- Java Programming Basics
- Java Tutorial
- Java Overview
- Java Environment Setup
- Java Program Structure
- Java Basic Syntax
- Java First Program
- Java Constants
- Java Separators
- Java Keywords
- Java Data Types
- Java Data Types
- Java Integers
- Java Floating Point
- Java Characters
- Java Booleans
- Java Numbers
- Java Programming Variables
- Java Variables
- Java Variable Types
- Java Variable Scope
- Java Type Conversion
- Java Type Casting
- Java Auto Type Promotion
- Java Type Promotion Rules
- Java Programming Arrays
- Java Arrays
- Java One Dimensional Array
- Java Multidimensional Array
- Java Programming Operators
- Java Operators
- Java Arithmetic Operators
- Java Increment Decrement
- Java Bitwise Operators
- Java Left Shift
- Java Right Shift
- Java Relational Operators
- Java Boolean Logical Operators
- Java Ternary(?) Operator
- Java Operator Precedence
- Java Control Statements
- Java Decision Making
- Java if if-else if-else-if
- Java switch Statement
- Java Loops
- Java while Loop
- Java do-while Loop
- Java for Loop
- Java for-each Loop
- Java Nested Loops
- Java break Statement
- Java continue Statement
- Java Class Object Method
- Java Classes and Objects
- Java Class
- Java Object
- Java new Operator
- Java Methods
- Java Constructors
- Java this Keyword
- Java Stack
- Java Overloading Recursion
- Java Method Overloading
- Java Constructor Overloading
- Java Object as Parameter
- Java Call by Value Reference
- Java Returning Objects
- Java Recursion
- Java Modifier Types
- Java Encapsulate Poly String
- Java Encapsulation
- Java Polymorphism
- Java Nested Inner Class
- Java Strings
- Java Command Line Arguments
- Java Variable Length Arguments
- Java Inheritance Abstraction
- Java Inheritance
- Java super Superclass
- Java Multilevel Hierarchy
- Java Method Overriding
- Java Abstraction
- Java Packages Interfaces
- Java Packages
- Java Access Protection
- Java Import Statement
- Java Interfaces
- Java Programming Exceptions
- Java Exception Handling
- Java try catch
- Java throw throws
- Java finally Block
- Java Built In Exceptions
- Java Exception Subclasses
- Java Chained Exceptions
- Java Multithreading
- Java Multithreading
- Java Thread Model
- Java Main Thread
- Java Create Thread
- Java Thread Priorities
- Java Synchronization
- Java Inter Thread Communication
- Java Suspend Resume Stop Thread
- Java Get Thread State
- Java Enum Autobox Annotation
- Java Enumerations
- Java Type Wrappers
- Java Autoboxing
- Java Annotation
- Java Marker Annotations
- Java Single Member Annotation
- Java Built In Annotations
- Java Type Annotations
- Java Repeating Annotations
- Java Data File Handling
- Java Files I/O
- Java Streams
- Java Read Console Input
- Java Write Console Output
- Java PrintWriter Class
- Java Read Write Files
- Java Automatically Close File
- Java Programming Advance
- Java Date and Time
- Java Regular Expressions
- Java Collections Framework
- Java Generics
- Java Data Structures
- Java Network Programming
- Java Serialization
- Java Send Email
- Java Applet Basics
- Java Documentation
- Java Programming Examples
- Java Programming Examples
Java Constructor Overloading
In Java, you can also overload the constructor methods. In fact, for almost all real world classes that you create, overloaded constructors will be norm, not the exception. To realize why, let's return to the Box class developed in the previous chapter. Here is the latest version of the Box :
class Box { double width; double height; double depth; /* this is the constructor for the Box */ Box(double wid, double hei, double dep) { width = wid; height = hei; depth = dep; } /* compute and return the volume */ double volume() { return width * height * depth; } }
As you can see that the Box() constructor requires the three parameters. It means that all the declarations of the Box objects must pass the three arguments to the Box() constructor. For instance, here the below statement is currently invalid :
Box ob = new Box();
Since Box() requires the three arguments, therefore it's an error to call without them. This raises some important questions which can be:
- What if you just wanted a box and didn't know what its initial dimensions were ?
- What if you want to be able to initialize a cube by determining only one value that would be used for all the three dimensions ?
As the Box class is currently written, these options are not available to you.
Java Constructor Overloading Example
The solution to these problems is quite easy, just overload the Box constructor so that it handles the situations that described. Here is a program that contains an improved version of the Box that does just that :
/* Java Program Example - Java Constructor Overloading * In this program, Box defines the three constructors to * initialize the dimensions of a box various ways. */ class Box { double width; double height; double depth; /* constructor used when all the dimensions specified */ Box(double wid, double hei, double dep) { width = wid; height = hei; depth = dep; } /* constructor used when no dimensions specified */ Box() { width = -1; // use -1 to indicate height = -1; // an uninitialized depth = -1; // box } /* constructor used when the cube is created */ Box(double len) { width = height = depth = len; } /* compute and return the volume */ double volume() { return width * height * depth; } } class OverloadConstructor { public static void main(String args[]) { /* create boxes using the various constructors */ Box mybox1 = new Box(100, 200, 150); Box mybox2 = new Box(); Box mycube = new Box(7); double vol; /* get the volume of the first box */ vol = mybox1.volume(); /* print the volume of the first box */ System.out.println("Volume of mybox1 is " + vol); /* get the volume of the second box */ vol = mybox2.volume(); /* print the volume of the second box */ System.out.println("Volume of mybox2 is " + vol); /* get the volume of the cube */ vol = mycube.volume(); /* print the volume of the cube */ System.out.println("Volume of mycube is " + vol); } }
When the above Java program is compile and executed, it will produce the following output:
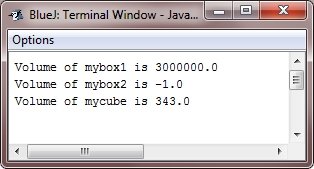
As you can see that the proper overloaded constructor is called based upon the parameters specified when new is executed.
« Previous Tutorial Next Tutorial »