- Java Programming Basics
- Java Tutorial
- Java Overview
- Java Environment Setup
- Java Program Structure
- Java Basic Syntax
- Java First Program
- Java Constants
- Java Separators
- Java Keywords
- Java Data Types
- Java Data Types
- Java Integers
- Java Floating Point
- Java Characters
- Java Booleans
- Java Numbers
- Java Programming Variables
- Java Variables
- Java Variable Types
- Java Variable Scope
- Java Type Conversion
- Java Type Casting
- Java Auto Type Promotion
- Java Type Promotion Rules
- Java Programming Arrays
- Java Arrays
- Java One Dimensional Array
- Java Multidimensional Array
- Java Programming Operators
- Java Operators
- Java Arithmetic Operators
- Java Increment Decrement
- Java Bitwise Operators
- Java Left Shift
- Java Right Shift
- Java Relational Operators
- Java Boolean Logical Operators
- Java Ternary(?) Operator
- Java Operator Precedence
- Java Control Statements
- Java Decision Making
- Java if if-else if-else-if
- Java switch Statement
- Java Loops
- Java while Loop
- Java do-while Loop
- Java for Loop
- Java for-each Loop
- Java Nested Loops
- Java break Statement
- Java continue Statement
- Java Class Object Method
- Java Classes and Objects
- Java Class
- Java Object
- Java new Operator
- Java Methods
- Java Constructors
- Java this Keyword
- Java Stack
- Java Overloading Recursion
- Java Method Overloading
- Java Constructor Overloading
- Java Object as Parameter
- Java Call by Value Reference
- Java Returning Objects
- Java Recursion
- Java Modifier Types
- Java Encapsulate Poly String
- Java Encapsulation
- Java Polymorphism
- Java Nested Inner Class
- Java Strings
- Java Command Line Arguments
- Java Variable Length Arguments
- Java Inheritance Abstraction
- Java Inheritance
- Java super Superclass
- Java Multilevel Hierarchy
- Java Method Overriding
- Java Abstraction
- Java Packages Interfaces
- Java Packages
- Java Access Protection
- Java Import Statement
- Java Interfaces
- Java Programming Exceptions
- Java Exception Handling
- Java try catch
- Java throw throws
- Java finally Block
- Java Built In Exceptions
- Java Exception Subclasses
- Java Chained Exceptions
- Java Multithreading
- Java Multithreading
- Java Thread Model
- Java Main Thread
- Java Create Thread
- Java Thread Priorities
- Java Synchronization
- Java Inter Thread Communication
- Java Suspend Resume Stop Thread
- Java Get Thread State
- Java Enum Autobox Annotation
- Java Enumerations
- Java Type Wrappers
- Java Autoboxing
- Java Annotation
- Java Marker Annotations
- Java Single Member Annotation
- Java Built In Annotations
- Java Type Annotations
- Java Repeating Annotations
- Java Data File Handling
- Java Files I/O
- Java Streams
- Java Read Console Input
- Java Write Console Output
- Java PrintWriter Class
- Java Read Write Files
- Java Automatically Close File
- Java Programming Advance
- Java Date and Time
- Java Regular Expressions
- Java Collections Framework
- Java Generics
- Java Data Structures
- Java Network Programming
- Java Serialization
- Java Send Email
- Java Applet Basics
- Java Documentation
- Java Programming Examples
- Java Programming Examples
Java Bitwise Operators
Java provides several bitwise operators that can be applied to the integer types: long, int, short, char, and long. These operators act upon the individual bits of their operands.
Java Bitwise Operators List
The Java Bitwise Operators are summarized here in this table :
Operator | Name |
---|---|
~ | Bitwise unary NOT |
& | Bitwise AND |
| | Bitwise OR |
^ | Bitwise exclusive OR |
>> | Shift right |
>>> | Shift right zero fill |
<< | Shift left |
&= | Bitwise AND assignment |
|= | Bitwise OR assignment |
^= | Bitwise exclusive OR assignment |
>>= | Shift right assignment |
>>>= | Shift right zero fill assignment |
<<= | Shift left assignment |
Since, bitwise operators manipulate the bits within an integer, it is important to understand, what effects such manipulations might have on a value. Specifically, it is useful to know, how Java stores integer values and how it represents negative numbers. So, before proceeding, let's briefly review these two topics.
All integer types are represented by binary numbers of varying bit widths. For example, byte value for 42 in binary is 00101010, here each position represents a power of two, starting with 20 at the rightmost bit. The next bit position to the left would be 21, or 2, continuing towards the left with 22, or 4, then 8, 16, 32, and so on. So 42 has 1 bits set at the positions 1, 3, and 5 (counting from 0 at the right); thus, 42 is the sum of 21 + 23 + 25, which is 2 + 8 + 32.
