- JavaScript Basics
- JS Home
- JS Syntax
- JS Placements
- JS Output
- JS Statements
- JS Keywords
- JS Comments
- JS Variables
- JS var
- JS let
- JS const
- JS var Vs let Vs const
- JS Operators
- JS Arithmetic Operators
- JS Assignment Operators
- JS Comparison Operators
- JS Logical Operators
- JS Bitwise Operators
- JS Ternary Operator
- JS Operator Precedence
- JS Data Types
- JS typeof
- JS Conditional Statements
- JS Conditional Statement
- JS if Statement
- JS if...else Statement
- JS switch Statement
- JS Loops
- JS for Loop
- JS while Loop
- JS do...while Loop
- JS break Statement
- JS continue Statement
- JS break Vs. continue
- JavaScript Popup Boxes
- JS Dialog Box
- JS alert Box
- JS confirm Box
- JS prompt Box
- JavaScript Functions
- JS Functions
- JS setTimeout() Method
- JS setInterval() Method
- JavaScript Events
- JS Events
- JS onclick Event
- JS onload Event
- JS Mouse Events
- JS onreset Event
- JS onsubmit Event
- JavaScript Arrays
- JS Array
- JS Find Length of Array
- JS Add Elements at Beginning
- JS Add Element at End
- JS Remove First Element
- JS Remove Last Element
- JS Get First Index
- JS Get Last Index
- JS Reverse an Array
- JS Sort an Array
- JS Concatenate Arrays
- JS join()
- JS toString()
- JS from()
- JS Check if Value Exists
- JS Check if Array
- JS Slice an Array
- JS splice()
- JS find()
- JS findIndex()
- JS entries()
- JS every()
- JS fill()
- JS filter()
- JS forEach()
- JS map()
- JavaScript Strings
- JS String
- JS Length of String
- JS Convert to Lowercase
- JS Convert to Uppercase
- JS String Concatenation
- JS search()
- JS indexOf()
- JS search() Vs. indexOf()
- JS match()
- JS match() Vs. search()
- JS replace()
- JS toString()
- JS String()
- JS includes()
- JS substr()
- JS Slice String
- JS charAt()
- JS repeat()
- JS split()
- JS charCodeAt()
- JS fromCharCode()
- JS startsWith()
- JS endsWith()
- JS trim()
- JS lastIndexOf()
- JavaScript Objects
- JS Objects
- JS Boolean Object
- JavaScript Math/Number
- JS Math Object
- JS Math.abs()
- JS Math.max()
- JS Math.min()
- JS Math.pow()
- JS Math.sqrt()
- JS Math.cbrt()
- JS Math.round()
- JS Math.ceil()
- JS Math.floor()
- JS Math.trunc
- JS toFixed()
- JS toPrecision()
- JS Math.random()
- JS Math.sign()
- JS Number.isInteger()
- JS NaN
- JS Number()
- JS parseInt()
- JS parseFloat()
- JavaScript Date and Time
- JS Date and Time
- JS Date()
- JS getFullYear()
- JS getMonth()
- JS getDate()
- JS getDay()
- JS getHours()
- JS getMinutes()
- JS getSeconds()
- JS getMilliseconds()
- JS getTime()
- JS getUTCFullYear()
- JS getUTCMonth()
- JS getUTCDate()
- JS getUTCDay()
- JS getUTCHours()
- JS getUTCMinutes()
- JS getUTCSeconds()
- JS getUTCMilliseconds()
- JS toDateString()
- JS toLocaleDateString()
- JS toLocaleTimeString()
- JS toLocaleString()
- JS toUTCString()
- JS getTimezoneOffset()
- JS toISOString()
- JavaScript Browser Objects
- JS Browser Objects
- JS Window Object
- JS Navigator Object
- JS History Object
- JS Screen Object
- JS Location Object
- JavaScript Document Object
- JS Document Object Collection
- JS Document Object Properties
- JS Document Object Methods
- JS Document Object with Forms
- JavaScript DOM
- JS DOM
- JS DOM Nodes
- JS DOM Levels
- JS DOM Interfaces
- JavaScript Cookies
- JS Cookies
- JS Create/Delete Cookies
- JavaScript Regular Expression
- JS Regular Expression
- JS RegEx .
- JS RegEx \w and \W
- JS RegEx \d and \D
- JS RegEx \s and \S
- JS RegEx \b and \B
- JS RegEx \0
- JS RegEx \n
- JS RegEx \xxx
- JS RegEx \xdd
- JS RegEx Quantifiers
- JS RegEx test()
- JS RegEx lastIndex
- JS RegEx source
- JavaScript Advance
- JS Page Redirection
- JS Form Validation
- JS Validations
- JS Error Handling
- JS Exception Handling
- JS try-catch throw finally
- JS onerror Event
- JS Multimedia
- JS Animation
- JS Image Map
- JS Debugging
- JS Browser Detection
- JS Security
- JavaScript Misc
- JS innerHTML
- JS getElementById()
- JS getElementsByClassName()
- JS getElementsByName()
- JS getElementsByTagName()
- JS querySelector()
- JS querySelectorAll()
- JS document.write()
- JS console.log()
- JS instanceof
- JavaScript Programs
- JavaScript Programs
JavaScript Cookies
Cookies lets you to store the user information in the web page. Cookies are basically the data, which are stored in a small text files, on the user's computer.
