- Java Programming Basics
- Java Tutorial
- Java Overview
- Java Environment Setup
- Java Program Structure
- Java Basic Syntax
- Java First Program
- Java Constants
- Java Separators
- Java Keywords
- Java Data Types
- Java Data Types
- Java Integers
- Java Floating Point
- Java Characters
- Java Booleans
- Java Numbers
- Java Programming Variables
- Java Variables
- Java Variable Types
- Java Variable Scope
- Java Type Conversion
- Java Type Casting
- Java Auto Type Promotion
- Java Type Promotion Rules
- Java Programming Arrays
- Java Arrays
- Java One Dimensional Array
- Java Multidimensional Array
- Java Programming Operators
- Java Operators
- Java Arithmetic Operators
- Java Increment Decrement
- Java Bitwise Operators
- Java Left Shift
- Java Right Shift
- Java Relational Operators
- Java Boolean Logical Operators
- Java Ternary(?) Operator
- Java Operator Precedence
- Java Control Statements
- Java Decision Making
- Java if if-else if-else-if
- Java switch Statement
- Java Loops
- Java while Loop
- Java do-while Loop
- Java for Loop
- Java for-each Loop
- Java Nested Loops
- Java break Statement
- Java continue Statement
- Java Class Object Method
- Java Classes and Objects
- Java Class
- Java Object
- Java new Operator
- Java Methods
- Java Constructors
- Java this Keyword
- Java Stack
- Java Overloading Recursion
- Java Method Overloading
- Java Constructor Overloading
- Java Object as Parameter
- Java Call by Value Reference
- Java Returning Objects
- Java Recursion
- Java Modifier Types
- Java Encapsulate Poly String
- Java Encapsulation
- Java Polymorphism
- Java Nested Inner Class
- Java Strings
- Java Command Line Arguments
- Java Variable Length Arguments
- Java Inheritance Abstraction
- Java Inheritance
- Java super Superclass
- Java Multilevel Hierarchy
- Java Method Overriding
- Java Abstraction
- Java Packages Interfaces
- Java Packages
- Java Access Protection
- Java Import Statement
- Java Interfaces
- Java Programming Exceptions
- Java Exception Handling
- Java try catch
- Java throw throws
- Java finally Block
- Java Built In Exceptions
- Java Exception Subclasses
- Java Chained Exceptions
- Java Multithreading
- Java Multithreading
- Java Thread Model
- Java Main Thread
- Java Create Thread
- Java Thread Priorities
- Java Synchronization
- Java Inter Thread Communication
- Java Suspend Resume Stop Thread
- Java Get Thread State
- Java Enum Autobox Annotation
- Java Enumerations
- Java Type Wrappers
- Java Autoboxing
- Java Annotation
- Java Marker Annotations
- Java Single Member Annotation
- Java Built In Annotations
- Java Type Annotations
- Java Repeating Annotations
- Java Data File Handling
- Java Files I/O
- Java Streams
- Java Read Console Input
- Java Write Console Output
- Java PrintWriter Class
- Java Read Write Files
- Java Automatically Close File
- Java Programming Advance
- Java Date and Time
- Java Regular Expressions
- Java Collections Framework
- Java Generics
- Java Data Structures
- Java Network Programming
- Java Serialization
- Java Send Email
- Java Applet Basics
- Java Documentation
- Java Programming Examples
- Java Programming Examples
Java Date and Time
Java Date Class
The Date class available in the java.util package, this class encapsulates the current date and time.
The Date class supports the two constructors. In which the first constructor initializes the object with the current date and time. Following is the syntax:
Date( )
The following constructor accepts one argument that equals the number of milliseconds that have elapsed since midnight, January 1, 1970
Date(long millisec)
Java Date and Time Example
Here are some examples on date and time in Java. Use Date object with method named toString() to print the current date/time.