All integer types (except char) are signed integers. This means that they can represent negative values as well as positive ones. Java uses encoding known as two's complement, which means that negative numbers are represented by inverting (converting 1's to 0's and vice-versa) all of the bits in a value, then adding 1 to the result. For example, -42 is represented by inverting all the bits in 42, or 00101010, which yields 11010101, then adding 1, which results in 11010110, or -42. To decode any negative numbers, first invert all of the bits, then add 1. For example, -42, or 11010110 inverted, yields 00101001, or 41, so when you add 1 you get 42.
The reason that Java (and most other computer languages) uses two's complement is easy to see when you consider the issue of zero crossing. Assuming a byte value, zero (0) is represented by 00000000. In one's complement, simply inverting all of the bits creates 11111111, that creates negative zero. The trouble is that negative zero is invalid in integer maths. This problem is solved by using the two's complement to represent the negative values. When using the two's complement, 1 is added to the complement, which producing 100000000. This produces a 1 bit too far to the left to fit back into the byte value, resulting in desired behavior, where -0 is the same as 0, and 11111111 is the encoding for -1. Although we used a byte value in the previous example, the same basic principle applies to all of Java's integer types.
Because Java uses two's complement to store the negative numbers, and because all integers are signed values in Java, applying the bitwise operators can easily produce an unexpected results. For example, turning on the high-order bit will cause the resulting value to be interpreted as negative number, whether this is what you intended or not. To avoid unpleasant surprises, just remember that high-order bit determines the sign of an integer, no matter how that high-order bit gets set.
The Bitwise Logical Operators
The bitwise logical operators are &, |, ^, and ~.
Here this table shows the outcome of each operation. In the discussion that follows, always keep in mind that the bitwise operators are applied to each individual bit within each operand.
A | B | A | B | A & B | A ^ B | ~A |
---|---|---|---|---|---|
0 | 0 | 0 | 0 | 0 | 1 |
1 | 0 | 1 | 0 | 1 | 0 |
0 | 1 | 1 | 0 | 1 | 1 |
1 | 1 | 1 | 1 | 0 | 0 |
The Bitwise NOT Operator
The bitwise NOT operator also called the bitwise complement, the unary NOT operator, ~, inverts all of the bits of its operand.
Example
For example, the number 42, which has this bit pattern:
00101010
becomes
11010101
after the NOT operator is applied.
The Bitwise AND Operator
The bitwise AND operator, &, produces a 1 bit if both the operands are also 1. A zero is produced in all the other cases.
Example
Following is an example that demonstrates the bitwise AND operator:
00101010 42 &00001111 15 _________ 00001010 10
The Bitwise OR Operator
The bitwise OR operator, |, combines bits such that if either of the bits in the operands is 1, then the resultant bit is 1.
Example
Following is an example that demonstrates the bitwise OR operator:
00101010 42 |00001111 15 _________ 00101111 47
The Bitwise XOR Operator
The bitwise XOR operator, ^, combines bits such that if exactly one operand is 1, then the result is 1. Otherwise, the result is zero.
Example
The following example shows the effect of the bitwise XOR (^) operator. This example also demonstrates a useful attribute of a XOR operation. Notice here, how the bit pattern of 42 is inverted wherever the second operand has a 1 bit. Wherever the second operand has 0 bit, the first operand is unchanged. You will find this property useful when performing some types of bit manipulations.
00101010 42 ^00001111 15 _________ 00100101 37
The Bitwise Logical Operators Example
The following program demonstrates the bitwise logical operators :
/* Java Program Example - Java Bitwise Operators * This program demonstrate the bitwise logical operators */ public class JavaProgram { public static void main(String args[]) { String binary[] = { "0000", "0001", "0010", "0011", "0100", "0101", "0110", "0111", "1000", "1001", "1010", "1011", "1100", "1110", "1111" }; int a = 3; // 0 + 2 + 1 or 0011 in binary int b = 6; // 4 + 2 + 0 or 0110 in binary int c = a | b; int d = a & b; int e = a ^ b; int f = (~a & b) | (a & ~b); int g = ~a & 0x0f; System.out.println(" a = " + binary[a]); System.out.println(" b = " + binary[b]); System.out.println(" a|b = " + binary[c]); System.out.println(" a&b = " + binary[d]); System.out.println(" a^b = " + binary[e]); System.out.println("(~a&b)|(a&~b) = " + binary[f]); System.out.println(" ~a = " + binary[g]); } }
When the above Java program is compile and executed, it will produce the following output:
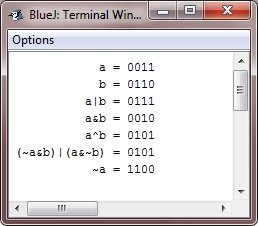
« Previous Tutorial Next Tutorial »