- JavaScript Basics
- JS Home
- JS Syntax
- JS Placements
- JS Output
- JS Statements
- JS Keywords
- JS Comments
- JS Variables
- JS var
- JS let
- JS const
- JS var Vs let Vs const
- JS Operators
- JS Arithmetic Operators
- JS Assignment Operators
- JS Comparison Operators
- JS Logical Operators
- JS Bitwise Operators
- JS Ternary Operator
- JS Operator Precedence
- JS Data Types
- JS typeof
- JS Conditional Statements
- JS Conditional Statement
- JS if Statement
- JS if...else Statement
- JS switch Statement
- JS Loops
- JS for Loop
- JS while Loop
- JS do...while Loop
- JS break Statement
- JS continue Statement
- JS break Vs. continue
- JavaScript Popup Boxes
- JS Dialog Box
- JS alert Box
- JS confirm Box
- JS prompt Box
- JavaScript Functions
- JS Functions
- JS setTimeout() Method
- JS setInterval() Method
- JavaScript Events
- JS Events
- JS onclick Event
- JS onload Event
- JS Mouse Events
- JS onreset Event
- JS onsubmit Event
- JavaScript Arrays
- JS Array
- JS Find Length of Array
- JS Add Elements at Beginning
- JS Add Element at End
- JS Remove First Element
- JS Remove Last Element
- JS Get First Index
- JS Get Last Index
- JS Reverse an Array
- JS Sort an Array
- JS Concatenate Arrays
- JS join()
- JS toString()
- JS from()
- JS Check if Value Exists
- JS Check if Array
- JS Slice an Array
- JS splice()
- JS find()
- JS findIndex()
- JS entries()
- JS every()
- JS fill()
- JS filter()
- JS forEach()
- JS map()
- JavaScript Strings
- JS String
- JS Length of String
- JS Convert to Lowercase
- JS Convert to Uppercase
- JS String Concatenation
- JS search()
- JS indexOf()
- JS search() Vs. indexOf()
- JS match()
- JS match() Vs. search()
- JS replace()
- JS toString()
- JS String()
- JS includes()
- JS substr()
- JS Slice String
- JS charAt()
- JS repeat()
- JS split()
- JS charCodeAt()
- JS fromCharCode()
- JS startsWith()
- JS endsWith()
- JS trim()
- JS lastIndexOf()
- JavaScript Objects
- JS Objects
- JS Boolean Object
- JavaScript Math/Number
- JS Math Object
- JS Math.abs()
- JS Math.max()
- JS Math.min()
- JS Math.pow()
- JS Math.sqrt()
- JS Math.cbrt()
- JS Math.round()
- JS Math.ceil()
- JS Math.floor()
- JS Math.trunc
- JS toFixed()
- JS toPrecision()
- JS Math.random()
- JS Math.sign()
- JS Number.isInteger()
- JS NaN
- JS Number()
- JS parseInt()
- JS parseFloat()
- JavaScript Date and Time
- JS Date and Time
- JS Date()
- JS getFullYear()
- JS getMonth()
- JS getDate()
- JS getDay()
- JS getHours()
- JS getMinutes()
- JS getSeconds()
- JS getMilliseconds()
- JS getTime()
- JS getUTCFullYear()
- JS getUTCMonth()
- JS getUTCDate()
- JS getUTCDay()
- JS getUTCHours()
- JS getUTCMinutes()
- JS getUTCSeconds()
- JS getUTCMilliseconds()
- JS toDateString()
- JS toLocaleDateString()
- JS toLocaleTimeString()
- JS toLocaleString()
- JS toUTCString()
- JS getTimezoneOffset()
- JS toISOString()
- JavaScript Browser Objects
- JS Browser Objects
- JS Window Object
- JS Navigator Object
- JS History Object
- JS Screen Object
- JS Location Object
- JavaScript Document Object
- JS Document Object Collection
- JS Document Object Properties
- JS Document Object Methods
- JS Document Object with Forms
- JavaScript DOM
- JS DOM
- JS DOM Nodes
- JS DOM Levels
- JS DOM Interfaces
- JavaScript Cookies
- JS Cookies
- JS Create/Delete Cookies
- JavaScript Regular Expression
- JS Regular Expression
- JS RegEx .
