- Java Programming Basics
- Java Tutorial
- Java Overview
- Java Environment Setup
- Java Program Structure
- Java Basic Syntax
- Java First Program
- Java Constants
- Java Separators
- Java Keywords
- Java Data Types
- Java Data Types
- Java Integers
- Java Floating Point
- Java Characters
- Java Booleans
- Java Numbers
- Java Programming Variables
- Java Variables
- Java Variable Types
- Java Variable Scope
- Java Type Conversion
- Java Type Casting
- Java Auto Type Promotion
- Java Type Promotion Rules
- Java Programming Arrays
- Java Arrays
- Java One Dimensional Array
- Java Multidimensional Array
- Java Programming Operators
- Java Operators
- Java Arithmetic Operators
- Java Increment Decrement
- Java Bitwise Operators
- Java Left Shift
- Java Right Shift
- Java Relational Operators
- Java Boolean Logical Operators
- Java Ternary(?) Operator
- Java Operator Precedence
- Java Control Statements
- Java Decision Making
- Java if if-else if-else-if
- Java switch Statement
- Java Loops
- Java while Loop
- Java do-while Loop
- Java for Loop
- Java for-each Loop
- Java Nested Loops
- Java break Statement
- Java continue Statement
- Java Class Object Method
- Java Classes and Objects
- Java Class
- Java Object
- Java new Operator
- Java Methods
- Java Constructors
- Java this Keyword
- Java Stack
- Java Overloading Recursion
- Java Method Overloading
- Java Constructor Overloading
- Java Object as Parameter
- Java Call by Value Reference
- Java Returning Objects
- Java Recursion
- Java Modifier Types
- Java Encapsulate Poly String
- Java Encapsulation
- Java Polymorphism
- Java Nested Inner Class
- Java Strings
- Java Command Line Arguments
- Java Variable Length Arguments
- Java Inheritance Abstraction
- Java Inheritance
- Java super Superclass
- Java Multilevel Hierarchy
- Java Method Overriding
- Java Abstraction
- Java Packages Interfaces
- Java Packages
- Java Access Protection
- Java Import Statement
- Java Interfaces
- Java Programming Exceptions
- Java Exception Handling
- Java try catch
- Java throw throws
- Java finally Block
- Java Built In Exceptions
- Java Exception Subclasses
- Java Chained Exceptions
- Java Multithreading
- Java Multithreading
- Java Thread Model
- Java Main Thread
- Java Create Thread
- Java Thread Priorities
- Java Synchronization
- Java Inter Thread Communication
- Java Suspend Resume Stop Thread
- Java Get Thread State
- Java Enum Autobox Annotation
- Java Enumerations
- Java Type Wrappers
- Java Autoboxing
- Java Annotation
- Java Marker Annotations
- Java Single Member Annotation
- Java Built In Annotations
- Java Type Annotations
- Java Repeating Annotations
- Java Data File Handling
- Java Files I/O
- Java Streams
- Java Read Console Input
- Java Write Console Output
- Java PrintWriter Class
- Java Read Write Files
- Java Automatically Close File
- Java Programming Advance
- Java Date and Time
- Java Regular Expressions
- Java Collections Framework
- Java Generics
- Java Data Structures
- Java Network Programming
- Java Serialization
- Java Send Email
- Java Applet Basics
- Java Documentation
- Java Programming Examples
- Java Programming Examples
Java Type Wrappers
As you know that Java uses the primitive types (simple types), such as int or double, to hold the basic data types supported by the language.
Primitive types, rather than objects, are used for these quantities for the sake of performance. Using objects for these values would add an unacceptable overhead to even the simplest of calculations. Thus, the primitive types are not part of the object hierarchy, and they do not inherit the Object.
Despite the performance benefit offered by the primitive types, there are times when you will need an object representation. For example, you can't pass the primitive type by reference to a method. Also, many of the standard data structures implemented by Java operate on objects, which means that you can't use these data structures to store the primitive types. To handle these and other situations, Java provides type wrappers, which are classes that encapsulate a primitive type within an object.
The type wrappers are Integer, Short, Double, Long, Float, Byte, Character, and Boolean. These classes offer a wide array of methods that allow you to fully integrate the primitive types into the Java's object hierarchy. Let's examine each.
Character
Character is a wrapper around a char. The constructor for the Character is
Character(char ch)
Here, ch specifies the character that will be wrapped by the Character object being created.
To obtain the char value contained in a Character object, call the charValue(), shown below :
char charValue()
It returns the encapsulated character.
Boolean
Boolean is a wrapper around the boolean values. It defines these constructors:
Boolean(boolean boolValue) Boolean(String boolString)
In the first version, boolValue must be either true or false. And in the second version, if boolString contains the string "true" (in uppercase or lowercase), then the new Boolean object will be true. Otherwise, it will be false.
To obtain a boolean value from a Boolean object, use booleanValue(), shown below :
boolean booleanValue()
It returns the boolean equivalent of the invoking object.
Java Numeric Type Wrappers
By far, the most commonly used type wrappers are those that represent the numeric values. These are Short, Byte, Integer, Float, Long, and Double. All of the numeric type wrappers inherit the abstract class Number. Numbers declares methods that return the value of an object in each of the different number formats. These methods are shown below :
byte byteValue() double doubleValue() float floatValue() int intValue() short shortValue() long longValue()
For example, doubleValue() returns the value of an object as a double, floatValue() returns the value as a float(), and so on. These methods are implemented by each of the numeric type wrappers.
All of the numeric type wrappers define the constructors that allow an object to be constructed from a given value, or a string representation of that value. For example, following are the constructors defined for the Integer :
Integer(int num) Integer(String str)
If str does not contain a valid numeric value, then a NumericFormatException is thrown.
All of the type wrappers override the toString(). It returns the human-readable form of the value contained within the wrapper. This allows you to output the value by passing a type wrapper object to the println().
Java Type Wrappers Example
The following program demonstrates how to use a numeric type wrapper to encapsulate a value and then extract that value:
/* Java Program Example - Java Type Wrappers * This program demonstrate a type wrapper */ class TypeWrapDemo { public static void main(String args[]) { Integer iObj = new Integer(10); int i = iObj.intValue(); System.out.println(i + " " + iObj); // display 10 10 } }
Above Java program produce the output :
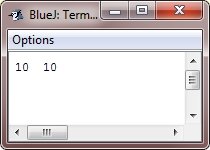
This program wraps the integer value 10 inside an Integer object called iObj. The program then obtains this value by calling the intValue() and stores the result in i.
The process of encapsulating a value within an object is called boxing. Thus, in the program, this lines boxes the value 10 into an Integer :
Integer iObj = new Integer(10);
The process of extracting a value from a type wrapper is called unboxing. For example, the program unboxes the value in iObj with this statement:
int i = iObj.intValue();
The same general procedure used by the preceding programs to box and unbox values has been employed since the original version of Java. However, since JDK 5, Java fundamentally improved on this through the addition of autoboxing (described in the next chapter).
« Previous Tutorial Next Tutorial »