- Java Programming Basics
- Java Tutorial
- Java Overview
- Java Environment Setup
- Java Program Structure
- Java Basic Syntax
- Java First Program
- Java Constants
- Java Separators
- Java Keywords
- Java Data Types
- Java Data Types
- Java Integers
- Java Floating Point
- Java Characters
- Java Booleans
- Java Numbers
- Java Programming Variables
- Java Variables
- Java Variable Types
- Java Variable Scope
- Java Type Conversion
- Java Type Casting
- Java Auto Type Promotion
- Java Type Promotion Rules
- Java Programming Arrays
- Java Arrays
- Java One Dimensional Array
- Java Multidimensional Array
- Java Programming Operators
- Java Operators
- Java Arithmetic Operators
- Java Increment Decrement
- Java Bitwise Operators
- Java Left Shift
- Java Right Shift
- Java Relational Operators
- Java Boolean Logical Operators
- Java Ternary(?) Operator
- Java Operator Precedence
- Java Control Statements
- Java Decision Making
- Java if if-else if-else-if
- Java switch Statement
- Java Loops
- Java while Loop
- Java do-while Loop
- Java for Loop
- Java for-each Loop
- Java Nested Loops
- Java break Statement
- Java continue Statement
- Java Class Object Method
- Java Classes and Objects
- Java Class
- Java Object
- Java new Operator
- Java Methods
- Java Constructors
- Java this Keyword
- Java Stack
- Java Overloading Recursion
- Java Method Overloading
- Java Constructor Overloading
- Java Object as Parameter
- Java Call by Value Reference
- Java Returning Objects
- Java Recursion
- Java Modifier Types
- Java Encapsulate Poly String
- Java Encapsulation
- Java Polymorphism
- Java Nested Inner Class
- Java Strings
- Java Command Line Arguments
- Java Variable Length Arguments
- Java Inheritance Abstraction
- Java Inheritance
- Java super Superclass
- Java Multilevel Hierarchy
- Java Method Overriding
- Java Abstraction
- Java Packages Interfaces
- Java Packages
- Java Access Protection
- Java Import Statement
- Java Interfaces
- Java Programming Exceptions
- Java Exception Handling
- Java try catch
- Java throw throws
- Java finally Block
- Java Built In Exceptions
- Java Exception Subclasses
- Java Chained Exceptions
- Java Multithreading
- Java Multithreading
- Java Thread Model
- Java Main Thread
- Java Create Thread
- Java Thread Priorities
- Java Synchronization
- Java Inter Thread Communication
- Java Suspend Resume Stop Thread
- Java Get Thread State
- Java Enum Autobox Annotation
- Java Enumerations
- Java Type Wrappers
- Java Autoboxing
- Java Annotation
- Java Marker Annotations
- Java Single Member Annotation
- Java Built In Annotations
- Java Type Annotations
- Java Repeating Annotations
- Java Data File Handling
- Java Files I/O
- Java Streams
- Java Read Console Input
- Java Write Console Output
- Java PrintWriter Class
- Java Read Write Files
- Java Automatically Close File
- Java Programming Advance
- Java Date and Time
- Java Regular Expressions
- Java Collections Framework
- Java Generics
- Java Data Structures
- Java Network Programming
- Java Serialization
- Java Send Email
- Java Applet Basics
- Java Documentation
- Java Programming Examples
- Java Programming Examples
Java Arithmetic and Modulus Operator
Arithmetic Operators
Arithmetic operators are used in the mathematical expressions in same way that they are used in algebra.
All Arithmetic Operators List
The following table lists all the arithmetic operators :
Operator | Name |
---|---|
+ | Addition (unary plus) |
- | Subtraction (unary minus) |
* | Multiplication |
/ | Division |
% | Modulus |
++ | Increment |
+= | Addition assignment |
-= | Subtraction assignment |
*= | Multiplication assignment |
/= | Division assignment |
%= | Modulus assignment |
-- | Decrement |
The operands in arithmetic operators must be of a numeric type. You cannot use them on boolean types, but you can use them on the char types, since the char type in Java is, essentially a subset of int.