As cookies are simply a plain text data record of these 5 variable-length fields:
- domain - domain name of the website
- path - path to the directory or web page
- expires - date when the cookie will expire
- secure - if this field contains the word "secure", then the cookie only be retrieved with secure server, otherwise no such restrictions exists
- name=value - cookies are set and then retrieved in the form of key/value pairs
JavaScript Create Cookie
To create cookie using JavaScript, the simplest way is to assign a string value to the object, document.cookie. Here is the general form to create a cookie:
document.cookie = "key1=value1;key2=value2;expires=date";
Here, expires attribute is optional. Provide a valid date and time to make cookie expires at that date and time. Here is an example to create a cookie:
username=fresherearth
Here is another example, creates a cookie with JavaScript:
document.cookie="username=Deepak Patel";
Here is another example, creates cookie having expiration date:
document.cookie="username=Anoop Patel; expires=Tue, 16 Dec 2013 12:00:00 UTC";
Here is one more example, shows how to set path the cookie belongs to with a path parameter like this:
document.cookie="username=Shivam Patel; expires=Tue, 16 Dec 2013 12:00:00 UTC; path=/";
JavaScript Read Cookie
Here is an example, shows how to read cookie with JavaScript:
var x = document.cookie;
Here, document.cookie will return all the cookies in one string much like cookie1=value; cookie2=value; cookie3=value;
JavaScript Change Cookie
Here is an example, shows how to change a cookie with JavaScript:
document.cookie="username=Deepak Patel; expires=Tue, 16 Dec 2013 12:00:00 UTC; path=/";
Now after performing this, the old cookie is overwritten.
JavaScript Delete Cookie
To delete cookie with JavaScript, simply set the expires parameter to a passed date. Here is an example, shows how to delete a cookie with JavaScript:
document.cookie = "username=; expires=Thu, 01 Jan 1970 00:00:00 UTC";
JavaScript Cookie Example
Here is a complete example on JavaScript cookie:
<!DOCTYPE html> <html> <head> <title>JavaScript Cookies Example</title> <script> function createCookie(cookieName,cookieValue,cookieexpirydays) { var d = new Date(); d.setTime(d.getTime() + (cookieexpirydays*24*60*60*1000)); var expires = "expires=" + d.toGMTString(); document.cookie = cookieName+"="+cookieValue+"; "+expires; } function getCookie(cookieName) { var name = cookieName + "="; var ca = document.cookie.split(';'); for(var i=0; i<ca.length; i++) { var c = ca[i]; while (c.charAt(0)==' ') c = c.substring(1); if (c.indexOf(name) == 0) { return c.substring(name.length, c.length); } } return ""; } function checkCookie() { var user=getCookie("username"); if (user != "") { alert("Hi " + user + "! Welcome to fresherearth.com"); } else { user = prompt("Please enter your name: ",""); if (user != "" && user != null) { createCookie("username", user, 30); } } } </script> </head> <body> <input type="button" onclick="checkCookie()" value="Cookie"> </body> </html>
Here is the sample output of the above cookie example using JavaScript:
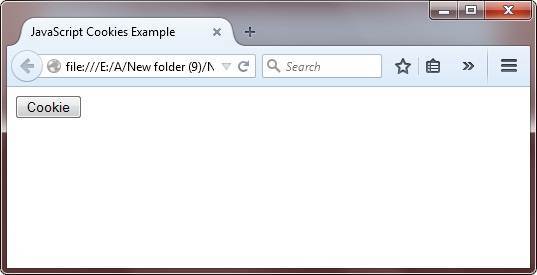
Now click on the Cookie button to create the cookie. Here is the output produced after clicking on the Cookie button on Mozilla Firefox browser:
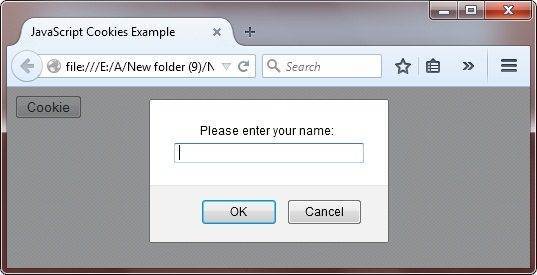
Now enter your name to create cookie and press on the OK button. Here we have entered fresherearth as name. After entering the name and clicking on the OK button, again click on the Cookie button, you will watch the following output:
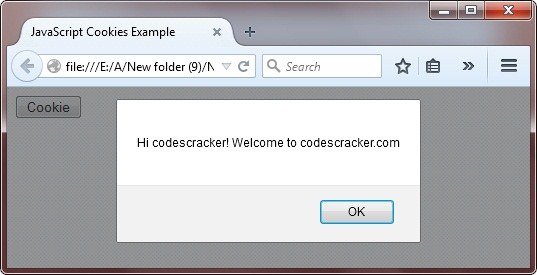
Here is the live demo output of the above JavaScript cookie program. Now, just click on the Cookie button and type your name to create a cookie. Now click again on the Cookie button to watch the greeting:
« Previous Tutorial Next Tutorial »