- JavaScript Basics
- JS Home
- JS Syntax
- JS Placements
- JS Output
- JS Statements
- JS Keywords
- JS Comments
- JS Variables
- JS var
- JS let
- JS const
- JS var Vs let Vs const
- JS Operators
- JS Arithmetic Operators
- JS Assignment Operators
- JS Comparison Operators
- JS Logical Operators
- JS Bitwise Operators
- JS Ternary Operator
- JS Operator Precedence
- JS Data Types
- JS typeof
- JS Conditional Statements
- JS Conditional Statement
- JS if Statement
- JS if...else Statement
- JS switch Statement
- JS Loops
- JS for Loop
- JS while Loop
- JS do...while Loop
- JS break Statement
- JS continue Statement
- JS break Vs. continue
- JavaScript Popup Boxes
- JS Dialog Box
- JS alert Box
- JS confirm Box
- JS prompt Box
- JavaScript Functions
- JS Functions
- JS setTimeout() Method
- JS setInterval() Method
- JavaScript Events
- JS Events
- JS onclick Event
- JS onload Event
- JS Mouse Events
- JS onreset Event
- JS onsubmit Event
- JavaScript Arrays
- JS Array
- JS Find Length of Array
- JS Add Elements at Beginning
- JS Add Element at End
- JS Remove First Element
- JS Remove Last Element
- JS Get First Index
- JS Get Last Index
- JS Reverse an Array
- JS Sort an Array
- JS Concatenate Arrays
- JS join()
- JS toString()
- JS from()
- JS Check if Value Exists
- JS Check if Array
- JS Slice an Array
- JS splice()
- JS find()
- JS findIndex()
- JS entries()
- JS every()
- JS fill()
- JS filter()
- JS forEach()
- JS map()
- JavaScript Strings
- JS String
- JS Length of String
- JS Convert to Lowercase
- JS Convert to Uppercase
- JS String Concatenation
- JS search()
- JS indexOf()
- JS search() Vs. indexOf()
- JS match()
- JS match() Vs. search()
- JS replace()
- JS toString()
- JS String()
- JS includes()
- JS substr()
- JS Slice String
- JS charAt()
- JS repeat()
- JS split()
- JS charCodeAt()
- JS fromCharCode()
- JS startsWith()
- JS endsWith()
- JS trim()
- JS lastIndexOf()
- JavaScript Objects
- JS Objects
- JS Boolean Object
- JavaScript Math/Number
- JS Math Object
- JS Math.abs()
- JS Math.max()
- JS Math.min()
- JS Math.pow()
- JS Math.sqrt()
- JS Math.cbrt()
- JS Math.round()
- JS Math.ceil()
- JS Math.floor()
- JS Math.trunc
- JS toFixed()
- JS toPrecision()
- JS Math.random()
- JS Math.sign()
- JS Number.isInteger()
- JS NaN
- JS Number()
- JS parseInt()
- JS parseFloat()
- JavaScript Date and Time
- JS Date and Time
- JS Date()
- JS getFullYear()
- JS getMonth()
- JS getDate()
- JS getDay()
- JS getHours()
- JS getMinutes()
- JS getSeconds()
- JS getMilliseconds()
- JS getTime()
- JS getUTCFullYear()
- JS getUTCMonth()
- JS getUTCDate()
- JS getUTCDay()
- JS getUTCHours()
- JS getUTCMinutes()
- JS getUTCSeconds()
- JS getUTCMilliseconds()
- JS toDateString()
- JS toLocaleDateString()
- JS toLocaleTimeString()
- JS toLocaleString()
- JS toUTCString()
- JS getTimezoneOffset()
- JS toISOString()
- JavaScript Browser Objects
- JS Browser Objects
- JS Window Object
- JS Navigator Object
- JS History Object
- JS Screen Object
- JS Location Object
- JavaScript Document Object
- JS Document Object Collection
- JS Document Object Properties
- JS Document Object Methods
- JS Document Object with Forms
- JavaScript DOM
- JS DOM
- JS DOM Nodes
- JS DOM Levels
- JS DOM Interfaces
- JavaScript Cookies
- JS Cookies
- JS Create/Delete Cookies
- JavaScript Regular Expression
- JS Regular Expression
- JS RegEx .
- JS RegEx \w and \W
- JS RegEx \d and \D
- JS RegEx \s and \S
- JS RegEx \b and \B
- JS RegEx \0
- JS RegEx \n
- JS RegEx \xxx
- JS RegEx \xdd
- JS RegEx Quantifiers
- JS RegEx test()
- JS RegEx lastIndex
- JS RegEx source
- JavaScript Advance
- JS Page Redirection
- JS Form Validation
- JS Validations
- JS Error Handling
- JS Exception Handling
- JS try-catch throw finally
- JS onerror Event
- JS Multimedia
- JS Animation
- JS Image Map
- JS Debugging
- JS Browser Detection
- JS Security
- JavaScript Misc
- JS innerHTML
- JS getElementById()
- JS getElementsByClassName()
- JS getElementsByName()
- JS getElementsByTagName()
- JS querySelector()
- JS querySelectorAll()
- JS document.write()
- JS console.log()
- JS instanceof
- JavaScript Programs
- JavaScript Programs
JavaScript Animation
Animation is a type of optical illusion where the rapid display of a sequence of images or frames of 2D or 3D artwork creates an illusion of movement. An animation movie or cartoon film is an example of animation.
