- JavaScript Basics
- JS Home
- JS Syntax
- JS Placements
- JS Output
- JS Statements
- JS Keywords
- JS Comments
- JS Variables
- JS var
- JS let
- JS const
- JS var Vs let Vs const
- JS Operators
- JS Arithmetic Operators
- JS Assignment Operators
- JS Comparison Operators
- JS Logical Operators
- JS Bitwise Operators
- JS Ternary Operator
- JS Operator Precedence
- JS Data Types
- JS typeof
- JS Conditional Statements
- JS Conditional Statement
- JS if Statement
- JS if...else Statement
- JS switch Statement
- JS Loops
- JS for Loop
- JS while Loop
- JS do...while Loop
- JS break Statement
- JS continue Statement
- JS break Vs. continue
- JavaScript Popup Boxes
- JS Dialog Box
- JS alert Box
- JS confirm Box
- JS prompt Box
- JavaScript Functions
- JS Functions
- JS setTimeout() Method
- JS setInterval() Method
- JavaScript Events
- JS Events
- JS onclick Event
- JS onload Event
- JS Mouse Events
- JS onreset Event
- JS onsubmit Event
- JavaScript Arrays
- JS Array
- JS Find Length of Array
- JS Add Elements at Beginning
- JS Add Element at End
- JS Remove First Element
- JS Remove Last Element
- JS Get First Index
- JS Get Last Index
- JS Reverse an Array
- JS Sort an Array
- JS Concatenate Arrays
- JS join()
- JS toString()
- JS from()
- JS Check if Value Exists
- JS Check if Array
- JS Slice an Array
- JS splice()
- JS find()
- JS findIndex()
- JS entries()
- JS every()
- JS fill()
- JS filter()
- JS forEach()
- JS map()
- JavaScript Strings
- JS String
- JS Length of String
- JS Convert to Lowercase
- JS Convert to Uppercase
- JS String Concatenation
- JS search()
- JS indexOf()
- JS search() Vs. indexOf()
- JS match()
- JS match() Vs. search()
- JS replace()
- JS toString()
- JS String()
- JS includes()
- JS substr()
- JS Slice String
- JS charAt()
- JS repeat()
- JS split()
- JS charCodeAt()
- JS fromCharCode()
- JS startsWith()
- JS endsWith()
- JS trim()
- JS lastIndexOf()
- JavaScript Objects
- JS Objects
- JS Boolean Object
- JavaScript Math/Number
- JS Math Object
- JS Math.abs()
- JS Math.max()
- JS Math.min()
- JS Math.pow()
- JS Math.sqrt()
- JS Math.cbrt()
- JS Math.round()
- JS Math.ceil()
- JS Math.floor()
- JS Math.trunc
- JS toFixed()
- JS toPrecision()
- JS Math.random()
- JS Math.sign()
- JS Number.isInteger()
- JS NaN
- JS Number()
- JS parseInt()
- JS parseFloat()
- JavaScript Date and Time
- JS Date and Time
- JS Date()
- JS getFullYear()
- JS getMonth()
- JS getDate()
- JS getDay()
- JS getHours()
- JS getMinutes()
- JS getSeconds()
- JS getMilliseconds()
- JS getTime()
- JS getUTCFullYear()
- JS getUTCMonth()
- JS getUTCDate()
- JS getUTCDay()
- JS getUTCHours()
- JS getUTCMinutes()
- JS getUTCSeconds()
- JS getUTCMilliseconds()
- JS toDateString()
- JS toLocaleDateString()
- JS toLocaleTimeString()
- JS toLocaleString()
- JS toUTCString()
- JS getTimezoneOffset()
- JS toISOString()
- JavaScript Browser Objects
- JS Browser Objects
- JS Window Object
- JS Navigator Object
- JS History Object
- JS Screen Object
- JS Location Object
- JavaScript Document Object
- JS Document Object Collection
- JS Document Object Properties
- JS Document Object Methods
- JS Document Object with Forms
- JavaScript DOM
- JS DOM
- JS DOM Nodes
- JS DOM Levels
- JS DOM Interfaces
- JavaScript Cookies
- JS Cookies
- JS Create/Delete Cookies
- JavaScript Regular Expression
- JS Regular Expression
- JS RegEx .
