- PHP Basics
- Learn PHP
- PHP Comments
- PHP Data Types
- PHP Variables
- PHP Operators
- PHP echo
- PHP print
- PHP echo vs. print
- PHP if else
- PHP switch
- PHP for Loop
- PHP while Loop
- PHP do...while Loop
- PHP foreach Loop
- PHP break and continue
- PHP Arrays
- PHP print_r()
- PHP unset()
- PHP Strings
- PHP Functions
- PHP File Handling
- PHP File Handling
- PHP Open File
- PHP Create a File
- PHP Write to File
- PHP Read File
- PHP feof()
- PHP fgetc()
- PHP fgets()
- PHP Close File
- PHP Delete File
- PHP Append to File
- PHP Copy File
- PHP file_get_contents()
- PHP file_put_contents()
- PHP file_exists()
- PHP filesize()
- PHP Rename File
- PHP fseek()
- PHP ftell()
- PHP rewind()
- PHP disk_free_space()
- PHP disk_total_space()
- PHP Create Directory
- PHP Remove Directory
- PHP Get Files/Directories
- PHP Get filename
- PHP Get Path
- PHP filemtime()
- PHP file()
- PHP include()
- PHP require()
- PHP include() vs. require()
- PHP and MySQLi
- PHP and MySQLi
- PHP MySQLi Setup
- PHP MySQLi Create DB
- PHP MySQLi Create Table
- PHP MySQLi Connect to DB
- PHP MySQLi Insert Record
- PHP MySQLi Update Record
- PHP MySQLi Fetch Record
- PHP MySQLi Delete Record
- PHP MySQLi SignUp Page
- PHP MySQLi LogIn Page
- PHP MySQLi Store User Data
- PHP MySQLi Close Connection
- PHP Misc Topics
- PHP Object Oriented
- PHP new Keyword
- PHP Cookies
- PHP Sessions
- PHP Date and Time
- PHP GET vs. POST
- PHP File Upload
- PHP Image Processing
PHP Reference
Reference in PHP allows two different PHP variables to write to the same value, for example
$reference_var1 = &$reference_var2;
Here both the variable, that is, $reference_var1 and $reference_var2 are equal.
You can say, both variable point to the same place.
PHP Reference Example
Let's take an example that demonstrates reference in PHP.
<html> <head> <title>PHP Reference Example</title> </head> <body> <?php $num1 = 10; $num2 = &$num1; $sum = $num1 + $num2; echo $sum; ?> </body> </html>
Below is the sample output produced by the above reference example code in PHP.
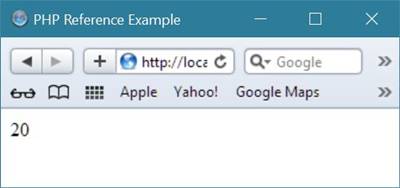
Main Use of Reference in PHP
If you assign the reference of any variable say $first_ref_var to a another variable say $second_ref_var, then if you change the value of variable $first_ref_var, the $second_ref_var's value will also be changed automatically, as you have assigned the reference of that variable.
Let's take another example to understand about the main use of reference in PHP.
<html> <head> <title>Reference example in PHP</title> </head> <body> <?php echo "With Reference:<br/>"; // initialize first variable with value $num1 = 10; // initialize the reference of first variable to second variable $num2 = &$num1; $sum = $num1 + $num2; echo $sum; echo "<br/>"; // change the value of first variable $num1 = 20; echo $num1; echo "<br/>"; // you will see, the value of second variable will also be changed // this is the benefit of using reference in PHP echo $num2; echo "<hr/>"; echo "Without Reference:<br/>"; $var1 = 10; $var2 = $var1; $add = $var1 + $var2; echo $add; $var1 = 20; echo "<br/>"; echo $var1; echo "<br/>"; echo $var2; ?> </body> </html>
Here is the sample output of the above reference example in PHP.
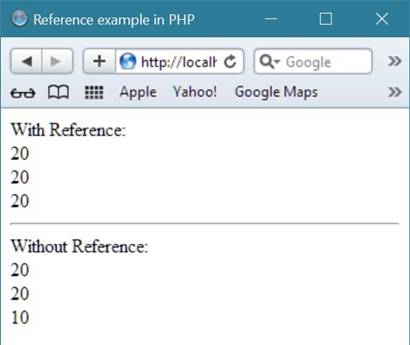
« Previous Tutorial Next Tutorial »