- Java Programming Basics
- Java Tutorial
- Java Overview
- Java Environment Setup
- Java Program Structure
- Java Basic Syntax
- Java First Program
- Java Constants
- Java Separators
- Java Keywords
- Java Data Types
- Java Data Types
- Java Integers
- Java Floating Point
- Java Characters
- Java Booleans
- Java Numbers
- Java Programming Variables
- Java Variables
- Java Variable Types
- Java Variable Scope
- Java Type Conversion
- Java Type Casting
- Java Auto Type Promotion
- Java Type Promotion Rules
- Java Programming Arrays
- Java Arrays
- Java One Dimensional Array
- Java Multidimensional Array
- Java Programming Operators
- Java Operators
- Java Arithmetic Operators
- Java Increment Decrement
- Java Bitwise Operators
- Java Left Shift
- Java Right Shift
- Java Relational Operators
- Java Boolean Logical Operators
- Java Ternary(?) Operator
- Java Operator Precedence
- Java Control Statements
- Java Decision Making
- Java if if-else if-else-if
- Java switch Statement
- Java Loops
- Java while Loop
- Java do-while Loop
- Java for Loop
- Java for-each Loop
- Java Nested Loops
- Java break Statement
- Java continue Statement
- Java Class Object Method
- Java Classes and Objects
- Java Class
- Java Object
- Java new Operator
- Java Methods
- Java Constructors
- Java this Keyword
- Java Stack
- Java Overloading Recursion
- Java Method Overloading
- Java Constructor Overloading
- Java Object as Parameter
- Java Call by Value Reference
- Java Returning Objects
- Java Recursion
- Java Modifier Types
- Java Encapsulate Poly String
- Java Encapsulation
- Java Polymorphism
- Java Nested Inner Class
- Java Strings
- Java Command Line Arguments
- Java Variable Length Arguments
- Java Inheritance Abstraction
- Java Inheritance
- Java super Superclass
- Java Multilevel Hierarchy
- Java Method Overriding
- Java Abstraction
- Java Packages Interfaces
- Java Packages
- Java Access Protection
- Java Import Statement
- Java Interfaces
- Java Programming Exceptions
- Java Exception Handling
- Java try catch
- Java throw throws
- Java finally Block
- Java Built In Exceptions
- Java Exception Subclasses
- Java Chained Exceptions
- Java Multithreading
- Java Multithreading
- Java Thread Model
- Java Main Thread
- Java Create Thread
- Java Thread Priorities
- Java Synchronization
- Java Inter Thread Communication
- Java Suspend Resume Stop Thread
- Java Get Thread State
- Java Enum Autobox Annotation
- Java Enumerations
- Java Type Wrappers
- Java Autoboxing
- Java Annotation
- Java Marker Annotations
- Java Single Member Annotation
- Java Built In Annotations
- Java Type Annotations
- Java Repeating Annotations
- Java Data File Handling
- Java Files I/O
- Java Streams
- Java Read Console Input
- Java Write Console Output
- Java PrintWriter Class
- Java Read Write Files
- Java Automatically Close File
- Java Programming Advance
- Java Date and Time
- Java Regular Expressions
- Java Collections Framework
- Java Generics
- Java Data Structures
- Java Network Programming
- Java Serialization
- Java Send Email
- Java Applet Basics
- Java Documentation
- Java Programming Examples
- Java Programming Examples
Java Strings
The String is probably the most commonly used class in Java's class library. The reason for this is that strings are a very important part of programming.
The first thing to understand about strings is that every string you create is actually an object of the type String. Even string constants are actually the String objects. For example, in the following statement
System.out.println("This is a String");
the string "This is a String" is a String object.
The second thing to understand about strings is that objects of the type String are immutable, once a String object is created, its contents cannot be altered. While this may seem like a serious restriction, it is not, for the following two reasons :
- If you need to change a string, you can always create a new one that contains the modifications.
- Java defines peer classes of String, called the StringBuffer and the StringBuilder, which allow strings to be altered, so all of the normal string manipulations are string available in Java.
Create Strings in Java
In Java, String can be constructed in a variety of ways. The easiest way is to use a statement like this :
String myStr = "this is a string";
Once you have created a String object, you can use it anywhere that a string is allowed. For example, the following statement displays myStr :
System.out.println(myStr);
Java defines one operator for the String objects: +. It is used to concatenate two strings. For example, the following statement
String myStr = "I" + " like " + "Java";
results in myStr containing "I like Java"
Java Strings Example
The following Java program demonstrates the preceding concepts of Strings :
/* Java Program Example - Java Strings Class * This program demonstrates the Strings */ class JavaProgram { public static void main(String args[]) { String strObj1 = "First String"; String strObj2 = "Second String"; String strObj3 = strObj1 + " and " + strObj2; /* now print the values of all the String objects */ System.out.println(strObj1); System.out.println(strObj2); System.out.println(strObj3); } }
When the above Java program is compile and executed, it will produce the following output:
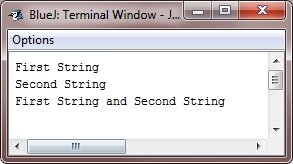
« Previous Tutorial Next Tutorial »