- Java Programming Basics
- Java Tutorial
- Java Overview
- Java Environment Setup
- Java Program Structure
- Java Basic Syntax
- Java First Program
- Java Constants
- Java Separators
- Java Keywords
- Java Data Types
- Java Data Types
- Java Integers
- Java Floating Point
- Java Characters
- Java Booleans
- Java Numbers
- Java Programming Variables
- Java Variables
- Java Variable Types
- Java Variable Scope
- Java Type Conversion
- Java Type Casting
- Java Auto Type Promotion
- Java Type Promotion Rules
- Java Programming Arrays
- Java Arrays
- Java One Dimensional Array
- Java Multidimensional Array
- Java Programming Operators
- Java Operators
- Java Arithmetic Operators
- Java Increment Decrement
- Java Bitwise Operators
- Java Left Shift
- Java Right Shift
- Java Relational Operators
- Java Boolean Logical Operators
- Java Ternary(?) Operator
- Java Operator Precedence
- Java Control Statements
- Java Decision Making
- Java if if-else if-else-if
- Java switch Statement
- Java Loops
- Java while Loop
- Java do-while Loop
- Java for Loop
- Java for-each Loop
- Java Nested Loops
- Java break Statement
- Java continue Statement
- Java Class Object Method
- Java Classes and Objects
- Java Class
- Java Object
- Java new Operator
- Java Methods
- Java Constructors
- Java this Keyword
- Java Stack
- Java Overloading Recursion
- Java Method Overloading
- Java Constructor Overloading
- Java Object as Parameter
- Java Call by Value Reference
- Java Returning Objects
- Java Recursion
- Java Modifier Types
- Java Encapsulate Poly String
- Java Encapsulation
- Java Polymorphism
- Java Nested Inner Class
- Java Strings
- Java Command Line Arguments
- Java Variable Length Arguments
- Java Inheritance Abstraction
- Java Inheritance
- Java super Superclass
- Java Multilevel Hierarchy
- Java Method Overriding
- Java Abstraction
- Java Packages Interfaces
- Java Packages
- Java Access Protection
- Java Import Statement
- Java Interfaces
- Java Programming Exceptions
- Java Exception Handling
- Java try catch
- Java throw throws
- Java finally Block
- Java Built In Exceptions
- Java Exception Subclasses
- Java Chained Exceptions
- Java Multithreading
- Java Multithreading
- Java Thread Model
- Java Main Thread
- Java Create Thread
- Java Thread Priorities
- Java Synchronization
- Java Inter Thread Communication
- Java Suspend Resume Stop Thread
- Java Get Thread State
- Java Enum Autobox Annotation
- Java Enumerations
- Java Type Wrappers
- Java Autoboxing
- Java Annotation
- Java Marker Annotations
- Java Single Member Annotation
- Java Built In Annotations
- Java Type Annotations
- Java Repeating Annotations
- Java Data File Handling
- Java Files I/O
- Java Streams
- Java Read Console Input
- Java Write Console Output
- Java PrintWriter Class
- Java Read Write Files
- Java Automatically Close File
- Java Programming Advance
- Java Date and Time
- Java Regular Expressions
- Java Collections Framework
- Java Generics
- Java Data Structures
- Java Network Programming
- Java Serialization
- Java Send Email
- Java Applet Basics
- Java Documentation
- Java Programming Examples
- Java Programming Examples
Java Constructor
Java allows objects to initialize themselves whenever they are created. This automatic initialization is performed by the use of a constructor.
A constructor initializes an object immediately upon creation. It has the same name as the class in which it resides and is syntactically same to a method. Once defined, the constructor is automatically called whenever an object is created, before the new operator completes.
Constructor look a little strange because they have no any return type, not even void. This is because the implicit return type of a class constructor is the class type itself. It's constructor's job to initialize the internal state of an object so that the code creating an instance will have a fully initialized, usable object immediately.