/* Java Program Example - Java Date and Time * This program get the current date and time * and then display it on the screen */ import java.util.Date; class GetCurrentDateTime { public static void main(String args[]) { Date date = new Date(); System.out.println(date.toString()); } }
Here is the sample output produced by the above Java program:
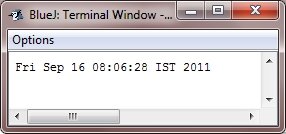
Format Date in Java
Here is an example program, showing how to format date in Java:
/* Java Program Example - Java Date and Time * This program format the date using the * SimpleDateFormat */ import java.util.*; import java.text.*; class JavaDateTime { public static void main(String args[]) { Date dob = new Date( ); SimpleDateFormat sdob = new SimpleDateFormat ("E yyyy.MM.dd 'at' hh:mm:ss a zzz"); System.out.println("Current Date : " + sdob.format(dob)); } }
Here is the output of the above Java program:
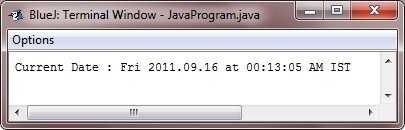
Here is another program to format date using printf in Java:
/* Java Program Example - Java Date and Time * This program format the date using the * printf() method */ import java.util.Date; class JavaDateTime { public static void main(String args[]) { Date date = new Date(); String sob = String.format("Current Date/Time : %tc", date); System.out.printf(sob); } }
Here is the output produced by the above Java program:
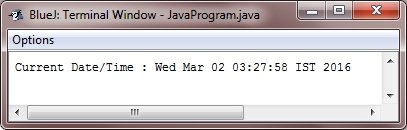
Here is an example showing how to parse strings into dates in Java:
/* Java Program Example - Java Date and Time * Parsing Strings into Dates */ import java.util.*; import java.text.*; class JavaDateTime { public static void main(String args[]) { SimpleDateFormat sdfob = new SimpleDateFormat ("yyyy-MM-dd"); String input = args.length == 0 ? "1818-11-11" : args[0]; System.out.print(input + " Parses as "); Date t; try { t = sdfob.parse(input); System.out.println(t); } catch (ParseException e) { System.out.println("Unparseable using " + sdfob); } } }
It will produce the following result:
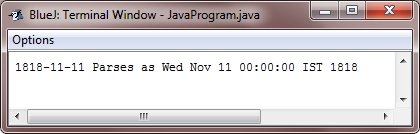
Here is an example showing how to sleep for a while in Java:
/* Java Program Example - Java Date and Time */ import java.util.*; class JavaDateTime { public static void main(String args[]) { try { System.out.println(new Date( ) + "\n"); Thread.sleep(5*60*10); System.out.println(new Date( ) + "\n"); } catch (Exception e) { System.out.println("Got an exception..!!"); } } }
Here is the sample output of this Java program:
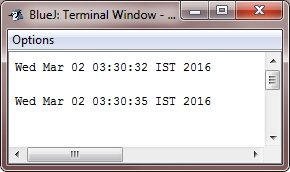
Measure Elapsed Time in Java
Here is an example showing how to measure an elapsed time in Java:
/* Java Program Example - Java Date and Time */ import java.util.*; class JavaDateTime { public static void main(String args[]) { try { long start_time = System.currentTimeMillis(); System.out.println(new Date() + "\n"); Thread.sleep(5*60*10); System.out.println(new Date() + "\n"); long end_time = System.currentTimeMillis(); long elapsed_time = end_time - start_time; System.out.println("Difference is : " + elapsed_time); } catch (Exception e) { System.out.println("Got an exception!"); } } }
This is the output produced when you run the above Java program:
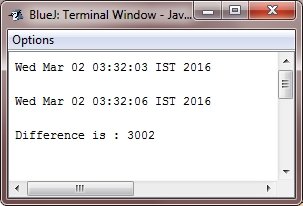
GregorianCalendar Class in Java
Here is another program uses GregorianCalendar class in Java:
/* Java Program Example - Java Date and Time */ import java.util.*; class JavaDateTime { public static void main(String args[]) { String months[] = { "Jan", "Feb", "Mar", "Apr", "May", "Jun", "Jul", "Aug", "Sep", "Oct", "Nov", "Dec" }; int year; GregorianCalendar gcalendar = new GregorianCalendar(); System.out.print("Date : "); System.out.print(months[gcalendar.get(Calendar.MONTH)]); System.out.print(" " + gcalendar.get(Calendar.DATE) + " "); System.out.println(year = gcalendar.get(Calendar.YEAR)); System.out.print("Time : "); System.out.print(gcalendar.get(Calendar.HOUR) + ":"); System.out.print(gcalendar.get(Calendar.MINUTE) + ":"); System.out.println(gcalendar.get(Calendar.SECOND)); if(gcalendar.isLeapYear(year)) { System.out.println("This year is a Leap Year."); } else { System.out.println("This year is not a Leap Year."); } } }
Following is the output produced by the above Java program:
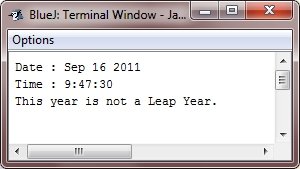
Here is another program that you may like, Print Time and Date in Java.
« Previous Tutorial Next Tutorial »