- JS RegEx \w and \W
- JS RegEx \d and \D
- JS RegEx \s and \S
- JS RegEx \b and \B
- JS RegEx \0
- JS RegEx \n
- JS RegEx \xxx
- JS RegEx \xdd
- JS RegEx Quantifiers
- JS RegEx test()
- JS RegEx lastIndex
- JS RegEx source
- JavaScript Advance
- JS Page Redirection
- JS Form Validation
- JS Validations
- JS Error Handling
- JS Exception Handling
- JS try-catch throw finally
- JS onerror Event
- JS Multimedia
- JS Animation
- JS Image Map
- JS Debugging
- JS Browser Detection
- JS Security
- JavaScript Misc
- JS innerHTML
- JS getElementById()
- JS getElementsByClassName()
- JS getElementsByName()
- JS getElementsByTagName()
- JS querySelector()
- JS querySelectorAll()
- JS document.write()
- JS console.log()
- JS instanceof
- JavaScript Programs
- JavaScript Programs
JavaScript Form Validation
As you know that you can validate any HTML form just by using the onsubmit attribute of the FORM element.
The onsubmit invokes a function, which is create by using JavaScript to check whether the field of the form is valid or not valid.
JavaScript Required Fields Validation
Using JavaScript, you are free to restrict a user to fill up the form fields. Here is an example shows how to validate required fields using JavaScript:
<!DOCTYPE HTML> <HEAD> <TITLE>JavaScript Required Fields Validation</TITLE> <SCRIPT type="text/javascript"> function validateRequiredFields(this_form) { var username=this_form.usrname.value; var password=this_form.usrpassword.value; if(username==null || username=="") { alert("Please enter your username"); this_form.usrname.focus(); return false; } if(password==null || password=="") { alert("Please enter your password"); this_form.usrpassword.focus(); return false; } return true; } </SCRIPT> </HEAD> <BODY> <FORM action="#" onsubmit="return validateRequiredFields(this)" method="Post"> Username: <input type= "text" name="usrname"/><span style="color:red;">*</span><br/> Password: <input type= "password" name="usrpassword"/><span style="color:red;">*</span><br/> <INPUT type="submit" value="Login"/> </FORM> </BODY> </HTML>
Here is the sample output produced by the above JavaScript required field form validation example program. This is the initial output:
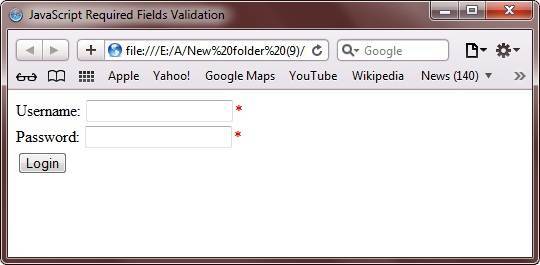
Without entering the username and the password, just click on the Login button, then you will watch the following output:
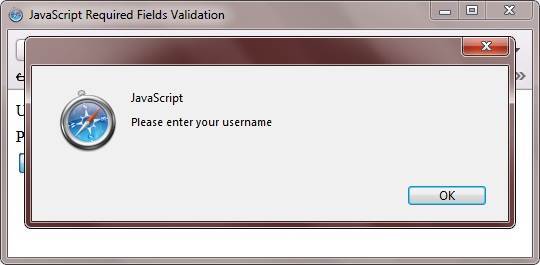
Now enter your username as shown in the following snapshot:
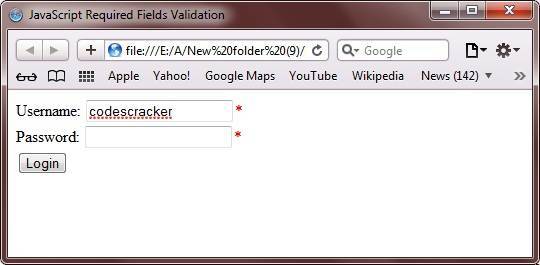
After entering the username, leave the password field and click on the Login button, then you will watch this output:
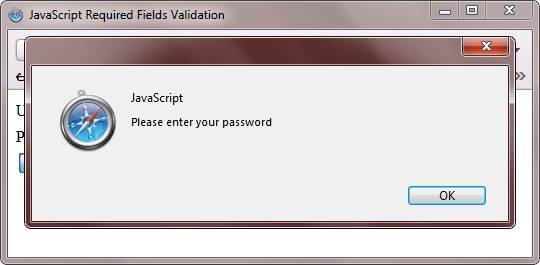
Here is the live demo output of the above JavaScript required field form validation example program.