Basic Arithmetic Operators
The basic arithmetic operations are:
- addition operator
- subtraction operator
- multiplication operator
- division operator
all acts as you would expect for all the numeric types.
The unary minus operator negates its single operand. The unary plus operator returns the value of its operand. Always remember that when the division operator is applied to an integer type, there will be no fractional component connected to the result.
Example
The following example program illustrates the arithmetic operators. It also illustrates the difference between floating-point division and integer division.
/* Java Program Example - Java Arithmetic Operators * This program demonstrates the basic arithmetic operators. */ public class JavaProgram { public static void main(String args[]) { /* arithmetic using integers */ System.out.println("Integer Arithmetic"); int a = 2 + 2; int b = a * 6; int c = b / 16; int d = c - a; int e = -d; System.out.println("a = " + a); System.out.println("b = " + b); System.out.println("c = " + c); System.out.println("d = " + d); System.out.println("e = " + c); /* arithmetic using doubles */ System.out.println("\nFloating Point Arithmetic"); double da = 2 + 2; double db = da * 6; double dc = db / 16; double dd = dc - da; double de = -dd; System.out.println("da = " + da); System.out.println("db = " + db); System.out.println("dc = " + dc); System.out.println("dd = " + dd); System.out.println("de = " + de); } }
When the above Java program is compile and executed, it will produce the following output:
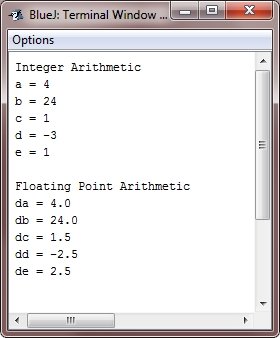
The Modulus Operator
The modulus operator (%) is a useful operator in Java, it returns the remainder of a division operation. It can be applied to the floating-point types and integer types both.
Example
The following example program illustrates the modulus operator (%) :
/* Java Program Example - Java Arithmetic Operators * This program demonstrates the modulus operator (%) */ public class JavaProgram { public static void main(String args[]) { int x = 46; double y = 46.25; System.out.println("46 mod 10 = " + x%10); System.out.println("46.25 mod 10 = " +y%10); } }
When the above Java program is compile and executed, it will produce the following output:
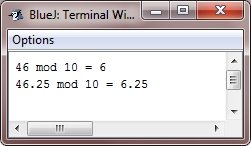
Arithmetic Compound Assignment Operators
Java allows special operators that can be used to combine an arithmetic operations with an assignment. As you probably know, statements like this are quite common in programming:
a = a + 4;
In Java, you can rewrite this statement as:
a += 4;
There are compound assignment operators for all of the arithmetic, binary operators. Thus, any statement of the form
var = var op expression;
can be rewritten as:
var op= expression;
Example
Following is a simple Java program that shows several op= assignment in action:
/* Java Program Example - Java Arithmetic Operators * This program demonstrates the several assignment operators. */ public class JavaProgram { public static void main(String args[]) { int a = 1; int b = 2; int c = 3; a += 10; b *= 4; c += a*b; c %= 6; System.out.println("a = " + a); System.out.println("b = " + b); System.out.println("c = " + c); } }
When the above Java program is compile and executed, it will produce the following output:
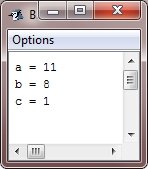
Example
Here is some examples based on arithmetic operators and modulus operator, that you can go for.
- Add two Numbers
- Addition, Subtraction, Multiplication, & Dividision
- Four-function Calculator
- Calculate Average and Percentage
- Calculate Arithmetic Mean
- Print Table of Number
- Add Digits of Number
« Previous Tutorial Next Tutorial »