- Java Programming Basics
- Java Tutorial
- Java Overview
- Java Environment Setup
- Java Program Structure
- Java Basic Syntax
- Java First Program
- Java Constants
- Java Separators
- Java Keywords
- Java Data Types
- Java Data Types
- Java Integers
- Java Floating Point
- Java Characters
- Java Booleans
- Java Numbers
- Java Programming Variables
- Java Variables
- Java Variable Types
- Java Variable Scope
- Java Type Conversion
- Java Type Casting
- Java Auto Type Promotion
- Java Type Promotion Rules
- Java Programming Arrays
- Java Arrays
- Java One Dimensional Array
- Java Multidimensional Array
- Java Programming Operators
- Java Operators
- Java Arithmetic Operators
- Java Increment Decrement
- Java Bitwise Operators
- Java Left Shift
- Java Right Shift
- Java Relational Operators
- Java Boolean Logical Operators
- Java Ternary(?) Operator
- Java Operator Precedence
- Java Control Statements
- Java Decision Making
- Java if if-else if-else-if
- Java switch Statement
- Java Loops
- Java while Loop
- Java do-while Loop
- Java for Loop
- Java for-each Loop
- Java Nested Loops
- Java break Statement
- Java continue Statement
- Java Class Object Method
- Java Classes and Objects
- Java Class
- Java Object
- Java new Operator
- Java Methods
- Java Constructors
- Java this Keyword
- Java Stack
- Java Overloading Recursion
- Java Method Overloading
- Java Constructor Overloading
- Java Object as Parameter
- Java Call by Value Reference
- Java Returning Objects
- Java Recursion
- Java Modifier Types
- Java Encapsulate Poly String
- Java Encapsulation
- Java Polymorphism
- Java Nested Inner Class
- Java Strings
- Java Command Line Arguments
- Java Variable Length Arguments
- Java Inheritance Abstraction
- Java Inheritance
- Java super Superclass
- Java Multilevel Hierarchy
- Java Method Overriding
- Java Abstraction
- Java Packages Interfaces
- Java Packages
- Java Access Protection
- Java Import Statement
- Java Interfaces
- Java Programming Exceptions
- Java Exception Handling
- Java try catch
- Java throw throws
- Java finally Block
- Java Built In Exceptions
- Java Exception Subclasses
- Java Chained Exceptions
- Java Multithreading
- Java Multithreading
- Java Thread Model
- Java Main Thread
- Java Create Thread
- Java Thread Priorities
- Java Synchronization
- Java Inter Thread Communication
- Java Suspend Resume Stop Thread
- Java Get Thread State
- Java Enum Autobox Annotation
- Java Enumerations
- Java Type Wrappers
- Java Autoboxing
- Java Annotation
- Java Marker Annotations
- Java Single Member Annotation
- Java Built In Annotations
- Java Type Annotations
- Java Repeating Annotations
- Java Data File Handling
- Java Files I/O
- Java Streams
- Java Read Console Input
- Java Write Console Output
- Java PrintWriter Class
- Java Read Write Files
- Java Automatically Close File
- Java Programming Advance
- Java Date and Time
- Java Regular Expressions
- Java Collections Framework
- Java Generics
- Java Data Structures
- Java Network Programming
- Java Serialization
- Java Send Email
- Java Applet Basics
- Java Documentation
- Java Programming Examples
- Java Programming Examples
Java Boolean Logical Operators
The Boolean logical operators operates only on boolean operands. All of the binary logical operators combine two boolean values to form a resultant boolean value.
Boolean Logical Operators List
Here, the table given below, lists the boolean operators available in Java:
Operator | Name |
---|---|
& | Logical AND |
| | Logical OR |
^ | Logical XOR (exclusive OR) |
|| | Short-circuit OR |
&& | Short-circuit AND |
! | Logical unary NOT |
&= | AND assignment |
|= | OR assignment |
^= | XOR assignment |
== | Equal to |
!= | Not equal to |
?: | Ternary if-then-else |
The Boolean logical operators, &, |, and ^, operate on boolean value in the same way that they operate on the bits of an integer. The logical unary NOT (!) operator inverts the Boolean state: !true == false and !false == true.
Java Boolean Logical Operations Effect
Here this table shows the effect of each logical operation:
A | B | A | B | A & B | A ^ B | !A |
---|---|---|---|---|---|
False | False | False | False | False | True |
True | False | True | False | True | False |
False | True | True | False | True | True |
True | True | True | True | False | False |
Boolean Logical Operators Example
Here is an example program of boolean logical operators. Following program almost the same as the BitLogic example shown earlier (in the bitwise operator chapter), but it operate on boolean logical values instead of binary bits:
/* Java Program Example - Java Boolean Logical Operators * Demonstrate the boolean logical operators. */ public class JavaProgram { public static void main(String args[]) { boolean a = true; boolean b = false; boolean c = a | b; boolean d = a & b; boolean e = a ^ b; boolean f = (!a & b) | (a & !b); boolean g = !a; System.out.println(" a = " + a); System.out.println(" b = " + b); System.out.println(" a|b = " + c); System.out.println(" a&b = " + d); System.out.println(" a^b = " + e); System.out.println("(!a&b)|(a&!b) = " + f); System.out.println(" !a = " + g); } }
When the above Java program is compile and executed, it will produce the following output:
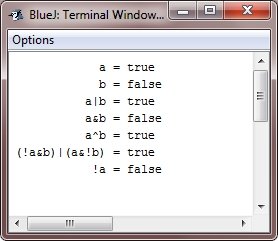
More Examples
Here are some examples listed based on some boolean logical operators, that you can go for. The Equal to (==) operator used most.
- Check Even or Odd
- Check Prime or Not
- Check Alphabet or Not
- Read File
- Read & Display File
- Copy File
- Merge two File
Here, don't be confuse with above some examples like reading file, writing to file etc. You will learn all one by one.
« Previous Tutorial Next Tutorial »