JavaScript Numbers Validation
You can use a JavaScript function named isNaN to validate a number object. Here is an example shows how to validate numbers using JavaScript:
<!DOCTYPE HTML> <HTML> <HEAD> <TITLE>JavaScript Number Validation</TITLE> <SCRIPT type="text/javascript"> function validateNumber(this_form2) { var num2 = this_form2.num2.value; if(num2==null|| num2=="") { alert("Please enter a number."); this_form2.num2.focus(); return false; } if(isNaN(num2)) { alert("It is not a valid number."); this_form2.num2.focus(); return false; } return true; } </SCRIPT> </HEAD> <BODY> <FORM action="#" onsubmit="return validateNumber(this)" method="Post"> Enter a Number: <INPUT type="text" name="num2"/><BR/> <INPUT type="submit" value="Validate"/> </FORM> </BODY> </HTML>
Here are some sample outputs of the above JavaScript number validation example program. This is the initial output:
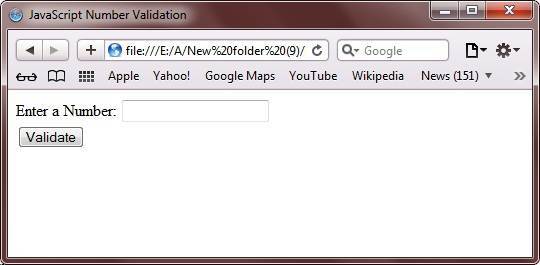
Now here is the output produced when you click on the Validate button without entering any number:
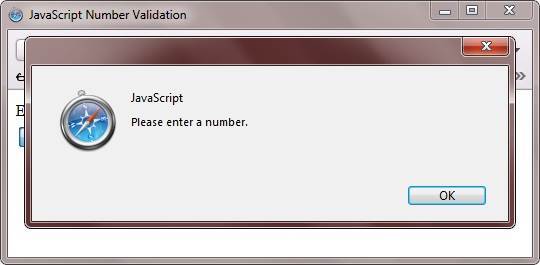
And here is the output produced when you enter a character except of number and click on the Validate button:
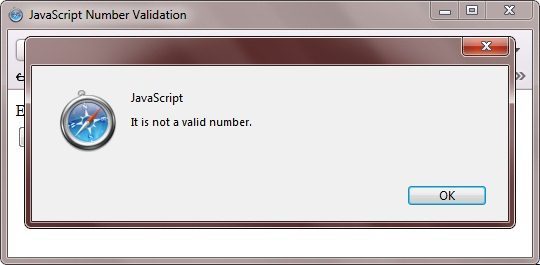
Here is the live demo output of the above number validation example using JavaScript:
JavaScript Username and Password Validation
You can also validate username and password field using JavaScript such as to check the values of the fields for null and blank or to restrict the length of the values and many more. Here is an example shows how to validate username and password field of the form using JavaScript:
<!DOCTYPE HTML> <HTML> <HEAD> <TITLE>JavaScript Username and Password Validation</TITLE> <SCRIPT type="text/javascript"> function validateUsernamePassword(this_form3) { var userName = this_form3.user_name.value; var pass1 = this_form3.password1.value; var pass2 = this_form3.password2.value; if(userName=="") { alert("Please enter your username."); this_form3.user_name.focus(); return