetic Operators
- Java Increment Decrement
- Java Bitwise Operators
- Java Left Shift
- Java Right Shift
- Java Relational Operators
- Java Boolean Logical Operators
- Java Ternary(?) Operator
- Java Operator Precedence
- Java Control Statements
- Java Decision Making
- Java if if-else if-else-if
- Java switch Statement
- Java Loops
- Java while Loop
- Java do-while Loop
- Java for Loop
- Java for-each Loop
- Java Nested Loops
- Java break Statement
- Java continue Statement
- Java Class Object Method
- Java Classes and Objects
- Java Class
- Java Object
- Java new Operator
- Java Methods
- Java Constructors
- Java this Keyword
- Java Stack
- Java Overloading Recursion
- Java Method Overloading
- Java Constructor Overloading
- Java Object as Parameter
- Java Call by Value Reference
- Java Returning Objects
- Java Recursion
- Java Modifier Types
- Java Encapsulate Poly String
- Java Encapsulation
- Java Polymorphism
- Java Nested Inner Class
- Java Strings
- Java Command Line Arguments
- Java Variable Length Arguments
- Java Inheritance Abstraction
- Java Inheritance
- Java super Superclass
- Java Multilevel Hierarchy
- Java Method Overriding
- Java Abstraction
- Java Packages Interfaces
- Java Packages
- Java Access Protection
- Java Import Statement
- Java Interfaces
- Java Programming Exceptions
- Java Exception Handling
- Java try catch
- Java throw throws
- Java finally Block
- Java Built In Exceptions
- Java Exception Subclasses
- Java Chained Exceptions
- Java Multithreading
- Java Multithreading
- Java Thread Model
- Java Main Thread
- Java Create Thread
- Java Thread Priorities
- Java Synchronization
- Java Inter Thread Communication
- Java Suspend Resume Stop Thread
- Java Get Thread State
- Java Enum Autobox Annotation
- Java Enumerations
- Java Type Wrappers
- Java Autoboxing
- Java Annotation
- Java Marker Annotations
- Java Single Member Annotation
- Java Built In Annotations
- Java Type Annotations
- Java Repeating Annotations
- Java Data File Handling
- Java Files I/O
- Java Streams
- Java Read Console Input
- Java Write Console Output
- Java PrintWriter Class
- Java Read Write Files
- Java Automatically Close File
- Java Programming Advance
- Java Date and Time
- Java Regular Expressions
- Java Collections Framework
- Java Generics
- Java Data Structures
- Java Network Programming
- Java Serialization
- Java Send Email
- Java Applet Basics
- Java Documentation
- Java Programming Examples
- Java Programming Examples
Java throw and throws
Java throw Statement
Using the throw statement, it is possible to throw an exception explicitly for your program. Here is the general form to use throw statement in Java:
throw ThrowableInstance;
Here, ThrowableInstance must be an object of type Throwable or a subclass of Throwable.
Primitive types, such as int or char, as well as non-Throwable classes, such as String and Object, cannot be used as exceptions.
There are the two ways you can obtain a Throwable object, using a parameter in a catch clause or creating one with the new operator/keyword.
The flow of execution stops immediately after the throw statement, any subsequent statements are not executed. The nearest enclosing try block is inspected to see if it has a catch statement that matches the type of exception. If it does find a match, control is transferred to that statement. If not, then the next enclosing try statement is inspected, and so on. If no matching catch is found, the default exception handler halts the program and prints the stack trace.
Java throw Example
Following is a simple program that creates and throws an exception. The handler that catches the exception rethrows it to the outer handler.
/* Java Program Example - Java throw * This program demonstrate throw */ class JavaProgram { static void demometh() { try { throw new NullPointerException("demo"); } catch(NullPointerException e) { System.out.println("Caught inside demometh"); throw e; // rethrow the exception } } public static void main(String args[]) { try { demometh(); } catch(NullPointerException e) { System.out.println("Recaught : " + e); } } }
This program gets two chances to deal with the same error. First, the main() sets up an exception context and then calls the demometh(). The demometh() method then sets up another exception-handling context and immediately throws a new instance of NullPointerException, which is caught on the next line. The exception is then rethrown. Following is the resulting output:
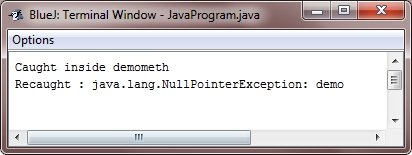
The program also illustrates how to create one of the Java's standard exception objects. Pay close attention to the following line :
throw new NullPointerException("demo");
Here, the new operator/keyword is used to construct an instance of NullPointerException.
Many of the Java's built-in run-time exceptions have at least two constructors, one with no parameter and the other that takes a string parameter. When the second form is used, the argument specifies a string that describes the exception. This string is displayed when the object is used as an argument to the print() or println(). It can also be obtained by a call to the getMessage(), which is defined by Throwable.
Java throws
If a method is capable of causing an exception that it does not handle, it must specify this behaviour so that the callers of the method can guard themselves against that exception. You can do this by including a throws clause in the method's declaration.
A throws clause lists the types of exceptions that a method might throw. This is necessary for all the exceptions, except those of type Error or RuntimeException, or any of their subclasses. All the other exceptions that a method can throw must be declared in throws clause. If they are not, a compile-time error will result.
Following is the general form of a method declaration that includes a throws clause :
type method-name(parameter-list) throws exception-list { // body of the method }
Here, exception-list is a comma-separated list of the exceptions that a method can throw.
Java throws Example
Following is an example of an incorrect program that tries to throw an exception that it does not catch. Because the program does not specify a throws clause to declare this fact, the program will not compile.
Java Programming Example Source Code (will not compile):/* Java Program Example - Java throws * This program contains an error and * will not compile */ class JavaProgram { static void demometh() { System.out.println("Inside demometh"); throw new IllegalAccessException("demo"); } public static void main(String args[]) { demometh(); } }
To make this program compile, you need to make the following two changes :
- You need to declare that demometh() throws IllegalAccessException.
- The main() must define a try / catch statement that catches this exception.
Now the corrected version of the previous program is shown below :
/* Java Program Example - Java throws * This program is the correct version * of the previous program */ class JavaProgram { static void demometh() throws IllegalAccessException { System.out.println("Inside demometh"); throw new IllegalAccessException("demo"); } public static void main(String args[]) { try { demometh(); } catch(IllegalAccessException e) { System.out.println("Caught " + e); } } }
When the above Java program is compile and executed, it will produce the following output:
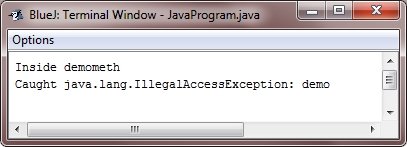
« Previous Tutorial Next Tutorial »