Types of Animation
Animation can be divided into two categories given in the following table.
Animation Name | Description |
---|---|
Sprite Animation | Sprite animation defines a rectangular image in which the parts of the image are made transparent where you want to show the background. It has the ability to move an image over another image. |
Frame Animation | Frame animation allows you to create fast slide shows. In this animation, you can create a number of slides or frames by making small changes in each frame. As the slide sets are displayed in quick succession, therefore, the changes appears as motion. |
A high-quality animation contains the combination of both the animations, that is, the sprite animation and the frame animation.
Timing Functions to Create Animations
JavaScript provides timing functions to create animation which are listed here in the following table.
Function | Description |
---|---|
setTimeout(function, duration) | This function is used to execute the code sometime in the future |
setInterval(function, duration) | This function is used to execute the code after specified intervals of time |
clearTimeout(setTimeout_variable) | This function is used to clear the timer set by the setTimeout() function |
JavaScript Animations Example
Here is a simple animation example using JavaScript. This is manual animation example in JavaScript:
<html> <head> <title>JavaScript Manual Animation</title> <script type="text/javascript"> var image_object = null; function init() { image_object = document.getElementById('color1'); image_object.style.position= 'relative'; image_object.style.left = '0px'; } function moveRight() { image_object.style.left = parseInt(image_object.style.left) + 10 + 'px'; } window.onload =init; </script> </head> <body> <form> <img id="color1" src="color1.jpg" style="height:100px;weight:100px;"/> <p>Click on the <b>Animate</b> button to animate the image manually.</p> <input type="button" value="Animate" onclick="moveRight();" /> </form> </body> </html>
Here is the sample output produced by the above manual animation example code in JavaScript:
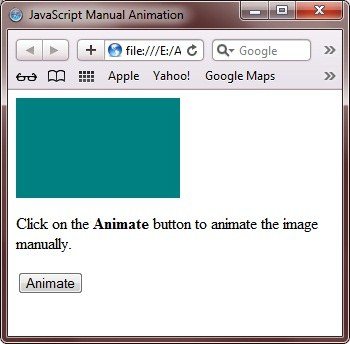
To animate the image, just click on the Animate button. After clicking on the Animate button, one time, the output will become as shown in the figure given below:
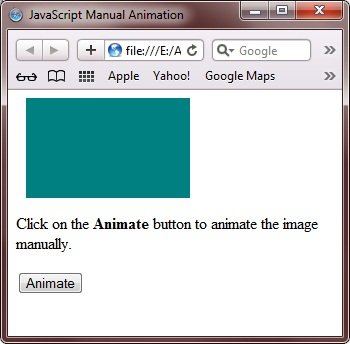
Now again click on the Animate button, the output will looks like:
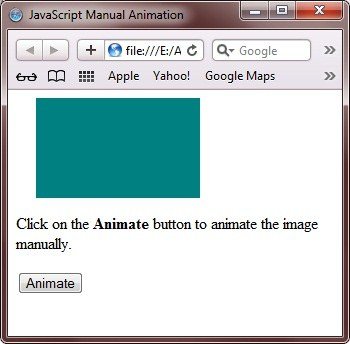
Here is another example on animation using JavaScript:
<html> <head> <title>JavaScript Automatic Animation</title> <script type="text/javascript"> var image_object = null; var animate ; function initializn() { image_object = document.getElementById('image_1'); image_object.style.position= 'relative'; image_object.style.left = '0px'; } function moveRight() { image_object.style.left = parseInt(image_object.style.left) + 10 + 'px'; animate = setTimeout(moveRight,20); // call moveRight in 20msec } function stop() { clearTimeout(animate); image_object.style.left = '0px'; } window.onload = initializn; </script> </head> <body> <form> <img id="image_1" src="img1.jpg" style="height:100px;weight:100px;"/> <p>Click the <b>Start/Start Animation</b> to start and stop the animation effect.</p> <input type="button" value="Start Animation" onclick="moveRight();" /> <input type="button" value="Stop Animation" onclick="stop();" /> </form> </body> </html>
Here is the sample output produced by the above JavaScript animation (automatic animation) example code:
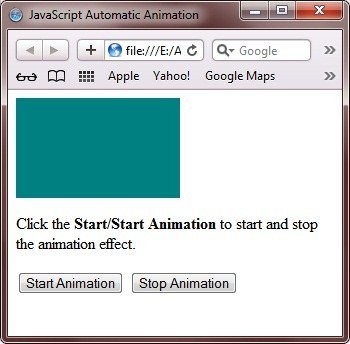
To start the animation effect, click on the Start Animation button, and to stop the animation effect, click on the Stop Animation button. Here is the live demo output of the above Animation example using JavaScript:
« Previous Tutorial Next Tutorial »