false; } if(userName.length<6) { alert("Username must be at least of 6 characters."); this_form3.user_name.focus(); return false; } if(pass1=="") { alert("Please enter your password."); this_form3.password1.focus(); return false; } if(pass1.length<8) { alert("Password must contain at least of 8 digits"); this_form3.password1.focus(); return false; } if(pass2=="") { alert("Please re-enter your password for confirmation."); this_form3.password2.focus(); return false; } if(pass1.length>=8 && pass2.length>=8 ) { if(pass1!=pass2) { alert("Sorry! Password mismatched! Please try again."); return false; } } return true; } </SCRIPT> </HEAD> <BODY> <FORM action="#" onsubmit="return validateUsernamePassword(this)" method="Post"> Enter Username: <INPUT type="text" name="user_name"/><BR/> Enter Password: <INPUT type="password" name="password1"/><BR/> Re-enter Password: <INPUT type="password" name="password2"/><BR/> <INPUT type="submit" value="Login"/> </FORM> </BODY> </HTML>
Here are some sample outputs produced by the above JavaScript username and password field validation example program. This is the initial output:
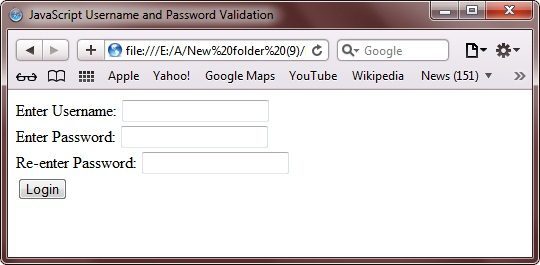
Now click on the Login button without entering anything. Then you will watch the following output:
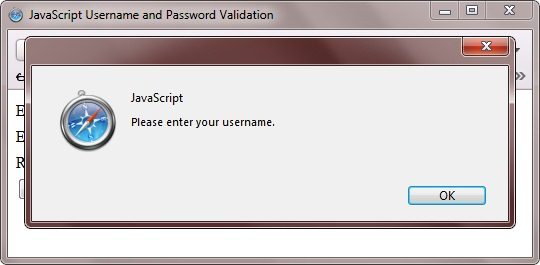
Now enter the username (contains only of 5 characters) and click on the Login button. Then you will watch the following output:
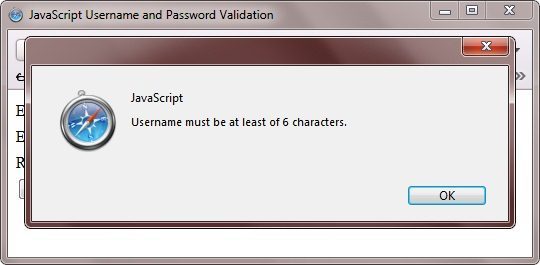
Now enter fresherearth as username and codes as password, press on the Login button. Then here is the output you will watch:
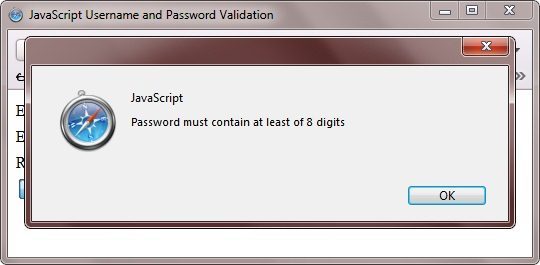
Now enter fresherearth as username, 123456789 as password, 123456780 as re-enter password, and click on the Login button. Then here is the output produced by the above username and password validation program using JavaScript:
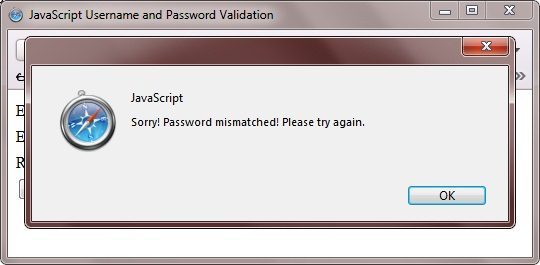
Here is the live demo output produced by the above JavaScript username and password example program.
