- C Programming
- C Tutorial
- C Basic Syntax
- C Data Types
- C if...else Statement
- C switch Statement
- C for Loop
- C while Loop
- C do...while Loop
- C Jump Statements
- C Arrays
- C Strings
- C Pointers
- C Functions
- C Library Functions
- C Recursion
- C Variable Scope
- C Structures
- C Linked List
- C Stacks
- C Queues
- C Binary Tree
- C Header Files
- C File I/O
- C Programming Examples
- C Programming Examples
Pointers in C programming with example
Pointers are very important in the C language. The correct understanding and use of pointers are important to successful C programming. There are the following reasons behind this:
- Pointers provide the means by which functions can modify their calling arguments.
- Pointers support dynamic allocation.
- Pointers can improve the efficiency of certain routines.
- Pointers support dynamic data structures like binary trees and linked lists.
What are pointers?
A variable that stores a memory address is referred to as a pointer. In addition, this address identifies the location in memory of another object, which is most commonly another variable. For example, if one variable stores the address of another variable, then the first variable is said to point to the second variable. This is because the first variable now contains the address of the second variable. The following is a diagram that illustrates the situation that has arisen:
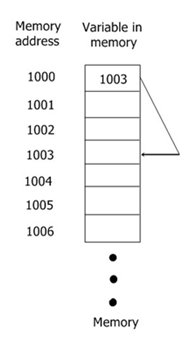
Pointer Variables in C
In order for a variable to function as a pointer, the variable must be declared in the following manner:
type *name;
As can be seen, a pointer declaration starts with the name of the base type, is followed by an asterisk (*), and then the variable's actual name. The pointer's type is determined by its base type, which can be any valid type.
The type of object that the pointer will point to can be determined based on the base type of the pointer.
Pointer Operators in C
There are two types of pointer operators:
- *
- &
The memory address of the operand is returned by the unary "&" operator when it is used. Always remember that a unary operator only requires one operand. Here's an example.
a = &count;
The above statement states that the memory address of the variable "count" is initialized to the variable "a."
And the "*" operator is used when we need to get the value located at the address. This operator is also called the "value at" or "value at address" operator. Consider the following code fragment as an example:
b = *a;
The above statement states that the value at "a" will be initialized to "b." Now let me create a proper example for your understanding.
#include <stdio.h> int main() { int count, *a, b; count = 10; a = &count; b = *a; printf("%d", b); return 0; }
This program produces the following output:
10
That is, first, 10 is initialized to "count," then the address of "count" is initialized to "a." Now using the following statement:
b = *a;
The value at "a" is initialized to "b." Since "a" holds the address of "count," therefore, whatever "count" stores, that value will be initialized to "b."
Don't forget to declare those variables as pointer variables that hold the address.
Pointers example in C
Let me create an example program demonstrating the pointer in the C programming language:
#include <stdio.h> int main() { int a = 99; int *ptr1, *ptr2; ptr1 = &a; ptr2 = ptr1; printf("Value of ptr1: %d\n", *ptr1); printf("Value of ptr2: %d\n\n", *ptr2); printf("Address pointed to by ptr1: %p\n", ptr1); printf("Address pointed to by ptr2: %p\n\n", ptr2); return 0; }
The following snapshot shows the output produced by the above program when I run it in the "Code::Blocks" IDE in my system:
The "%p" format specifier is used to output the address on the output console.
Assuming that the pointer variable "myptr" is of the "int" type and the size of "int" in my system is "4", and if "myptr" initially points to an address of "1000", then "myptr++" states that the pointer moves to the next address, that is, it now holds "1004." For example:
#include <stdio.h> int main() { int num = 10, *myptr; myptr = # printf("The address of 'myptr' = %p \n", myptr); myptr++; printf("Now the current address of 'myptr' = %p \n", myptr); myptr++; printf("Now the current address of 'myptr' = %p \n", myptr); return 0; }
The following snapshot shows the sample run of the above program:
We can also use pointers to work with arrays. Consider the following code fragment:
int arr[10], *ptr; ptr = arr;
Now the pointer variable "ptr" holds the address of the very first element of the array "arr," which is "arr[0]." Because array indexing always starts at zero. Now let's move on to the following statement:
*(ptr+4);
It points to the value or element available at the fourth index. In other words, the above statement refers to the fifth element. Consider the following program as an example illustrating this concept:
#include <stdio.h> int main() { int arr[] = {11, 22, 33, 44, 55, 66, 77, 88, 99, 110}; int *ptr; ptr = arr; printf("The first element = %d \n", *ptr); ptr++; printf("The second element = %d \n", *ptr); ptr++; printf("The third element = %d \n", *ptr); return 0; }
The output is:
The first element = 11 The second element = 22 The third element = 33
However, the same program can also be written in this way:
#include <stdio.h> int main() { int arr[] = {11, 22, 33, 44, 55, 66, 77, 88, 99, 110}; int *ptr; ptr = arr; printf("The first element = %d \n", *ptr); printf("The second element = %d \n", *(ptr+1)); printf("The third element = %d \n", *(ptr+2)); return 0; }
« Previous Tutorial Next Tutorial »