- Java Programming Basics
- Java Tutorial
- Java Overview
- Java Environment Setup
- Java Program Structure
- Java Basic Syntax
- Java First Program
- Java Constants
- Java Separators
- Java Keywords
- Java Data Types
- Java Data Types
- Java Integers
- Java Floating Point
- Java Characters
- Java Booleans
- Java Numbers
- Java Programming Variables
- Java Variables
- Java Variable Types
- Java Variable Scope
- Java Type Conversion
- Java Type Casting
- Java Auto Type Promotion
- Java Type Promotion Rules
- Java Programming Arrays
- Java Arrays
- Java One Dimensional Array
- Java Multidimensional Array
- Java Programming Operators
- Java Operators
- Java Arithmetic Operators
- Java Increment Decrement
- Java Bitwise Operators
- Java Left Shift
- Java Right Shift
- Java Relational Operators
- Java Boolean Logical Operators
- Java Ternary(?) Operator
- Java Operator Precedence
- Java Control Statements
- Java Decision Making
- Java if if-else if-else-if
- Java switch Statement
- Java Loops
- Java while Loop
- Java do-while Loop
- Java for Loop
- Java for-each Loop
- Java Nested Loops
- Java break Statement
- Java continue Statement
- Java Class Object Method
- Java Classes and Objects
- Java Class
- Java Object
- Java new Operator
- Java Methods
- Java Constructors
- Java this Keyword
- Java Stack
- Java Overloading Recursion
- Java Method Overloading
- Java Constructor Overloading
- Java Object as Parameter
- Java Call by Value Reference
- Java Returning Objects
- Java Recursion
- Java Modifier Types
- Java Encapsulate Poly String
- Java Encapsulation
- Java Polymorphism
- Java Nested Inner Class
- Java Strings
- Java Command Line Arguments
- Java Variable Length Arguments
- Java Inheritance Abstraction
- Java Inheritance
- Java super Superclass
- Java Multilevel Hierarchy
- Java Method Overriding
- Java Abstraction
- Java Packages Interfaces
- Java Packages
- Java Access Protection
- Java Import Statement
- Java Interfaces
- Java Programming Exceptions
- Java Exception Handling
- Java try catch
- Java throw throws
- Java finally Block
- Java Built In Exceptions
- Java Exception Subclasses
- Java Chained Exceptions
- Java Multithreading
- Java Multithreading
- Java Thread Model
- Java Main Thread
- Java Create Thread
- Java Thread Priorities
- Java Synchronization
- Java Inter Thread Communication
- Java Suspend Resume Stop Thread
- Java Get Thread State
- Java Enum Autobox Annotation
- Java Enumerations
- Java Type Wrappers
- Java Autoboxing
- Java Annotation
- Java Marker Annotations
- Java Single Member Annotation
- Java Built In Annotations
- Java Type Annotations
- Java Repeating Annotations
- Java Data File Handling
- Java Files I/O
- Java Streams
- Java Read Console Input
- Java Write Console Output
- Java PrintWriter Class
- Java Read Write Files
- Java Automatically Close File
- Java Programming Advance
- Java Date and Time
- Java Regular Expressions
- Java Collections Framework
- Java Generics
- Java Data Structures
- Java Network Programming
- Java Serialization
- Java Send Email
- Java Applet Basics
- Java Documentation
- Java Programming Examples
- Java Programming Examples
Java Autoboxing and Unboxing
Beginning with the JDK 5, Java added the following two important features:
- autoboxing
- auto-unboxing
Java Autoboxing
Autoboxing is a process through which a primitive type is automatically encapsulated (boxed) into its equivalent type wrapper whenever an object of that type is required. There is no need to explicitly construct an object.
Java Auto-unboxing
Auto-unboxing is a process through which the value of a boxed object is automatically extracted (unboxed) from type wrapper when its value is required. There is no need to call a method such as doubleValue() or intValue().
The addition of the autoboxing and auto-unboxing greatly streamlines the coding of several algorithms, removing the tedium of manually boxing and unboxing the values. It also helps to prevent errors. Moreover, it is very important to generics, which operate only on objects. Now, autoboxing makes working with the Collections Framework
With the autoboxing, it is no longer necessary to manually construct an object in order to wrap a primitive type. You require only to assign that value to a type-wrapper reference. Java automatically constructs the object for you. For example, here is a modern way to construct an Integer object that has the value 10:
Integer iObj = 10; // autobox an int
Notice here that the object is not explicitly created through the use of the new keyword. Java handles this for you, automatically.
How to Unbox an Object ?
To unbox an object, just assign that object reference to a primitive type variable. For example, to unbox the iObj, you can use the following statement:
int i = iObj; // auto-unbox
Java handles the details for you.
Java Autoboxing Unboxing Example
Following is the preceding program (rewritten) to use autoboxing/unboxing:
/* Java Program Example - Java Autoboxing * This program demonstrate autoboxing/unboxing */ class AutoBoxingDemo { public static void main(String args[]) { Integer iObj = 10; // autobox an int int i = iObj; // auto-unbox System.out.println(i + " " + iObj); // displays 10 10 } }
Above Java program will produce this output:
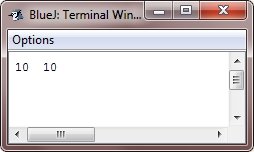
Java Autoboxing and Methods
In addition to simple case of assignments, autoboxing automatically occurs whenever a primitive type must be converted into an object, auto-unboxing takes place when an object must be converted into a primitive type. Thus, autoboxing/unboxing may occur when an assignment is passed to a method, or when a value is returned by a method. For example, consider the following example program:
/* Java Program Example - Java Autoboxing/Unboxing * Autoboxing/unboxing takes place with * method parameters and return values */ class AutoBoxingDemo { /* takes an integer parameter and return * an int value */ static int meth(Integer val) { return val; // auto-unbox to int } public static void main(String args[]) { /* pass an int to the method named meth() * and assign the return value to an Integer. * Here, the * argument 10 is autoboxed into an Integer. * The return value is also autoboxed into * an Integer */ Integer iObj = meth(10); System.out.println(iObj); } }
Above Java program will display the following output:
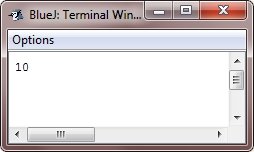
In this program, notice that, meth() specifies an Integer parameter and returns an int result. Inside the main() method, the method meth() is passed the value 10. Because meth() is expecting an Integer, this value is automatically boxed. Then, the method named meth() returns the int equivalent of its argument. This causes val to be auto-unboxed. Next, this int value is assigned to iObj in the main() method, which causes the int return value to be autoboxed.
« Previous Tutorial Next Tutorial »