JavaScript Phone Number Validation
You can also validate the Phone number entered by the user using JavaScript. To validate phone number, you have to check the following three conditions:
- user must enter a value
- value must be an integer value
- value must have at least of 10 digits
Here is an example shows how to validate phone number entered by the user using JavaScript:
<!DOCTYPE HTML> <HTML> <HEAD> <TITLE>JavaScript Phone Number Validation</TITLE> <SCRIPT type="text/javascript"> function validatePhoneNumber(this_form4) { var phon=this_form4.phonnumbe.value; if(phon==null||phon=="") { alert("Please enter your phone number."); this_form4.phonnumbe.focus(); return false; } if(phon.length<10) { alert("Phone number must be at least of 10 digits."); this_form4.phonnumbe.focus(); return false; } if(isNaN(phon)) { alert("Sorry! You have entered an invalid phone number! Please try again."); this_form4.phonnumbe.focus(); return false; } return true; } </SCRIPT> </HEAD> <BODY> <FORM action="#" onsubmit="return validatePhoneNumber(this)" method="Post"> Phone Number: <input type="text" name="phonnumbe"/><BR/> <INPUT type="submit" value="Validate"/> </FORM> </BODY> </HTML>
Here is the sample outputs produced by the above JavaScript phone number validation example program. This is the initial output:
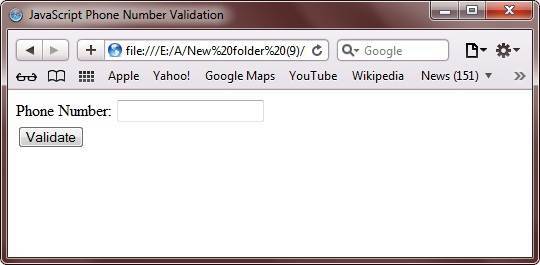
Now press on the Validate button without entering anything. Here is the output you will watch:
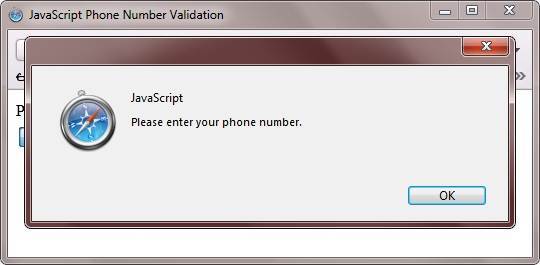
Now enter fresherearth as phone number and click on the Validate button, you will watch the following output:
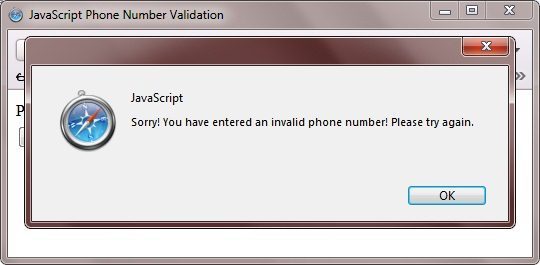
Here is the live demo output produced by the above phone number validation example program using JavaScript:
JavaScript Time Validation
You can also validate time using JavaScript. In other word, you can check whether the entered time is in correct format or not using JavaScript. Here is an example shows how to validate time using JavaScript.