Java Constructor Example
Now let's start by defining a simple constructor that simply sets the dimensions of each box to the same values :
/* Java Program Example - Java Constructor * In this program, Box uses a constructor to * initialize the dimensions of a box */ class Box { double width; double height; double depth; /* this is the constructor for the Box */ Box() { System.out.println("Constructing Box"); width = 100; height = 100; depth = 100; } /* calculate and return the volume */ double volume() { return width * height * depth; } } class BoxDemo { public static void main(String args[]) { /* declare, allocate, and initialize the Box objects */ Box mybox1 = new Box(); Box mybox2 = new Box(); double vol; /* get the volume of the first box */ vol = mybox1.volume(); /* print the volume of the first box */ System.out.println("Volume of the first box is " + vol); /* get the volume of the second box */ vol = mybox2.volume(); /* print the volume of the second box */ System.out.println("Volume of the second box is " + vol); } }
When the above Java program is compile and executed, it will produce the following output:
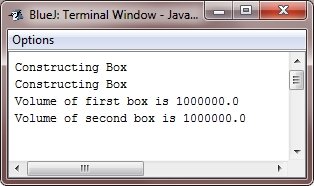
As you will see that both mybox1 and mybox2 were initialized by the Box() constructor when they were created. Since the constructor gives same dimensions to all the boxes, 100 by 100 by 100, both mybox1 and mybox2 will have the same volume. The statement println() inside the Box() is for the sake of illustrations only.
Most constructors will not display anything rather they will just initialized an object.
Before moving on, let's first examine the new operator. As you know, when you allocate an object, you use the below general form :
class-var = new classname();
Now you can understand why the parentheses are needed after the class name. What is actually going on is that the constructor for the class is being called. Thus, in the line
Box mybox1 = new Box();
new Box() is calling the constructor Box(). When you do not explicitly define a constructor for a class, then Java creates a default constructor for the class that's why the preceding line of code worked in earlier versions of Box that didn't define a constructor.
The default constructor automatically initializes all the instance variables to their default values, that are zero, null, and false, for numeric types, reference types, and boolean, respectively. The default constructor is often sufficient for the simple classes, but it usually won't do for more sophisticated ones. Once you define your own constructor, then the default constructor is no longer used.
Parameterized Constructor in Java
While the constructor Box() in the previous example does initialize a Box object, it is not very useful, all the boxes have the same dimensions. The simple solution is to add parameters to the constructor.
As you can likely guess, this makes it much more useful. For instance, here this version of Box defines a parameterized constructor that sets the dimension of a box as specified by those parameters. Pay special attention on how Box objects are created.
Java Parameterized Constructor Example
Here is an example program, illustrates the concept and use of parameterized constructor in Java:
/* Java Program Example - Java Constructors * In this program, Box uses a parameterized * constructor to initialize the dimensions * of a box */ class Box { double width; double height; double depth; /* this is the constructor for Box */ Box(double wid, double hei, double dep) { System.out.println("Constructing Box"); width = wid; height = hei; depth = dep; } /* compute and return the volume */ double volume() { return width * height * depth; } } class BoxDemo { public static void main(String args[]) { /* declare, allocate, and initialize Box objects */ Box mybox1 = new Box(100, 200, 150); Box mybox2 = new Box(30, 60, 90); double vol; /* get the volume of the first box */ vol = mybox1.volume(); /* print the volume of the first box */ System.out.println("Volume of the first box is " + vol); /* get the volume of the second box */ vol = mybox2.volume(); /* print the volume of the second box */ System.out.println("Volume of the second box is " + vol); } }
When the above Java program is compile and executed, it will produce the following output:
Above Java Programming Output: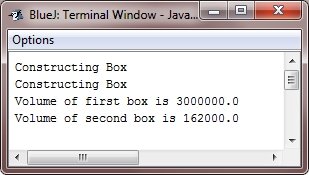
As you can see, the values 100, 200, and 150 are passed to the constructor Box() whenever new creates the object. Therefore, mybox1's copy of width, height, and depth will contain the values 100, 200, and 150, respectively.
« Previous Tutorial Next Tutorial »