- JavaScript Basics
- JS Home
- JS Syntax
- JS Placements
- JS Output
- JS Statements
- JS Keywords
- JS Comments
- JS Variables
- JS var
- JS let
- JS const
- JS var Vs let Vs const
- JS Operators
- JS Arithmetic Operators
- JS Assignment Operators
- JS Comparison Operators
- JS Logical Operators
- JS Bitwise Operators
- JS Ternary Operator
- JS Operator Precedence
- JS Data Types
- JS typeof
- JS Conditional Statements
- JS Conditional Statement
- JS if Statement
- JS if...else Statement
- JS switch Statement
- JS Loops
- JS for Loop
- JS while Loop
- JS do...while Loop
- JS break Statement
- JS continue Statement
- JS break Vs. continue
- JavaScript Popup Boxes
- JS Dialog Box
- JS alert Box
- JS confirm Box
- JS prompt Box
- JavaScript Functions
- JS Functions
- JS setTimeout() Method
- JS setInterval() Method
- JavaScript Events
- JS Events
- JS onclick Event
- JS onload Event
- JS Mouse Events
- JS onreset Event
- JS onsubmit Event
- JavaScript Arrays
- JS Array
- JS Find Length of Array
- JS Add Elements at Beginning
- JS Add Element at End
- JS Remove First Element
- JS Remove Last Element
- JS Get First Index
- JS Get Last Index
- JS Reverse an Array
- JS Sort an Array
- JS Concatenate Arrays
- JS join()
- JS toString()
- JS from()
- JS Check if Value Exists
- JS Check if Array
- JS Slice an Array
- JS splice()
- JS find()
- JS findIndex()
- JS entries()
- JS every()
- JS fill()
- JS filter()
- JS forEach()
- JS map()
- JavaScript Strings
- JS String
- JS Length of String
- JS Convert to Lowercase
- JS Convert to Uppercase
- JS String Concatenation
- JS search()
- JS indexOf()
- JS search() Vs. indexOf()
- JS match()
- JS match() Vs. search()
- JS replace()
- JS toString()
- JS String()
- JS includes()
- JS substr()
- JS Slice String
- JS charAt()
- JS repeat()
- JS split()
- JS charCodeAt()
- JS fromCharCode()
- JS startsWith()
- JS endsWith()
- JS trim()
- JS lastIndexOf()
- JavaScript Objects
- JS Objects
- JS Boolean Object
- JavaScript Math/Number
- JS Math Object
- JS Math.abs()
- JS Math.max()
- JS Math.min()
- JS Math.pow()
- JS Math.sqrt()
- JS Math.cbrt()
- JS Math.round()
- JS Math.ceil()
- JS Math.floor()
- JS Math.trunc
- JS toFixed()
- JS toPrecision()
- JS Math.random()
- JS Math.sign()
- JS Number.isInteger()
- JS NaN
- JS Number()
- JS parseInt()
- JS parseFloat()
- JavaScript Date and Time
- JS Date and Time
- JS Date()
- JS getFullYear()
- JS getMonth()
- JS getDate()
- JS getDay()
- JS getHours()
- JS getMinutes()
- JS getSeconds()
- JS getMilliseconds()
- JS getTime()
- JS getUTCFullYear()
- JS getUTCMonth()
- JS getUTCDate()
- JS getUTCDay()
- JS getUTCHours()
- JS getUTCMinutes()
- JS getUTCSeconds()
- JS getUTCMilliseconds()
- JS toDateString()
- JS toLocaleDateString()
- JS toLocaleTimeString()
- JS toLocaleString()
- JS toUTCString()
- JS getTimezoneOffset()
- JS toISOString()
- JavaScript Browser Objects
- JS Browser Objects
- JS Window Object
- JS Navigator Object
- JS History Object
- JS Screen Object
- JS Location Object
- JavaScript Document Object
- JS Document Object Collection
- JS Document Object Properties
- JS Document Object Methods
- JS Document Object with Forms
- JavaScript DOM
- JS DOM
- JS DOM Nodes
- JS DOM Levels
- JS DOM Interfaces
- JavaScript Cookies
- JS Cookies
- JS Create/Delete Cookies
- JavaScript Regular Expression
- JS Regular Expression
- JS RegEx .
- JS RegEx \w and \W
- JS RegEx \d and \D
- JS RegEx \s and \S
- JS RegEx \b and \B
- JS RegEx \0
- JS RegEx \n
- JS RegEx \xxx
- JS RegEx \xdd
- JS RegEx Quantifiers
- JS RegEx test()
- JS RegEx lastIndex
- JS RegEx source
- JavaScript Advance
- JS Page Redirection
- JS Form Validation
- JS Validations
- JS Error Handling
- JS Exception Handling
- JS try-catch throw finally
- JS onerror Event
- JS Multimedia
- JS Animation
- JS Image Map
- JS Debugging
- JS Browser Detection
- JS Security
- JavaScript Misc
- JS innerHTML
- JS getElementById()
- JS getElementsByClassName()
- JS getElementsByName()
- JS getElementsByTagName()
- JS querySelector()
- JS querySelectorAll()
- JS document.write()
- JS console.log()
- JS instanceof
- JavaScript Programs
- JavaScript Programs
JavaScript Page Redirection
Page redirection helps to send user to page B instead of page A. In other words, page redirection in JavaScript means that when a user clicks on a URL to go to the page A, but internally you are directed the user to another page B.
JavaScript Page Redirection Example
Here is an example of page redirection using JavaScript.
<html> <head> <title>JavaScript Page Redirection</title> <script type="text/javascript"> function pageRedirect() { var borwser_name = navigator.appName; if(borwser_name == "Google Chrome") { window.location="http://fresherearth.com/java"; } else if(borwser_name == "Netscape") { window.location="http://fresherearth.com/c"; } else if(borwser_name =="Microsoft Internet Explorer") { window.location="http://fresherearth.com/cpp"; } else { window.location="http://fresherearth.com"; } } </script> </head> <body> <input type="submit" value="Click to Activate Page Redirection" onclick="pageRedirect()"> </body> </html>
Here are some sample outputs produced by the above page redirection example program using JavaScript. This is the initial output:
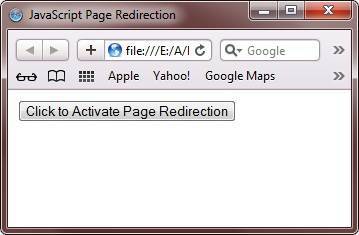
Now click on the Click to Activate Page Redirection button. Here is the output produced in Safari browser:
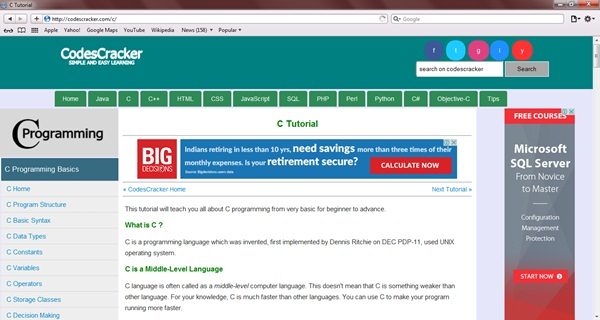
You can also use setTimeout() function to redirect to another page in given time. To use setTimeout() function in page redirection using JavaScript, here is the general form:
setTimeout('Redirect()', 5000);
The function Redirect() in setTimeout(), triggers after 5 seconds.
« Previous Tutorial Next Tutorial »