<!DOCTYPE HTML> <HTML> <HEAD> <TITLE>JavaScript Time Validation</TITLE> <SCRIPT type="text/javascript"> function validateTime(this_form5) { var timeVa = this_form5.timeval.value; if(timeVa == "") { alert("Please enter the Time."); this_form5.timeval.focus(); return false; } var positio = timeVa.indexOf(":"); if(positio<0) { alert("Sorry! You have entered an invalid Time! The colon ( : ) is missing between HH and MM."); this_form5.timeval.focus(); return false; } if(positio>2 || positio<1) { alert("Sorry! You have entered an invalid Time! The Time format should be HH:MM AM/PM."); this_form5.timeval.focus(); return false; } var hours = timeVa.substring(0, positio); if(hours>12) { alert("Sorry! You have entered an invalid Time! Hours cannot be greater than 12."); this_form5.timeval.focus(); return false; } if(hours<=0) { alert("Sorry! You have entered an invalid Time! Hours cannot be smallar than 0."); this_form5.timeval.focus(); return false; } var min = timeVa.substring(positio+1, positio+3); if(min>59) { alert("Sorry! You have entered an invalid Time! Mins cannot be greater than 59."); this_form5.timeval.focus(); return false; } if(min<0) { alert("Sorry! You have entered an invalid Time! Mins cannot be smallar than 0."); this_form5.timeval.focus(); return false; } var t1 = timeVa.substring(5,8); if(t1!= "AM" && t1!= "am" && t1!="PM" && t1!= "pm") { alert("Sorry! You have entered an invalid Time! Time should be end with AM or PM."); this_form5.timeval.focus(); return false; } return true; } </SCRIPT> </HEAD> <BODY> <FORM action="#" onsubmit="return validateTime(this)" method="Post"> Enter Time (HH:MM AM/PM): <input type="text" name="timeval"/><br/> <INPUT type="submit" value="Validate"/> </FORM> </BODY> </HTML>
Here are some sample outputs produced by the above JavaScript time validation example program. This is the initial output:
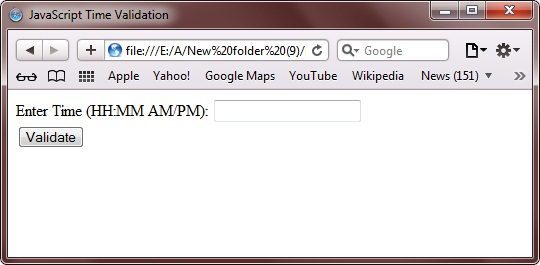
Now enter 10 12AM and click on the Validate button. You will watch the following output:
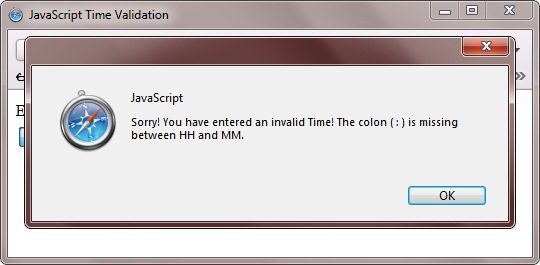
Here is the live demo output produced by the above time validation program using JavaScript.
JavaScript Date Validation
You can also validate date entered by the user using JavaScript. You can validate date depends on the format such as mm/dd/yyyy format. In this format you have to validate according to the mm/dd/yyyy format that means the month and date should have exactly of 2 digits and the year should have four digits and at last all the three (day, month, and year) must be separated by the / symbol.
