- Java Programming Basics
- Java Tutorial
- Java Overview
- Java Environment Setup
- Java Program Structure
- Java Basic Syntax
- Java First Program
- Java Constants
- Java Separators
- Java Keywords
- Java Data Types
- Java Data Types
- Java Integers
- Java Floating Point
- Java Characters
- Java Booleans
- Java Numbers
- Java Programming Variables
- Java Variables
- Java Variable Types
- Java Variable Scope
- Java Type Conversion
- Java Type Casting
- Java Auto Type Promotion
- Java Type Promotion Rules
- Java Programming Arrays
- Java Arrays
- Java One Dimensional Array
- Java Multidimensional Array
- Java Programming Operators
- Java Operators
- Java Arithmetic Operators
- Java Increment Decrement
- Java Bitwise Operators
- Java Left Shift
- Java Right Shift
- Java Relational Operators
- Java Boolean Logical Operators
- Java Ternary(?) Operator
- Java Operator Precedence
- Java Control Statements
- Java Decision Making
- Java if if-else if-else-if
- Java switch Statement
- Java Loops
- Java while Loop
- Java do-while Loop
- Java for Loop
- Java for-each Loop
- Java Nested Loops
- Java break Statement
- Java continue Statement
- Java Class Object Method
- Java Classes and Objects
- Java Class
- Java Object
- Java new Operator
- Java Methods
- Java Constructors
- Java this Keyword
- Java Stack
- Java Overloading Recursion
- Java Method Overloading
- Java Constructor Overloading
- Java Object as Parameter
- Java Call by Value Reference
- Java Returning Objects
- Java Recursion
- Java Modifier Types
- Java Encapsulate Poly String
- Java Encapsulation
- Java Polymorphism
- Java Nested Inner Class
- Java Strings
- Java Command Line Arguments
- Java Variable Length Arguments
- Java Inheritance Abstraction
- Java Inheritance
- Java super Superclass
- Java Multilevel Hierarchy
- Java Method Overriding
- Java Abstraction
- Java Packages Interfaces
- Java Packages
- Java Access Protection
- Java Import Statement
- Java Interfaces
- Java Programming Exceptions
- Java Exception Handling
- Java try catch
- Java throw throws
- Java finally Block
- Java Built In Exceptions
- Java Exception Subclasses
- Java Chained Exceptions
- Java Multithreading
- Java Multithreading
- Java Thread Model
- Java Main Thread
- Java Create Thread
- Java Thread Priorities
- Java Synchronization
- Java Inter Thread Communication
- Java Suspend Resume Stop Thread
- Java Get Thread State
- Java Enum Autobox Annotation
- Java Enumerations
- Java Type Wrappers
- Java Autoboxing
- Java Annotation
- Java Marker Annotations
- Java Single Member Annotation
- Java Built In Annotations
- Java Type Annotations
- Java Repeating Annotations
- Java Data File Handling
- Java Files I/O
- Java Streams
- Java Read Console Input
- Java Write Console Output
- Java PrintWriter Class
- Java Read Write Files
- Java Automatically Close File
- Java Programming Advance
- Java Date and Time
- Java Regular Expressions
- Java Collections Framework
- Java Generics
- Java Data Structures
- Java Network Programming
- Java Serialization
- Java Send Email
- Java Applet Basics
- Java Documentation
- Java Programming Examples
- Java Programming Examples
Java while Loop
The while loop is the most fundamental loop statement of Java. It repeats a statement or block while its controlling expression is true. Here is the general form of while loop in Java:
while(condition) { // body of the loop }
The condition can be any Boolean expression. The body of the loop will be executed as long as the conditional expression is true. When the condition becomes false, the control passes to the next line of code immediately following the loop. The curly braces are unnecessary if only a single statement is being repeated.
Java while Loop Example
Following is a while loop that counts down from 10, printing exactly 10 lines of "tick" :
/* Java Program Example - Java while Loop * Demonstrate the while Loop */ public class JavaProgram { public static void main(String args[]) { int n = 10; while(n>0) { System.out.println("tick " + n); n--; } } }
When the above Java program is compile and executed, it will produce the following output:
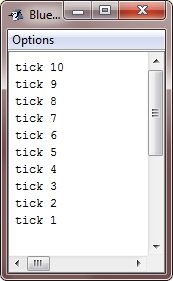
Since the while loop evaluates its conditional expression at the top of the loop, the body of the loop will not execute even once if the condition is false to begin with. For example, in the following code fragment, the call to println() is never executed:
int a = 10, b = 20; while(a > b) { System.out.println("This will not be displayed."); }
The body of the while loop can be empty. This is because a null statement (once that consists only of a semicolon) is syntactically valid in Java. For example, consider the following program:
/* Java Program Example - Java while Loop * The target of a loop can be empty */ public class JavaProgram { public static void main(String args[]) { int i, j; i = 100; j = 200; // find midpoint between i and j while(++i < --j); // there is no body in this loop System.out.println("Midpoint is " + i); } }
When the above Java program is compile and executed, it will produce the following output:
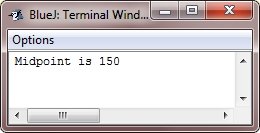
Example Explained
The value of i is incremented, and the value of j is decremented. These values are then compared with one another. If the new value of i is still less than the new value of j, then the loop repeats. If i is equal to or greater than j, the loop stops. Upon exit from the loop, i will hold a value that is midway between the original values of i and j. (Of course, this procedure only works when i is less than j to begin with.) As you can see, there is no need for a loop body, all of the action occurs within the conditional expression, itself. In professionally written Java code, short loops are frequently coded without bodies when the controlling expression can handle all of the details itself.
More Examples
Here are the list of some more examples, that you may like:
- Decimal to Binary Conversion
- Decimal to Octal Conversion
- Decimal to Hexadecimal Conversion
- Binary to Decimal Conversion
- Binary to Octal Conversion
- Binary to Hexadecimal Conversion
- Octal to Decimal Conversion
- Octal to Binary Conversion
- Octal to Hexadecimal Conversion
- Hexadecimal to Decimal Conversion
- Hexadecimal to Binary Conversion
- Hexadecimal to Octal Conversion
« Previous Tutorial Next Tutorial »