- JS RegEx \w and \W
- JS RegEx \d and \D
- JS RegEx \s and \S
- JS RegEx \b and \B
- JS RegEx \0
- JS RegEx \n
- JS RegEx \xxx
- JS RegEx \xdd
- JS RegEx Quantifiers
- JS RegEx test()
- JS RegEx lastIndex
- JS RegEx source
- JavaScript Advance
- JS Page Redirection
- JS Form Validation
- JS Validations
- JS Error Handling
- JS Exception Handling
- JS try-catch throw finally
- JS onerror Event
- JS Multimedia
- JS Animation
- JS Image Map
- JS Debugging
- JS Browser Detection
- JS Security
- JavaScript Misc
- JS innerHTML
- JS getElementById()
- JS getElementsByClassName()
- JS getElementsByName()
- JS getElementsByTagName()
- JS querySelector()
- JS querySelectorAll()
- JS document.write()
- JS console.log()
- JS instanceof
- JavaScript Programs
- JavaScript Programs
JavaScript Boolean
The JavaScript Boolean object is a wrapper class and a member of global objects. It is used to convert the non-boolean values into boolean values.
Boolean object has following two values:
- true
- false
JavaScript Boolean Object Properties
The table given below describes properties of the Boolean object in JavaScript.
Property | Description |
---|---|
constructor | returns the function which has created the prototype of the Boolean object |
prototype | allows you to add properties and methods to an object |
JavaScript Boolean Object Methods
Here is the table describes the methods of the Boolean object in JavaScript.
Method | Description |
---|---|
toString() | converts the boolean value into string and returns the string |
valueOf() | returns the primitive value of a Boolean object |
Here is the general form to create a boolean object in JavaScript:
var val = new Boolean(value);
JavaScript Boolean() Function
The JavaScript Boolean() function is used to find out if an expression or variable is true or not. Here is an example:
Boolean(20 > 9) // returns true
The above expression will return true.
JavaScript Boolean Object Example
Here is an example uses boolean object in JavaScript:
<!DOCTYPE html> <html> <head> <title>JavaScript Boolean Object Example</title> <script> function boolean_fun1() { document.getElementById("boolean_para1").innerHTML = Boolean(10 > 9); } </script> </head> <body> <p>To get the answer, just click on the <b>Answer</b> button given below.</p> <p id="boolean_para1">Is 200 > 9 ?</p> <button onclick="boolean_fun1()">Answer</button> </body> </html>
Here is the output produced by the above JavaScript Boolean object example program. This is the initial output:
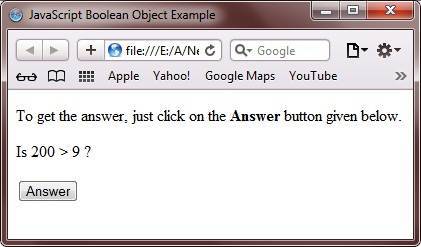
Now to find the answer, that is whether 200 is greater than 9 or not, just click on the Answer button. After clicking on the Answer button you will watch the following output:
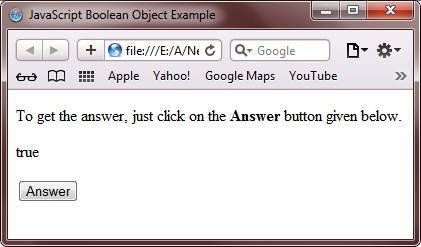
Here is the live demo output produced by the above Boolean object example program in JavaScript.
To get the answer, just click on the Answer button given below.
Is 200 > 9 ?
Here is another example of Boolean Object in JavaScript.
<!DOCTYPE HTML> <HEAD> <TITLE>JavaScript Boolean Object Example</TITLE> <SCRIPT type="text/javascript"> var boolean_object1=new Boolean(0); var boolean_object2=new Boolean(8); var boolean_object3=new Boolean(1); var boolean_object4=new Boolean(""); var boolean_object5=new Boolean("fresherearth"); var boolean_object6=new Boolean('False'); var boolean_object7=new Boolean(null); var boolean_object8=new Boolean(NaN); document.write("The value 0 is boolean "+boolean_object1+"<br>"); document.write("The value 8 is boolean "+boolean_object2+"<br>"); document.write("The value 1 is boolean "+boolean_object3+"<br>"); document.write("An empty string is boolean "+boolean_object4+"<br>"); document.write("The String \"fresherearth\" is boolean "+boolean_object5+"<br>"); document.write("The String 'False' is boolean "+boolean_object6+"<br>"); document.write("null is boolean "+boolean_object7+"<br>"); document.write("NaN is boolean "+boolean_object8); </SCRIPT> </HEAD> <BODY> </BODY> </HTML>
Here is the output produced by the above JavaScript Boolean Object example.
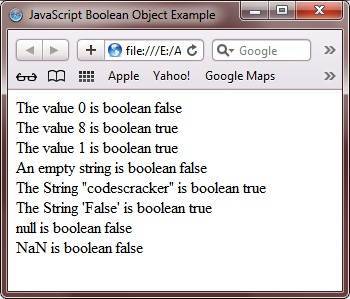
« Previous Tutorial Next Tutorial »