Here is an example demonstrates how to validate date entered by the user using JavaScript:
<!DOCTYPE HTML> <HTML> <HEAD> <TITLE>JavaScript Date Validation</TITLE> <SCRIPT type="text/javascript"> function isDate(dateTxtVal) { var objecDate; var mSeconds; if (dateTxtVal=="") { alert("Please enter the Date."); return false; } var dayVal = dateTxtVal.substring(3,5); var monthVal = dateTxtVal.substring(0,2)-1; var yearVal = dateTxtVal.substring(6,10); if (dateTxtVal.substring(2,3) != '/') { alert("Sorry! You entered the Date in wrong format! Please enter the date in correct format."); return false; } if (dateTxtVal.substring(5,6) != '/') { alert("Sorry! You entered the Date in wrong format! Please enter the date in correct format."); return false; } mSeconds = (new Date(yearVal, monthVal, dayVal)).getTime(); objecDate = new Date(); objecDate.setTime(mSeconds); if (objecDate.getDate()!= dayVal) { alert("Sorry! You have entered the wrong Day! Please try again."); return false; } if (objecDate.getMonth()!= monthVal) { alert("Sorry! You have entered the wrong month! Please try again."); return false; } if (objecDate.getFullYear()!= yearVal) { alert("Sorry! You have entered the wrong year! Please try again."); return false; } return true; } function validateDate(thisform) { var dateTxtVal = thisform.dateVa.value; if (isDate(dateTxtVal)) return true; else return false; } </SCRIPT> </HEAD> <BODY> <FORM action="#" onsubmit="return validateDate(this)" method="Post"> Enter Date (mm/dd/yyyy): <INPUT type="text" name="dateVa"/><BR/> <INPUT type="submit" value="Validate"/> </FORM> </BODY> </HTML>
Here are some sample outputs produced by the above JavaScript date validation example program. This is the initial output:
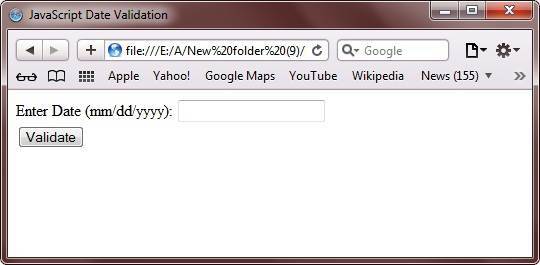
Now enter 10:05:2015 and click on the Validate button. Then you will watch the following output:
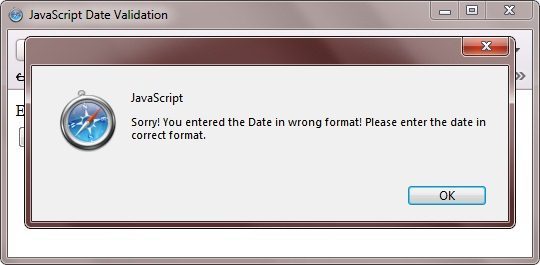
Here is the live demo output produced by the above date validation example using JavaScript.
JavaScript Form Validation More Examples
Here are some simple example program, shows how to validate form using JavaScript:
<!DOCTYPE html> <html> <head> <title>JavaScript Form Validation Example</title> <script> function validateFormFun() { var x = document.forms["formNumberOne"]["firstName"].value; if (x == null || x == "") { alert("Please enter your name first."); return false; } } </script> </head> <body> <form name="formNumberOne" action="#" onsubmit="return validateFormFun()" method="post"> Name: <input type="text" name="firstName"> <input type="submit" value="Validate"> </form> </body> </html>
Here is the live demo output produced by the form validation example using JavaScript:
Here is another example, demonstrates JavaScript form validation. In this example, if an input field contains invalid data, displays an error message:
<!DOCTYPE html> <html> <head> <title>JavaScript Form Validation Example</title> <script> function validateFormFun2() { var inputObject = document.getElementById("inputId2"); if (inputObject.checkValidity() == false) { document.getElementById("paragraph2").innerHTML = inputObject.validationMessage; } else { document.getElementById("paragraph2").innerHTML = "It is OK"; } } </script> </head> <body> <p>Enter your marks out of 100.</p> <input id="inputId2" type="number" min="0" max="100"> <button onclick="validateFormFun2()">Submit</button> <p>If entered mark is less than 0 or greater than 100 then an error message will be displayed below.</p> <p id="paragraph2"></p> </body> </html>
Here is the live demo output produced by the above form validation example using JavaScript:
Enter your marks out of 100.
If entered mark is less than 0 or greater than 100 then an error message will be displayed below.
« Previous Tutorial Next Tutorial »